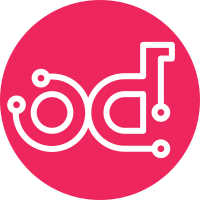
These tags are preserved by the Closure compiler and vulcanize in order to serve the license notices embedded in the outputs. In a standalone Gerrit server, these license are also covered in the LICENSES.txt served with the documentation. When serving PG assets from a CDN, it's less obvious what the corresponding LICENSES.txt file is, since the CDN is not directly linked to a running Gerrit server. Safer to embed the licenses in the assets themselves. Change-Id: Id1add1451fad1baa7916882a6bda02c326ccc988
136 lines
4.6 KiB
HTML
136 lines
4.6 KiB
HTML
<!DOCTYPE html>
|
|
<!--
|
|
@license
|
|
Copyright (C) 2017 The Android Open Source Project
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
-->
|
|
|
|
<meta name="viewport" content="width=device-width, minimum-scale=1.0, initial-scale=1.0, user-scalable=yes">
|
|
<title>gr-autocomplete-dropdown</title>
|
|
|
|
<script src="../../../bower_components/webcomponentsjs/webcomponents-lite.min.js"></script>
|
|
<script src="../../../bower_components/web-component-tester/browser.js"></script>
|
|
<link rel="import" href="../../../test/common-test-setup.html"/>
|
|
<link rel="import" href="gr-autocomplete-dropdown.html">
|
|
|
|
<script>void(0);</script>
|
|
|
|
<test-fixture id="basic">
|
|
<template>
|
|
<gr-autocomplete-dropdown></gr-autocomplete-dropdown>
|
|
</template>
|
|
</test-fixture>
|
|
|
|
<script>
|
|
suite('gr-autocomplete-dropdown', () => {
|
|
let element;
|
|
let sandbox;
|
|
|
|
setup(() => {
|
|
sandbox = sinon.sandbox.create();
|
|
element = fixture('basic');
|
|
element.open();
|
|
element.suggestions = [
|
|
{dataValue: 'test value 1', name: 'test name 1', text: 1},
|
|
{dataValue: 'test value 2', name: 'test name 2', text: 2}];
|
|
flushAsynchronousOperations();
|
|
});
|
|
|
|
teardown(() => {
|
|
sandbox.restore();
|
|
if (element.isOpen) element.close();
|
|
});
|
|
|
|
test('escape key', done => {
|
|
const closeSpy = sandbox.spy(element, 'close');
|
|
MockInteractions.pressAndReleaseKeyOn(element, 27);
|
|
flushAsynchronousOperations();
|
|
assert.isTrue(closeSpy.called);
|
|
done();
|
|
});
|
|
|
|
test('tab key', () => {
|
|
const handleTabSpy = sandbox.spy(element, '_handleTab');
|
|
const itemSelectedStub = sandbox.stub();
|
|
element.addEventListener('item-selected', itemSelectedStub);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 9);
|
|
assert.isTrue(handleTabSpy.called);
|
|
assert.equal(element.$.cursor.index, 0);
|
|
assert.isTrue(itemSelectedStub.called);
|
|
assert.deepEqual(itemSelectedStub.lastCall.args[0].detail, {
|
|
trigger: 'tab',
|
|
selected: element.getCursorTarget(),
|
|
});
|
|
});
|
|
|
|
test('enter key', () => {
|
|
const handleEnterSpy = sandbox.spy(element, '_handleEnter');
|
|
const itemSelectedStub = sandbox.stub();
|
|
element.addEventListener('item-selected', itemSelectedStub);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 13);
|
|
assert.isTrue(handleEnterSpy.called);
|
|
assert.equal(element.$.cursor.index, 0);
|
|
assert.deepEqual(itemSelectedStub.lastCall.args[0].detail, {
|
|
trigger: 'enter',
|
|
selected: element.getCursorTarget(),
|
|
});
|
|
});
|
|
|
|
test('down key', () => {
|
|
element.isHidden = true;
|
|
const nextSpy = sandbox.spy(element.$.cursor, 'next');
|
|
MockInteractions.pressAndReleaseKeyOn(element, 40);
|
|
assert.isFalse(nextSpy.called);
|
|
assert.equal(element.$.cursor.index, 0);
|
|
element.isHidden = false;
|
|
MockInteractions.pressAndReleaseKeyOn(element, 40);
|
|
assert.isTrue(nextSpy.called);
|
|
assert.equal(element.$.cursor.index, 1);
|
|
});
|
|
|
|
test('up key', () => {
|
|
element.isHidden = true;
|
|
const prevSpy = sandbox.spy(element.$.cursor, 'previous');
|
|
MockInteractions.pressAndReleaseKeyOn(element, 38);
|
|
assert.isFalse(prevSpy.called);
|
|
assert.equal(element.$.cursor.index, 0);
|
|
element.isHidden = false;
|
|
element.$.cursor.setCursorAtIndex(1);
|
|
assert.equal(element.$.cursor.index, 1);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 38);
|
|
assert.isTrue(prevSpy.called);
|
|
assert.equal(element.$.cursor.index, 0);
|
|
});
|
|
|
|
test('tapping selects item', () => {
|
|
const itemSelectedStub = sandbox.stub();
|
|
element.addEventListener('item-selected', itemSelectedStub);
|
|
|
|
MockInteractions.tap(element.$.suggestions.querySelectorAll('li')[1]);
|
|
flushAsynchronousOperations();
|
|
assert.deepEqual(itemSelectedStub.lastCall.args[0].detail, {
|
|
trigger: 'tap',
|
|
selected: element.$.suggestions.querySelectorAll('li')[1],
|
|
});
|
|
});
|
|
|
|
test('updated suggestions resets cursor stops', () => {
|
|
resetStopsSpy = sandbox.spy(element, '_resetCursorStops');
|
|
element.suggestions = [];
|
|
assert.isTrue(resetStopsSpy.called);
|
|
});
|
|
});
|
|
|
|
</script>
|