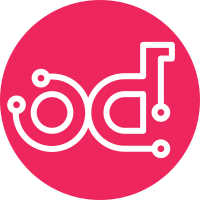
* added license templates for go, bash & yaml in tools dir * added a script that will add license information for all missing files. Type: go, yaml, yml, sh * skip adding license for all files within testdata * Syntax: > ./tools/add_license.sh * Skip license for manifests folder * Added one extra line after licene for yaml files * Added License after Hashbang for bash. * Add an extra line after hashbang and before license * Updated the go template to use multiline comments New Files: 1. tools/add_license.sh 2. tools/license_go.txt 3. tools/license_yaml.txt 4. tools/license_bash.txt Change-Id: Ia4da5b261e7cd518d446896b72c810421877472a Realtes-To:#147
116 lines
3.0 KiB
Go
116 lines
3.0 KiB
Go
/*
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
https://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
package secret_test
|
|
|
|
import (
|
|
"math/rand"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
|
|
"opendev.org/airship/airshipctl/pkg/secret"
|
|
)
|
|
|
|
const (
|
|
asciiLowers = "abcdefghijklmnopqrstuvwxyz"
|
|
asciiUppers = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
|
|
asciiNumbers = "0123456789"
|
|
asciiSymbols = "@#&-+=?"
|
|
|
|
defaultLength = 24
|
|
)
|
|
|
|
func TestDeterministicGenerateValidPassphrase(t *testing.T) {
|
|
assert := assert.New(t)
|
|
testSource := rand.NewSource(42)
|
|
engine := secret.NewPassphraseEngine(testSource)
|
|
|
|
// pre-calculated for rand.NewSource(42)
|
|
expectedPassphrases := []string{
|
|
"erx&97vfqd7LN3HJ?t@oPhds",
|
|
"##Xeuvf5Njy@hNWSaRoleFkf",
|
|
"jB=kirg7acIt-=fx1Fb-tZ+7",
|
|
"eOS#W8yoAljSThPL2oT&aUZu",
|
|
"vlaQqKr-jXSCJfXYnvGik3b1",
|
|
"rBKtHZkOmFUM75?c2UWiZjdh",
|
|
"9g?QV?w6BCWN2EKAc+dZ-Jun",
|
|
"X@IIyqAg7Mz@Wm8eRE6KMEf3",
|
|
"7JpQkLd3ufhj4bLB8S=ipjNP",
|
|
"XC?bDaHTa3mrBYLMG@#B=Q0B",
|
|
}
|
|
|
|
for i, expected := range expectedPassphrases {
|
|
actual := engine.GeneratePassphrase()
|
|
assert.Equal(expected, actual, "Test #%d failed", i)
|
|
}
|
|
}
|
|
|
|
func TestNondeterministicGenerateValidPassphrase(t *testing.T) {
|
|
assert := assert.New(t)
|
|
// Due to the nondeterminism of random number generators, this
|
|
// functionality is impossible to fully test. Let's just generate
|
|
// enough passphrases that we can be confident in the randomness.
|
|
// Fortunately, Go is pretty fast, so we can do upward of 10,000 of
|
|
// these without slowing down the test too much
|
|
charSets := []string{
|
|
asciiLowers,
|
|
asciiUppers,
|
|
asciiNumbers,
|
|
asciiSymbols,
|
|
}
|
|
|
|
engine := secret.NewPassphraseEngine(nil)
|
|
for i := 0; i < 10000; i++ {
|
|
passphrase := engine.GeneratePassphrase()
|
|
assert.Truef(len(passphrase) >= defaultLength,
|
|
"%s does not meet the length requirement", passphrase)
|
|
|
|
for _, charSet := range charSets {
|
|
assert.Truef(strings.ContainsAny(passphrase, charSet),
|
|
"%s does not contain any characters from [%s]",
|
|
passphrase, charSet)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestGenerateValidPassphraseN(t *testing.T) {
|
|
assert := assert.New(t)
|
|
testSource := rand.NewSource(42)
|
|
engine := secret.NewPassphraseEngine(testSource)
|
|
tests := []struct {
|
|
inputLegth int
|
|
expectedLength int
|
|
}{
|
|
{
|
|
inputLegth: 10,
|
|
expectedLength: defaultLength,
|
|
},
|
|
{
|
|
inputLegth: -5,
|
|
expectedLength: defaultLength,
|
|
},
|
|
{
|
|
inputLegth: 30,
|
|
expectedLength: 30,
|
|
},
|
|
}
|
|
|
|
for _, tt := range tests {
|
|
passphrase := engine.GeneratePassphraseN(tt.inputLegth)
|
|
assert.Len(passphrase, tt.expectedLength)
|
|
}
|
|
}
|