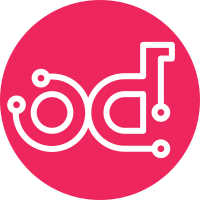
This commit removes any assertion from Go's "testing" package, preferring instead to use an assertion from the testify package. All tests now have uniformity. This also decrease the number of iterations in the password generation test, decreasing test runtime tenfold Change-Id: I8799110e93dfa19bebe9050528e865b4c991c3df
58 lines
1.3 KiB
Go
58 lines
1.3 KiB
Go
package cmd_test
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/spf13/cobra"
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"opendev.org/airship/airshipctl/cmd"
|
|
"opendev.org/airship/airshipctl/cmd/bootstrap"
|
|
"opendev.org/airship/airshipctl/pkg/environment"
|
|
"opendev.org/airship/airshipctl/testutil"
|
|
)
|
|
|
|
func TestRoot(t *testing.T) {
|
|
tests := []*testutil.CmdTest{
|
|
{
|
|
Name: "rootCmd-with-no-defaults",
|
|
CmdLine: "",
|
|
Cmd: getVanillaRootCmd(t),
|
|
},
|
|
{
|
|
Name: "rootCmd-with-defaults",
|
|
CmdLine: "",
|
|
Cmd: getDefaultRootCmd(t),
|
|
},
|
|
{
|
|
Name: "specialized-rootCmd-with-bootstrap",
|
|
CmdLine: "",
|
|
Cmd: getSpecializedRootCmd(t),
|
|
},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
testutil.RunTest(t, tt)
|
|
}
|
|
}
|
|
|
|
func getVanillaRootCmd(t *testing.T) *cobra.Command {
|
|
t.Helper()
|
|
rootCmd, _, err := cmd.NewRootCmd(nil)
|
|
require.NoError(t, err, "Could not create root commands")
|
|
return rootCmd
|
|
}
|
|
|
|
func getDefaultRootCmd(t *testing.T) *cobra.Command {
|
|
t.Helper()
|
|
rootCmd, _, err := cmd.NewAirshipCTLCommand(nil)
|
|
require.NoError(t, err, "Could not create root commands")
|
|
return rootCmd
|
|
}
|
|
|
|
func getSpecializedRootCmd(t *testing.T) *cobra.Command {
|
|
rootCmd := getVanillaRootCmd(t)
|
|
rootCmd.AddCommand(bootstrap.NewBootstrapCommand(&environment.AirshipCTLSettings{}))
|
|
return rootCmd
|
|
}
|