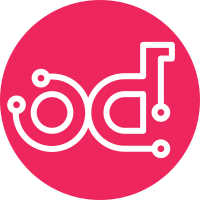
Also removes pkg dependencies that were introduced by the BMH logic. Change-Id: Ib1235b5a8043f2f53d90a818fff25df4fdb9ec20
55 lines
1.7 KiB
Go
55 lines
1.7 KiB
Go
/*
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
https://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
package client
|
|
|
|
import (
|
|
"github.com/pkg/errors"
|
|
|
|
clusterctlclient "sigs.k8s.io/cluster-api/cmd/clusterctl/client"
|
|
"sigs.k8s.io/cluster-api/cmd/clusterctl/client/cluster"
|
|
)
|
|
|
|
// Move implements interface to Clusterctl
|
|
func (c *Client) Move(fromKubeconfigPath, fromKubeconfigContext,
|
|
toKubeconfigPath, toKubeconfigContext, namespace string) error {
|
|
var err error
|
|
// ephemeral cluster client
|
|
pFrom := cluster.New(cluster.Kubeconfig{
|
|
Path: fromKubeconfigPath,
|
|
Context: fromKubeconfigContext}, nil).Proxy()
|
|
|
|
// If namespace is empty, try to detect it.
|
|
if namespace == "" {
|
|
var currentNamespace string
|
|
currentNamespace, err = pFrom.CurrentNamespace()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
namespace = currentNamespace
|
|
}
|
|
|
|
// clusterctl move
|
|
c.moveOptions = clusterctlclient.MoveOptions{
|
|
FromKubeconfig: clusterctlclient.Kubeconfig{Path: fromKubeconfigPath, Context: fromKubeconfigContext},
|
|
ToKubeconfig: clusterctlclient.Kubeconfig{Path: toKubeconfigPath, Context: toKubeconfigContext},
|
|
Namespace: namespace,
|
|
}
|
|
err = c.clusterctlClient.Move(c.moveOptions)
|
|
if err != nil {
|
|
return errors.Wrapf(err, "error during clusterctl move")
|
|
}
|
|
return nil
|
|
}
|