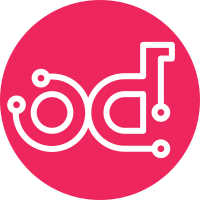
Jenkins Wiki has been fully taken off line, so update the remaining links to reference either the relevant plugin page or the github repo. Add extlink target for repo in jenkinsci github org. The sonatype-clm plugin seems to be more-or-less completely deprecated, so update the description to indicate this and link to the nexus-artifact-uploader plugin. Update the jjb sphinx plugin so that it generates references for the yamlfunctions. Change-Id: If2241e751d01a60a8cb4cbaea5b3176aeb92eab4 Signed-off-by: Pat Long <pllong@arista.com>
74 lines
2.4 KiB
Python
74 lines
2.4 KiB
Python
# -*- coding: utf-8 -*-
|
|
# Copyright (C) 2013 eNovance SAS <licensing@enovance.com>
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
|
|
"""
|
|
The flow Project module handles creating Jenkins flow projects.
|
|
You may specify ``flow`` in the ``project-type`` attribute of
|
|
the :ref:`Job` definition.
|
|
|
|
Requires the Jenkins :jenkins-plugins:`Build Flow Plugin <build-flow-plugin>`.
|
|
|
|
In order to use it for job-template you have to escape the curly braces by
|
|
doubling them in the DSL: { -> {{ , otherwise it will be interpreted by the
|
|
python str.format() command.
|
|
|
|
:Job Parameters:
|
|
* **dsl** (`str`): The DSL content. (optional)
|
|
* **needs-workspace** (`bool`): This build needs a workspace. \
|
|
(default false)
|
|
* **dsl-file** (`str`): Path to the DSL script in the workspace. \
|
|
Has effect only when `needs-workspace` is true. (optional)
|
|
|
|
Job example:
|
|
|
|
.. literalinclude::
|
|
/../../tests/yamlparser/fixtures/project_flow_template001.yaml
|
|
|
|
Job template example:
|
|
|
|
.. literalinclude::
|
|
/../../tests/yamlparser/fixtures/project_flow_template002.yaml
|
|
|
|
Job example runninng a DSL file from the workspace:
|
|
|
|
.. literalinclude::
|
|
/../../tests/yamlparser/fixtures/project_flow_template003.yaml
|
|
|
|
"""
|
|
|
|
import xml.etree.ElementTree as XML
|
|
|
|
import jenkins_jobs.modules.base
|
|
from jenkins_jobs.modules.helpers import convert_mapping_to_xml
|
|
|
|
|
|
class Flow(jenkins_jobs.modules.base.Base):
|
|
sequence = 0
|
|
|
|
def root_xml(self, data):
|
|
xml_parent = XML.Element("com.cloudbees.plugins.flow.BuildFlow")
|
|
|
|
needs_workspace = data.get("needs-workspace", False)
|
|
mapping = [
|
|
("dsl", "dsl", ""),
|
|
("needs-workspace", "buildNeedsWorkspace", False),
|
|
]
|
|
convert_mapping_to_xml(xml_parent, data, mapping, fail_required=True)
|
|
if needs_workspace and "dsl-file" in data:
|
|
XML.SubElement(xml_parent, "dslFile").text = data["dsl-file"]
|
|
|
|
return xml_parent
|