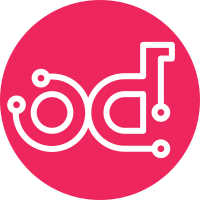
This changes the way the change list view is layed out (flexbox instead of tables) for a couple reasons: + Table elements (td, tr, etc.) cannot be arbitrarily appended to just any DOM node, which caused breakage when I originally attempted to use a <content> tag to bring in the header or group title content into gr-change-list-item. + The flexibility of flexbox allows us to style the change list much easier on smaller screens, so it was probably going to happen anyway. + Full-width (colspan="<total number of columns in the table>") rows for the headers is much more difficult when using elements outside of the gr-change-list-item brought in via <content> as described above. + gr-change-list-item was doing too much anyway. Feature: Issue 3700 Change-Id: I6536bf7d18adfa460507f8050a15d83e84af82a7
149 lines
4.8 KiB
HTML
149 lines
4.8 KiB
HTML
<!DOCTYPE html>
|
|
<!--
|
|
Copyright (C) 2015 The Android Open Source Project
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
-->
|
|
|
|
<meta name="viewport" content="width=device-width, minimum-scale=1.0, initial-scale=1.0, user-scalable=yes">
|
|
<title>gr-change-list</title>
|
|
|
|
<script src="../../bower_components/webcomponentsjs/webcomponents-lite.min.js"></script>
|
|
<script src="../../bower_components/web-component-tester/browser.js"></script>
|
|
<script src="../../bower_components/page/page.js"></script>
|
|
<script src="../scripts/fake-app.js"></script>
|
|
<script src="../scripts/changes.js"></script>
|
|
<script src="../scripts/util.js"></script>
|
|
|
|
<link rel="import" href="../../bower_components/iron-test-helpers/iron-test-helpers.html">
|
|
<link rel="import" href="../elements/gr-change-list.html">
|
|
|
|
<test-fixture id="basic">
|
|
<template>
|
|
<gr-change-list></gr-change-list>
|
|
</template>
|
|
</test-fixture>
|
|
|
|
<test-fixture id="grouped">
|
|
<template>
|
|
<gr-change-list></gr-change-list>
|
|
</template>
|
|
</test-fixture>
|
|
|
|
<script>
|
|
suite('gr-change-list basic tests', function() {
|
|
var element;
|
|
|
|
setup(function() {
|
|
element = fixture('basic');
|
|
});
|
|
|
|
test('keyboard shortcuts', function() {
|
|
element.changes = [
|
|
{_number: 0},
|
|
{_number: 1},
|
|
{_number: 2},
|
|
];
|
|
flushAsynchronousOperations();
|
|
var elementItems = Polymer.dom(element.root).querySelectorAll(
|
|
'gr-change-list-item');
|
|
assert.equal(elementItems.length, 3);
|
|
assert.equal(element.selectedIndex, 0);
|
|
|
|
MockInteractions.pressAndReleaseKeyOn(element, 74); // 'j'
|
|
assert.equal(element.selectedIndex, 1);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 74); // 'j'
|
|
|
|
var showStub = sinon.stub(page, 'show');
|
|
assert.equal(element.selectedIndex, 2);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 13); // 'enter'
|
|
assert(showStub.lastCall.calledWithExactly('/c/2/'),
|
|
'Should navigate to /c/2/');
|
|
|
|
MockInteractions.pressAndReleaseKeyOn(element, 75); // 'k'
|
|
assert.equal(element.selectedIndex, 1);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 13); // 'enter'
|
|
assert(showStub.lastCall.calledWithExactly('/c/1/'),
|
|
'Should navigate to /c/1/');
|
|
|
|
MockInteractions.pressAndReleaseKeyOn(element, 75); // 'k'
|
|
MockInteractions.pressAndReleaseKeyOn(element, 75); // 'k'
|
|
MockInteractions.pressAndReleaseKeyOn(element, 75); // 'k'
|
|
assert.equal(element.selectedIndex, 0);
|
|
|
|
showStub.restore();
|
|
});
|
|
|
|
});
|
|
|
|
suite('gr-change-list groups', function() {
|
|
var element;
|
|
|
|
setup(function() {
|
|
element = fixture('basic');
|
|
});
|
|
|
|
test('keyboard shortcuts', function() {
|
|
element.groups = [
|
|
[
|
|
{_number: 0},
|
|
{_number: 1},
|
|
{_number: 2},
|
|
],
|
|
[
|
|
{_number: 3},
|
|
{_number: 4},
|
|
{_number: 5},
|
|
],
|
|
[
|
|
{_number: 6},
|
|
{_number: 7},
|
|
{_number: 8},
|
|
]
|
|
];
|
|
element.groupTitles = ['Group 1', 'Group 2', 'Group 3'];
|
|
flushAsynchronousOperations();
|
|
var elementItems = Polymer.dom(element.root).querySelectorAll(
|
|
'gr-change-list-item');
|
|
assert.equal(elementItems.length, 9);
|
|
assert.equal(element.selectedIndex, 0);
|
|
|
|
MockInteractions.pressAndReleaseKeyOn(element, 74); // 'j'
|
|
assert.equal(element.selectedIndex, 1);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 74); // 'j'
|
|
|
|
var showStub = sinon.stub(page, 'show');
|
|
assert.equal(element.selectedIndex, 2);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 13); // 'enter'
|
|
assert(showStub.lastCall.calledWithExactly('/c/2/'),
|
|
'Should navigate to /c/2/');
|
|
|
|
MockInteractions.pressAndReleaseKeyOn(element, 75); // 'k'
|
|
assert.equal(element.selectedIndex, 1);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 13); // 'enter'
|
|
assert(showStub.lastCall.calledWithExactly('/c/1/'),
|
|
'Should navigate to /c/1/');
|
|
|
|
MockInteractions.pressAndReleaseKeyOn(element, 74); // 'j'
|
|
MockInteractions.pressAndReleaseKeyOn(element, 74); // 'j'
|
|
MockInteractions.pressAndReleaseKeyOn(element, 74); // 'j'
|
|
assert.equal(element.selectedIndex, 4);
|
|
MockInteractions.pressAndReleaseKeyOn(element, 13); // 'enter'
|
|
assert(showStub.lastCall.calledWithExactly('/c/4/'),
|
|
'Should navigate to /c/4/');
|
|
showStub.restore();
|
|
});
|
|
|
|
});
|
|
</script>
|