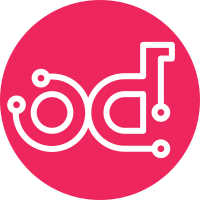
Using this new structure, developers can rebuild, test, and lint individually, saving time an effort in the event of a failure. Added Frontend unit testing alongside a more modular module design to make components and services easier to test and easy to manage dependencies for. Adding Yarn for more performant package management Change-Id: I56a57100b5d831bb31bc18e2c62c78bf63265324
59 lines
2.2 KiB
Bash
Executable File
59 lines
2.2 KiB
Bash
Executable File
#!/bin/bash
|
|
set -x
|
|
|
|
tools_bin_dir="${BASH_SOURCE%/*}"
|
|
node_version=v12.16.3
|
|
|
|
if [[ ! -d $tools_bin_dir/node-$node_version ]]; then
|
|
if [[ "$OSTYPE" == "linux-gnu"* ]]; then
|
|
# Linux
|
|
if ! curl -sfL "https://nodejs.org/dist/$node_version/node-$node_version-linux-x64.tar.gz" | tar zxf - --directory "$tools_bin_dir"; then
|
|
printf "Something went wrong while installing linux-gnu nodejs\n" 1>&2
|
|
exit 1
|
|
else
|
|
mv $tools_bin_dir/node-$node_version-linux-x64 $tools_bin_dir/node-$node_version
|
|
fi
|
|
elif [[ "$OSTYPE" == "darwin"* ]]; then
|
|
# Mac OSX
|
|
if ! curl -sfL "https://nodejs.org/dist/$node_version/node-$node_version-darwin-x64.tar.gz" | tar zxf - --directory "$tools_bin_dir"; then
|
|
printf "Something went wrong while installing Mac OSX nodejs\n" 1>&2
|
|
exit 1
|
|
else
|
|
mv $tools_bin_dir/node-$node_version-darwin-x64 $tools_bin_dir/node-$node_version
|
|
fi
|
|
elif [[ "$OSTYPE" == "cygwin" ]]; then
|
|
# Windows
|
|
if ! wget -qO- https://nodejs.org/dist/$node_version/node-$node_version-win-x64.zip | bsdtar -xf- -C tools; then
|
|
printf "Something went wrong while installing Windows nodejs\n" 1>&2
|
|
exit 1
|
|
else
|
|
mv $tools_bin_dir/node-$node_version-win-x64 $tools_bin_dir/node-$node_version
|
|
# the windows install doesn't conform to the same directory structure so making it conform
|
|
mkdir $tools_bin_dir/node-$node_version/bin
|
|
mv $tools_bin_dir/node-$node_version/n* $tools_bin_dir/node-$node_version/bin
|
|
chmod -R a+x $tools_bin_dir/node-$node_version/bin
|
|
fi
|
|
fi
|
|
|
|
# npm requires node to also be on the path
|
|
export PATH=$(realpath $tools_bin_dir)/node-$node_version/bin:$PATH
|
|
|
|
# Proxy / SSL issues when using custom CAs with proxy / self signed certs.
|
|
# This assumes the system certs are up to date and is on linux or in docker
|
|
if [ -r /etc/ssl/certs/ca-certificates.crt ]; then
|
|
export NODE_EXTRA_CA_CERTS=/etc/ssl/certs/ca-certificates.crt
|
|
fi
|
|
|
|
# angular-cli is required by angular build
|
|
if ! npm i -g @angular/cli; then
|
|
printf "Something went wrong while installing Angular CLI (ng)\n" 1>&2
|
|
exit 1
|
|
fi
|
|
# yarn is required by angular build
|
|
if ! npm i -g yarn; then
|
|
printf "Something went wrong while installing yarn\n" 1>&2
|
|
exit 1
|
|
fi
|
|
cd ..
|
|
fi
|