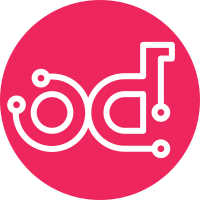
- use _account_id for fetching avatar images - use _account_id as fallback for computing owner link - email address is an optional information (e.g. not available for account 'Diffy Cuckoo (Automated Verifier)' on gerrit-review.googlesource.com) Change-Id: Id02be88a7e859e831a477b34828e8186629a6216
183 lines
4.8 KiB
HTML
183 lines
4.8 KiB
HTML
<!--
|
|
Copyright (C) 2015 The Android Open Source Project
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
-->
|
|
|
|
<link rel="import" href="../bower_components/polymer/polymer.html">
|
|
<link rel="import" href="gr-comment-list.html">
|
|
<link rel="import" href="gr-date-formatter.html">
|
|
|
|
<dom-module id="gr-message">
|
|
<template>
|
|
<style>
|
|
:host {
|
|
border-top: 1px solid #ddd;
|
|
display: block;
|
|
position: relative;
|
|
}
|
|
:host(:not([expanded])) {
|
|
cursor: pointer;
|
|
}
|
|
.avatar {
|
|
border-radius: 50%;
|
|
position: absolute;
|
|
left: var(--default-horizontal-margin);
|
|
}
|
|
:not(.hideAvatar):not(.showAvatar) .avatar,
|
|
.hideAvatar .avatar {
|
|
display: none;
|
|
}
|
|
.collapsed .contentContainer {
|
|
color: #777;
|
|
white-space: nowrap;
|
|
overflow-x: hidden;
|
|
text-overflow: ellipsis;
|
|
}
|
|
.showAvatar.expanded .contentContainer {
|
|
margin-left: calc(var(--default-horizontal-margin) + 2.5em);
|
|
padding: 10px 0;
|
|
}
|
|
.showAvatar.collapsed .contentContainer {
|
|
margin-left: calc(var(--default-horizontal-margin) + 1.75em);
|
|
padding: 10px 75px 10px 0;
|
|
}
|
|
.hideAvatar.collapsed .contentContainer,
|
|
.hideAvatar.expanded .contentContainer {
|
|
margin-left: 0;
|
|
padding: 10px 75px 10px 0;
|
|
}
|
|
.collapsed .avatar {
|
|
top: 8px;
|
|
}
|
|
.expanded .avatar {
|
|
top: 12px;
|
|
}
|
|
.collapsed .avatar {
|
|
height: 1.75em;
|
|
width: 1.75em;
|
|
}
|
|
.expanded .avatar {
|
|
height: 2.5em;
|
|
width: 2.5em;
|
|
}
|
|
.name {
|
|
font-weight: bold;
|
|
}
|
|
.collapsed .name,
|
|
.collapsed .content,
|
|
.collapsed .message {
|
|
display: inline;
|
|
}
|
|
.collapsed gr-comment-list {
|
|
display: none;
|
|
}
|
|
.collapsed .name,
|
|
.collapsed gr-date-formatter {
|
|
color: var(--default-text-color);
|
|
}
|
|
.expanded .name {
|
|
cursor: pointer;
|
|
}
|
|
.expanded .message {
|
|
white-space: pre-wrap;
|
|
}
|
|
gr-date-formatter {
|
|
position: absolute;
|
|
right: var(--default-horizontal-margin);
|
|
top: 10px;
|
|
}
|
|
</style>
|
|
<div class$="[[_computeClass(expanded, showAvatar)]]">
|
|
<img class="avatar" src$="[[_computeAvatarURL(message.author, showAvatar)]]">
|
|
<div class="contentContainer">
|
|
<div class="name" id="name">[[message.author.name]]</div>
|
|
<div class="content">
|
|
<div class="message">[[message.message]]</div>
|
|
<gr-comment-list
|
|
comments="[[comments]]"
|
|
change-num="[[changeNum]]"
|
|
patch-num="[[message._revision_number]]"></gr-comment-list>
|
|
</div>
|
|
<gr-date-formatter date-str="[[message.date]]"></gr-date-formatter>
|
|
</div>
|
|
</div>
|
|
</template>
|
|
<script>
|
|
(function() {
|
|
'use strict';
|
|
|
|
Polymer({
|
|
is: 'gr-message',
|
|
|
|
listeners: {
|
|
'tap': '_tapHandler',
|
|
'name.tap': '_collapseHandler',
|
|
},
|
|
|
|
properties: {
|
|
changeNum: Number,
|
|
message: Object,
|
|
comments: {
|
|
type: Object,
|
|
observer: '_commentsChanged',
|
|
},
|
|
expanded: {
|
|
type: Boolean,
|
|
value: true,
|
|
reflectToAttribute: true,
|
|
},
|
|
showAvatar: {
|
|
type: Boolean,
|
|
value: false,
|
|
},
|
|
},
|
|
|
|
ready: function() {
|
|
app.configReady.then(function(cfg) {
|
|
this.showAvatar = !!(cfg && cfg.plugin && cfg.plugin.has_avatars);
|
|
}.bind(this));
|
|
},
|
|
|
|
_commentsChanged: function(value) {
|
|
this.expanded = Object.keys(value || {}).length > 0;
|
|
},
|
|
|
|
_tapHandler: function(e) {
|
|
if (this.expanded) { return; }
|
|
this.expanded = true;
|
|
},
|
|
|
|
_collapseHandler: function(e) {
|
|
if (!this.expanded) { return; }
|
|
e.stopPropagation();
|
|
this.expanded = false;
|
|
},
|
|
|
|
_computeClass: function(expanded, showAvatar) {
|
|
var classes = [];
|
|
classes.push(expanded ? 'expanded' : 'collapsed');
|
|
classes.push(showAvatar ? 'showAvatar' : 'hideAvatar');
|
|
return classes.join(' ');
|
|
},
|
|
|
|
_computeAvatarURL: function(author, showAvatar) {
|
|
if (!showAvatar || !author) { return '' }
|
|
return '/accounts/' + author._account_id + '/avatar?s=100';
|
|
},
|
|
|
|
});
|
|
})();
|
|
</script>
|
|
</dom-module>
|