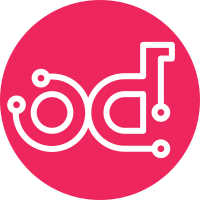
Adds the options implemented in Spyglass for intermediary validation to the plugin CLI Related Change: https://review.opendev.org/#/c/678642/ Depends-On: Iaa385d265b027426f8e5f2376462ffb4c0d1d3fa Change-Id: I15e348916c14927ff9a5e6a27e55a912f8dd3126
120 lines
3.7 KiB
Python
120 lines
3.7 KiB
Python
# Copyright 2019 AT&T Intellectual Property. All other rights reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
import logging
|
|
|
|
import click
|
|
import spyglass
|
|
from spyglass import cli
|
|
from spyglass.site_processors.site_processor import SiteProcessor
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
LOG_FORMAT = '%(asctime)s %(levelname)-8s %(name)s:' \
|
|
'%(funcName)s [%(lineno)3d] %(message)s'
|
|
|
|
EXCEL_FILE_OPTION = click.option(
|
|
'-x',
|
|
'--excel-file',
|
|
'excel_file',
|
|
type=click.Path(exists=True, readable=True, dir_okay=False),
|
|
required=True,
|
|
help='Path to the engineering Excel file. Required for '
|
|
'spyglass-plugin-xls plugin.',
|
|
)
|
|
|
|
EXCEL_SPEC_OPTION = click.option(
|
|
'-e',
|
|
'--excel-spec',
|
|
'excel_spec',
|
|
type=click.Path(exists=True, readable=True, dir_okay=False),
|
|
required=True,
|
|
help='Path to the Excel specification YAML file for the engineering '
|
|
'Excel file. Required for spyglass-plugin-xls plugin.',
|
|
)
|
|
|
|
|
|
@click.option(
|
|
'-v',
|
|
'--verbose',
|
|
is_flag=True,
|
|
default=False,
|
|
help='Enable debug messages in log.')
|
|
@click.group()
|
|
def excel(*, verbose):
|
|
"""Plugin for extracting site data from Excel spreadsheets"""
|
|
if verbose:
|
|
log_level = logging.DEBUG
|
|
else:
|
|
log_level = logging.INFO
|
|
logging.basicConfig(format=LOG_FORMAT, level=log_level)
|
|
|
|
|
|
@excel.command(
|
|
'intermediary',
|
|
short_help='generate intermediary',
|
|
help='Generates an intermediary file from passed excel data.')
|
|
@EXCEL_FILE_OPTION
|
|
@EXCEL_SPEC_OPTION
|
|
@cli.SITE_CONFIGURATION_FILE_OPTION
|
|
@cli.INTERMEDIARY_DIR_OPTION
|
|
@cli.INTERMEDIARY_SCHEMA_OPTION
|
|
@cli.NO_INTERMEDIARY_VALIDATION_OPTION
|
|
@cli.SITE_NAME_CONFIGURATION_OPTION
|
|
def generate_intermediary(*args, **kwargs):
|
|
process_input_ob = \
|
|
spyglass.cli.intermediary_processor('excel', **kwargs)
|
|
LOG.info("Generate intermediary yaml")
|
|
process_input_ob.generate_intermediary_yaml()
|
|
process_input_ob.dump_intermediary_file(kwargs['intermediary_dir'])
|
|
|
|
|
|
@excel.command(
|
|
'documents',
|
|
short_help='generates manifest documents and intermediary',
|
|
help='Generates manifest documents and intermediary file.')
|
|
@click.option(
|
|
'-i',
|
|
'--generate-intermediary',
|
|
'generate_intermediary',
|
|
is_flag=True,
|
|
default=False,
|
|
help='Flag to save the generated intermediary file used for the manifests.'
|
|
)
|
|
@EXCEL_FILE_OPTION
|
|
@EXCEL_SPEC_OPTION
|
|
@cli.SITE_CONFIGURATION_FILE_OPTION
|
|
@cli.INTERMEDIARY_DIR_OPTION
|
|
@cli.INTERMEDIARY_SCHEMA_OPTION
|
|
@cli.NO_INTERMEDIARY_VALIDATION_OPTION
|
|
@cli.SITE_NAME_CONFIGURATION_OPTION
|
|
@cli.TEMPLATE_DIR_OPTION
|
|
@cli.MANIFEST_DIR_OPTION
|
|
@cli.FORCE_OPTION
|
|
def generate_manifests_and_intermediary(*args, **kwargs):
|
|
process_input_ob = \
|
|
spyglass.cli.intermediary_processor('excel', **kwargs)
|
|
LOG.info("Generate intermediary yaml")
|
|
site_data = process_input_ob.generate_intermediary_yaml()
|
|
if kwargs["generate_intermediary"]:
|
|
LOG.debug("Dumping intermediary yaml")
|
|
process_input_ob.dump_intermediary_file(kwargs['intermediary_dir'])
|
|
else:
|
|
LOG.debug("Skipping dump for intermediary yaml")
|
|
|
|
LOG.info("Generating site Manifests")
|
|
processor_engine = SiteProcessor(
|
|
site_data, kwargs['manifest_dir'], kwargs['force'])
|
|
processor_engine.render_template(kwargs['template_dir'])
|