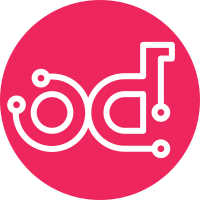
Currently only predefined Maven repositories are supported by Buck maven_jar. Additional logic exists to redirect to a local repository mirror. Current implementation relies on the repository name matching between repo passed to maven_jar and redirect definition defined in local.properties that is not under Git control. This change extends that by allowing to pass not only the repo name but the complete URL to maven_jar. The augmented implementation checks if it is a known Maven repository: if it is, then the behavious is unchanged, if not, then the passed URL is used. As result plugin's can use custom Maven repositories: GERRIT_FORGE = 'http://gerritforge.com/snapshot' maven_jar( name = 'gitblit', id = 'com.gitblit:gitblit:1.4.0', sha1 = '1b130dbf5578ace37507430a4a523f6594bf34fa', license = 'Apache2.0', repository = GERRIT_FORGE, ) Plugin owned Maven repositories can also be rewritten in local.properties. To achieve that custom repository name must be passed to the maven_jar() function, like known repositories, and the URL must be defined in local.properties. local.properties excerpt: download.GERRIT_FORGE = http://my.company.mirror/gerrit-forge BUCK excerpt: GERRIT_FORGE = 'GERRIT_FORGE:' maven_jar( name = 'gitblit', id = 'com.gitblit:gitblit:1.4.0', sha1 = '1b130dbf5578ace37507430a4a523f6594bf34fa', license = 'Apache2.0', repository = GERRIT_FORGE, ) Python unit test can be executed with other Java unit tests: buck test tools:python_tests Change-Id: Ib31d51f0884b1ca1a07b6492f861f404db115946
68 lines
1.9 KiB
Java
68 lines
1.9 KiB
Java
// Copyright (C) 2013 The Android Open Source Project
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
import static org.junit.Assert.assertTrue;
|
|
|
|
import com.google.common.io.ByteStreams;
|
|
import com.google.common.base.Splitter;
|
|
|
|
import java.io.File;
|
|
import java.io.IOException;
|
|
import java.io.InputStream;
|
|
import org.junit.Test;
|
|
|
|
public class PythonTestCaller {
|
|
|
|
@Test
|
|
public void resolveUrl() throws Exception {
|
|
PythonTestCaller.pythonUnit("tools", "util_test");
|
|
}
|
|
|
|
private static void pythonUnit(String d, String sut) throws Exception {
|
|
ProcessBuilder b =
|
|
new ProcessBuilder(Splitter.on(' ').splitToList(
|
|
"python -m unittest " + sut))
|
|
.directory(new File(d))
|
|
.redirectErrorStream(true);
|
|
Process p = null;
|
|
InputStream i = null;
|
|
byte[] out;
|
|
try {
|
|
p = b.start();
|
|
i = p.getInputStream();
|
|
out = ByteStreams.toByteArray(i);
|
|
} catch (IOException e) {
|
|
throw new Exception(e);
|
|
} finally {
|
|
if (p != null) {
|
|
p.getOutputStream().close();
|
|
}
|
|
if (i != null) {
|
|
i.close();
|
|
}
|
|
}
|
|
int value;
|
|
try {
|
|
value = p.waitFor();
|
|
} catch (InterruptedException e) {
|
|
throw new Exception("interrupted waiting for process");
|
|
}
|
|
String err = new String(out, "UTF-8");
|
|
if (value != 0) {
|
|
System.err.print(err);
|
|
}
|
|
assertTrue(err, value == 0);
|
|
}
|
|
}
|