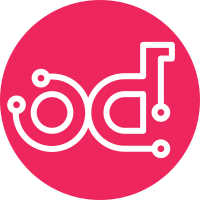
Also hide the changes via the hidden attribute upon initial load. The evaluation of hidden$= will set it correctly. Change-Id: I7d3207c622e03988da2c3020a2c698811b299f9a
146 lines
3.7 KiB
HTML
146 lines
3.7 KiB
HTML
<!--
|
|
Copyright (C) 2015 The Android Open Source Project
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
-->
|
|
|
|
<link rel="import" href="../bower_components/polymer/polymer.html">
|
|
<link rel="import" href="gr-ajax.html">
|
|
<link rel="import" href="gr-change-list.html">
|
|
|
|
<dom-module id="gr-change-list-view">
|
|
<template>
|
|
<style>
|
|
:host {
|
|
background-color: var(--view-background-color);
|
|
display: block;
|
|
margin: 0 1.25rem;
|
|
}
|
|
.loading {
|
|
margin-top: 1em;
|
|
color: #666;
|
|
background-color: #f1f2f3;
|
|
}
|
|
gr-change-list {
|
|
margin-top: 1em;
|
|
width: 100%;
|
|
}
|
|
nav {
|
|
margin-bottom: 1em;
|
|
padding: .5em 0;
|
|
text-align: center;
|
|
}
|
|
nav a {
|
|
display: inline-block;
|
|
}
|
|
nav a:first-of-type {
|
|
margin-right: .5em;
|
|
}
|
|
[hidden] {
|
|
display: none !important;
|
|
}
|
|
</style>
|
|
<gr-ajax
|
|
auto
|
|
url="/changes/"
|
|
params="[[_computeQueryParams(query, offset)]]"
|
|
last-response="{{_changes}}"
|
|
loading="{{_loading}}"></gr-ajax>
|
|
<div class="loading" hidden$="[[!_loading]]">Loading...</div>
|
|
<div hidden$="[[_loading]]" hidden>
|
|
<gr-change-list changes="{{_changes}}"></gr-change-list>
|
|
<nav>
|
|
<a href$="[[_computeNavLink(query, offset, -1)]]"
|
|
hidden$="[[_hidePrevArrow(offset)]]">← Prev</a>
|
|
<a href$="[[_computeNavLink(query, offset, 1)]]"
|
|
hidden$="[[_hideNextArrow(_changes.length)]]">Next →</a>
|
|
</nav>
|
|
</div>
|
|
</template>
|
|
<script>
|
|
(function() {
|
|
'use strict';
|
|
|
|
var DEFAULT_NUM_CHANGES = 25;
|
|
|
|
Polymer({
|
|
is: 'gr-change-list-view',
|
|
|
|
properties: {
|
|
/**
|
|
* URL params passed from the router.
|
|
*/
|
|
params: {
|
|
type: Object,
|
|
observer: '_paramsChanged',
|
|
},
|
|
|
|
/**
|
|
* Change objects loaded from the server.
|
|
*/
|
|
_changes: Array,
|
|
|
|
/**
|
|
* For showing a "loading..." string during ajax requests.
|
|
*/
|
|
_loading: {
|
|
type: Boolean,
|
|
value: true,
|
|
},
|
|
},
|
|
|
|
_paramsChanged: function(value) {
|
|
this.query = value.query;
|
|
this.offset = value.offset || 0;
|
|
},
|
|
|
|
_computeQueryParams: function(query, offset) {
|
|
var options = Changes.listChangesOptionsToHex(
|
|
Changes.ListChangesOption.LABELS,
|
|
Changes.ListChangesOption.DETAILED_ACCOUNTS
|
|
);
|
|
var obj = {
|
|
n: DEFAULT_NUM_CHANGES, // Number of results to return.
|
|
O: options,
|
|
S: offset || 0,
|
|
};
|
|
if (query && query.length > 0) {
|
|
obj.q = query;
|
|
}
|
|
return obj;
|
|
},
|
|
|
|
_computeNavLink: function(query, offset, direction) {
|
|
// Offset could be a string when passed from the router.
|
|
offset = +(offset || 0);
|
|
var newOffset = Math.max(0, offset + (25 * direction));
|
|
var href = '/q/' + query;
|
|
if (newOffset > 0) {
|
|
href += ',' + newOffset;
|
|
}
|
|
return href;
|
|
},
|
|
|
|
_hidePrevArrow: function(offset) {
|
|
return offset == 0;
|
|
},
|
|
|
|
_hideNextArrow: function(changesLen) {
|
|
return changesLen < DEFAULT_NUM_CHANGES;
|
|
},
|
|
|
|
});
|
|
})();
|
|
</script>
|
|
</dom-module>
|