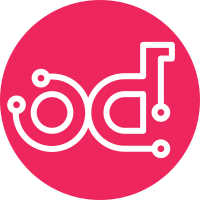
Gerrit remembers the last page that contains a change-list (either a dashboard or a page with search results) so that users can return there with the 'u' key. This is done by saving the last relative URL as a property at the gr-app level. The app relied on the 'location-changed' event to set this property, but in some cases that event is fired before the app params are set. One such case is on a fresh reload of a search page. In that case, the _handleSearchPageChange function has no way of knowing what view is currently loaded, and prematurely exits. With this change, Gerrit now observes the app params to set the property. Bug: Issue 7368 Change-Id: I62ead340677d9a416a4713ff003fc3ef18d3624f
169 lines
5.4 KiB
HTML
169 lines
5.4 KiB
HTML
<!DOCTYPE html>
|
|
<!--
|
|
@license
|
|
Copyright (C) 2016 The Android Open Source Project
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
-->
|
|
|
|
<meta name="viewport" content="width=device-width, minimum-scale=1.0, initial-scale=1.0, user-scalable=yes">
|
|
<title>gr-app</title>
|
|
|
|
<script src="../bower_components/webcomponentsjs/webcomponents-lite.min.js"></script>
|
|
<script src="../bower_components/web-component-tester/browser.js"></script>
|
|
<link rel="import" href="../test/common-test-setup.html"/>
|
|
<link rel="import" href="gr-app.html">
|
|
|
|
<script>void(0);</script>
|
|
|
|
<test-fixture id="basic">
|
|
<template>
|
|
<gr-app id="app"></gr-app>
|
|
</template>
|
|
</test-fixture>
|
|
|
|
<script>
|
|
suite('gr-app tests', () => {
|
|
let sandbox;
|
|
let element;
|
|
|
|
setup(done => {
|
|
sandbox = sinon.sandbox.create();
|
|
stub('gr-reporting', {
|
|
appStarted: sandbox.stub(),
|
|
});
|
|
stub('gr-account-dropdown', {
|
|
_getTopContent: sinon.stub(),
|
|
});
|
|
stub('gr-rest-api-interface', {
|
|
getAccount() { return Promise.resolve({}); },
|
|
getAccountCapabilities() { return Promise.resolve({}); },
|
|
getConfig() {
|
|
return Promise.resolve({
|
|
gerrit: {web_uis: ['GWT', 'POLYGERRIT']},
|
|
plugin: {},
|
|
});
|
|
},
|
|
getPreferences() { return Promise.resolve({my: []}); },
|
|
getDiffPreferences() { return Promise.resolve({}); },
|
|
getEditPreferences() { return Promise.resolve({}); },
|
|
getVersion() { return Promise.resolve(42); },
|
|
probePath() { return Promise.resolve(42); },
|
|
});
|
|
|
|
element = fixture('basic');
|
|
flush(done);
|
|
});
|
|
|
|
teardown(() => {
|
|
sandbox.restore();
|
|
});
|
|
|
|
test('reporting', () => {
|
|
assert.isTrue(element.$.reporting.appStarted.calledOnce);
|
|
});
|
|
|
|
test('location change updates gwt footer', done => {
|
|
element._path = '/test/path';
|
|
flush(() => {
|
|
const gwtLink = element.$$('#gwtLink');
|
|
assert.equal(gwtLink.href, 'http://' + location.host +
|
|
element.getBaseUrl() + '/?polygerrit=0#/test/path');
|
|
done();
|
|
});
|
|
});
|
|
|
|
test('_handleLocationChange handles hashes', done => {
|
|
const curLocation = {
|
|
pathname: '/c/1/1/testfile.txt',
|
|
hash: '#2',
|
|
host: location.host,
|
|
};
|
|
element._handleLocationChange({detail: curLocation});
|
|
|
|
flush(() => {
|
|
const gwtLink = element.$$('#gwtLink');
|
|
assert.equal(
|
|
gwtLink.href,
|
|
'http://' + location.host + element.getBaseUrl() +
|
|
'/?polygerrit=0#/c/1/1/testfile.txt@2'
|
|
);
|
|
done();
|
|
});
|
|
});
|
|
|
|
test('passes config to gr-plugin-host', () => {
|
|
return element.$.restAPI.getConfig.lastCall.returnValue.then(config => {
|
|
assert.deepEqual(element.$.plugins.config, config);
|
|
});
|
|
});
|
|
|
|
test('_paramsChanged sets search page', () => {
|
|
element._paramsChanged({base: {view: Gerrit.Nav.View.CHANGE}});
|
|
assert.notOk(element._lastSearchPage);
|
|
element._paramsChanged({base: {view: Gerrit.Nav.View.SEARCH}});
|
|
assert.ok(element._lastSearchPage);
|
|
});
|
|
|
|
suite('_jumpKeyPressed', () => {
|
|
let navStub;
|
|
|
|
setup(() => {
|
|
navStub = sandbox.stub(Gerrit.Nav, 'navigateToStatusSearch');
|
|
sandbox.stub(Date, 'now').returns(10000);
|
|
});
|
|
|
|
test('success', () => {
|
|
const e = {detail: {key: 'a'}, preventDefault: () => {}};
|
|
sandbox.stub(element, 'shouldSuppressKeyboardShortcut').returns(false);
|
|
element._lastGKeyPressTimestamp = 9000;
|
|
element._jumpKeyPressed(e);
|
|
assert.isTrue(navStub.calledOnce);
|
|
assert.equal(navStub.lastCall.args[0], 'abandoned');
|
|
});
|
|
|
|
test('no g key', () => {
|
|
const e = {detail: {key: 'a'}, preventDefault: () => {}};
|
|
sandbox.stub(element, 'shouldSuppressKeyboardShortcut').returns(false);
|
|
element._lastGKeyPressTimestamp = null;
|
|
element._jumpKeyPressed(e);
|
|
assert.isFalse(navStub.called);
|
|
});
|
|
|
|
test('g key too long ago', () => {
|
|
const e = {detail: {key: 'a'}, preventDefault: () => {}};
|
|
sandbox.stub(element, 'shouldSuppressKeyboardShortcut').returns(false);
|
|
element._lastGKeyPressTimestamp = 3000;
|
|
element._jumpKeyPressed(e);
|
|
assert.isFalse(navStub.called);
|
|
});
|
|
|
|
test('should suppress', () => {
|
|
const e = {detail: {key: 'a'}, preventDefault: () => {}};
|
|
sandbox.stub(element, 'shouldSuppressKeyboardShortcut').returns(true);
|
|
element._lastGKeyPressTimestamp = 9000;
|
|
element._jumpKeyPressed(e);
|
|
assert.isFalse(navStub.called);
|
|
});
|
|
|
|
test('unrecognized key', () => {
|
|
const e = {detail: {key: 'f'}, preventDefault: () => {}};
|
|
sandbox.stub(element, 'shouldSuppressKeyboardShortcut').returns(false);
|
|
element._lastGKeyPressTimestamp = 9000;
|
|
element._jumpKeyPressed(e);
|
|
assert.isFalse(navStub.called);
|
|
});
|
|
});
|
|
});
|
|
</script>
|