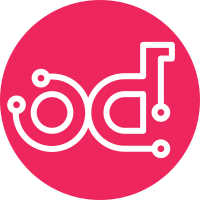
* command internal logic was revised and optimized * added ability to delete/reset context fields * unit tests were reorganized, new cases were added Change-Id: Ie35d11405e88fea21abf33cb75f44b03bb4644fd Signed-off-by: Ruslan Aliev <raliev@mirantis.com> Relates-To: #348
112 lines
2.9 KiB
Go
112 lines
2.9 KiB
Go
/*
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
package config
|
|
|
|
import (
|
|
"github.com/spf13/cobra"
|
|
"github.com/spf13/pflag"
|
|
|
|
"opendev.org/airship/airshipctl/pkg/config"
|
|
)
|
|
|
|
const (
|
|
setContextLong = `
|
|
Create or modify a context in the airshipctl config files.
|
|
`
|
|
|
|
setContextExample = `
|
|
# Create a new context named "exampleContext"
|
|
airshipctl config set-context exampleContext \
|
|
--manifest=exampleManifest \
|
|
|
|
# Update the manifest of the current-context
|
|
airshipctl config set-context \
|
|
--current \
|
|
--manifest=exampleManifest
|
|
`
|
|
|
|
setContextManifestFlag = "manifest"
|
|
setContextManagementConfigFlag = "management-config"
|
|
setContextCurrentFlag = "current"
|
|
)
|
|
|
|
// NewSetContextCommand creates a command for creating and modifying contexts
|
|
// in the airshipctl config
|
|
func NewSetContextCommand(cfgFactory config.Factory) *cobra.Command {
|
|
o := &config.ContextOptions{}
|
|
cmd := &cobra.Command{
|
|
Use: "set-context NAME",
|
|
Short: "Manage contexts",
|
|
Long: setContextLong[1:],
|
|
Example: setContextExample,
|
|
Args: cobra.MaximumNArgs(1),
|
|
RunE: setContextRunE(cfgFactory, o),
|
|
}
|
|
|
|
addSetContextFlags(cmd, o)
|
|
return cmd
|
|
}
|
|
|
|
func addSetContextFlags(cmd *cobra.Command, o *config.ContextOptions) {
|
|
flags := cmd.Flags()
|
|
|
|
flags.StringVar(
|
|
&o.Manifest,
|
|
setContextManifestFlag,
|
|
"",
|
|
"set the manifest for the specified context")
|
|
|
|
flags.StringVar(
|
|
&o.ManagementConfiguration,
|
|
setContextManagementConfigFlag,
|
|
"",
|
|
"set the management config for the specified context")
|
|
|
|
flags.BoolVar(
|
|
&o.Current,
|
|
setContextCurrentFlag,
|
|
false,
|
|
"update the current context")
|
|
}
|
|
|
|
func setContextRunE(cfgFactory config.Factory, o *config.ContextOptions) func(cmd *cobra.Command, args []string) error {
|
|
return func(cmd *cobra.Command, args []string) error {
|
|
ctxName := ""
|
|
if len(args) == 1 {
|
|
ctxName = args[0]
|
|
}
|
|
|
|
// Go through all the flags that have been set
|
|
var opts []config.ContextOption
|
|
fn := func(flag *pflag.Flag) {
|
|
switch flag.Name {
|
|
case setContextManifestFlag:
|
|
opts = append(opts, config.SetContextManifest(o.Manifest))
|
|
case setContextManagementConfigFlag:
|
|
opts = append(opts, config.SetContextManagementConfig(o.ManagementConfiguration))
|
|
}
|
|
}
|
|
cmd.Flags().Visit(fn)
|
|
|
|
options := &config.RunSetContextOptions{
|
|
CfgFactory: cfgFactory,
|
|
CtxName: ctxName,
|
|
Current: o.Current,
|
|
Writer: cmd.OutOrStdout(),
|
|
}
|
|
return options.RunSetContext(opts...)
|
|
}
|
|
}
|