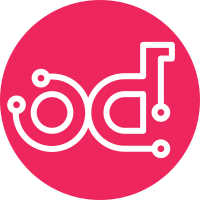
Airship 2 is using Argo for workflow management, rather than the builtin Armada workflow functionality. Hence, this adds an apply_chart CLI command to apply a single chart at a time, so that Argo can manage the higher level orchestration. Airship 2 is also using kubernetes as opposed to Deckhand as the document store. Hence this adds an ArmadaChart kubernetes CRD, which can be consumed by the apply_chart CLI command. The chart `dependencies` feature is intentionally not supported by the CRD, as there are additional complexities to make that work, and ideally this feature should be deprecated as charts should be building in there dependencies before consumption by Armada. Functional tests are included to excercise these features against a minikube cluster. Change-Id: I2bbed83d6d80091322a7e60b918a534188467239
99 lines
2.8 KiB
Python
99 lines
2.8 KiB
Python
# Copyright 2017 The Armada Authors.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
from urllib.parse import urlparse
|
|
|
|
import click
|
|
from oslo_config import cfg
|
|
from oslo_log import log
|
|
|
|
from armada.cli.apply import apply_create
|
|
from armada.cli.apply_chart import apply_chart
|
|
from armada.cli.delete import delete_charts
|
|
from armada.cli.rollback import rollback_charts
|
|
from armada.cli.test import test_charts
|
|
from armada.cli.tiller import tiller_service
|
|
from armada.cli.validate import validate_manifest
|
|
from armada.common.client import ArmadaClient
|
|
from armada.common.session import ArmadaSession
|
|
|
|
CONF = cfg.CONF
|
|
|
|
|
|
@click.group()
|
|
@click.option('--debug', help="Enable debug logging", is_flag=True)
|
|
@click.option(
|
|
'--api/--no-api',
|
|
help="Execute service endpoints. (requires url option)",
|
|
default=False)
|
|
@click.option(
|
|
'--url', help="Armada Service Endpoint", envvar='HOST', default=None)
|
|
@click.option(
|
|
'--token', help="Keystone Service Token", envvar='TOKEN', default=None)
|
|
@click.pass_context
|
|
def main(ctx, debug, api, url, token):
|
|
"""
|
|
Multi Helm Chart Deployment Manager
|
|
|
|
Common actions from this point include:
|
|
|
|
\b
|
|
$ armada apply
|
|
$ armada apply_chart
|
|
$ armada delete
|
|
$ armada rollback
|
|
$ armada test
|
|
$ armada tiller
|
|
$ armada validate
|
|
|
|
Environment:
|
|
|
|
\b
|
|
$TOKEN set auth token
|
|
$HOST set armada service host endpoint
|
|
|
|
This tool will communicate with deployed Tiller in your Kubernetes cluster.
|
|
"""
|
|
|
|
if not ctx.obj:
|
|
ctx.obj = {}
|
|
|
|
if api:
|
|
if not url or not token:
|
|
raise click.ClickException(
|
|
'When api option is enabled user needs to pass url and token')
|
|
else:
|
|
ctx.obj['api'] = api
|
|
parsed_url = urlparse(url)
|
|
ctx.obj['CLIENT'] = ArmadaClient(
|
|
ArmadaSession(
|
|
host=parsed_url.netloc,
|
|
scheme=parsed_url.scheme,
|
|
token=token))
|
|
|
|
if debug:
|
|
CONF.debug = debug
|
|
|
|
log.set_defaults(default_log_levels=CONF.default_log_levels)
|
|
log.setup(CONF, 'armada')
|
|
|
|
|
|
main.add_command(apply_create)
|
|
main.add_command(apply_chart)
|
|
main.add_command(delete_charts)
|
|
main.add_command(rollback_charts)
|
|
main.add_command(test_charts)
|
|
main.add_command(tiller_service)
|
|
main.add_command(validate_manifest)
|