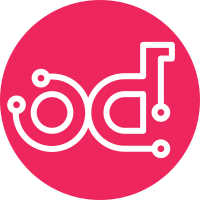
This is the first step in building a public JavaScript API that can be utilized by plugins to manage interaction with the web UI. This commit makes the following plugin JavaScript possible: Gerrit.install(function(self) { function onCallMe(c) { var f = c.textfield(); var t = c.checkbox(); var b = c.button('Phone', {onclick: function(){ c.call( {message: f.value, dial: t.checked}, function(r) { c.hide(); window.alert("Said " + r); c.refresh(); }); }}); c.popup(c.div( f, c.br(), c.label(t, 'Dial'), c.br(), b)); f.focus(); } self.onAction('revision', 'callme', onCallMe); }); JavaScript plugins are encouraged to protect the global namespace by running Gerrit.install(f), passing an anonymous function Gerrit can call to initialize the plugin. Gerrit.install() performs some black magic to identify which plugin the JavaScript is running from, exporting as Gerrit.getPluginName(). Obtaining the name is faster within the install() invocation as the string is cached in a global variable. A plugin's install function is given a reference to the plugin JavaScript object. This object has many helper methods to support communication with the server. Gerrit.onAction() accepts the type of view and the RestView name as defined in the plugin and a JavaScript function to execute when the user has activated the action button. An action callback is given a context object declaring a number of useful properties: change: The ChangeInfo object. revision: The RevisionInfo object. action: The ActionInfo object that invoked this callback. popup(e): Display a popup containing element e. hide(): Hide the popup that was created by popup. call(in,cb): Executes the RPC using action.method. get(cb): Executes the RPC using GET. post(in,cb): Executes the RPC using POST. put(in,cb): Executes the RPC using PUT. delete(cb): Executes the RPC using DELETE. HTML: br, hr, button, checkbox, div, label, span, textfield These make it easier to construct a tiny UI for use in a popup, such as gathering a small amount if input from the user to supply to the RPC. go(token): Navigate the web UI to the application URL. refresh(): Redisplay the current web UI view. Any result data from the server is parsed as JSON and passed as-is to the callback function. A JSON string result is given as a string, a JSON object or array is passed as-is. A corresponding REST API view on revisions can be declared: class Module extends RestApiModule { @Override protected void configure() { post(RevisionResource.REVISION_KIND, "callme").to(CallMe.class); } } class CallMe implements RestModifyView<RevisionResource, CallMe.Input>, UiAction<RevisionResource> { static class Input { String message; } @Override public String apply(RevisionResource resource, Input input) { return "You said: " + input.message; } @Override public UiAction.Description getDescription(RevisionResource resource) { return new UiAction.Description().setLabel("Call Me"); } } Currently the JavaScript code must be registered at the server side to appear in the host page download: class Web extends AbstractModule { @Override protected void configure() { DynamicSet.bind(binder(), WebUiPlugin.class) .toInstance(new JavaScriptPlugin("maybe.js")); } I find this binding method for JavaScript code is awkard and would like to improve on it in the future. Finally both modules must be registered in the plugin manifest: gerrit_plugin( name = 'actiondemo', srcs = glob(['src/main/java/**/*.java']), resources = glob(['src/main/resources/**/*']), manifest_entries = [ "Gerrit-Module: com.googlesource.gerrit.plugins.actiondemo.Module", "Gerrit-HttpModule: com.googlesource.gerrit.plugins.actiondemo.Web", ], ) Change-Id: I2d572279ac978e644772b1cedbecc080746a2306
90 lines
3.0 KiB
Plaintext
90 lines
3.0 KiB
Plaintext
Gerrit Code Review for Git
|
|
==========================
|
|
|
|
Index
|
|
-----
|
|
|
|
. General info
|
|
.. link:licenses.html[Licenses and Notices]
|
|
. Installing
|
|
.. link:intro-quick.html[A Quick Introduction To Gerrit]
|
|
.. link:install.html[Installation Guide]
|
|
. Tutorial
|
|
.. Get started
|
|
... External link: link:http://source.android.com/submit-patches/workflow[Default Android Workflow]
|
|
.. Web
|
|
... Registering a new Gerrit account
|
|
... link:user-search.html[Searching Changes]
|
|
... link:user-notify.html[Subscribing to Email Notifications]
|
|
.. Ssh
|
|
... ssh connection details
|
|
... link:cmd-index.html[Command Line Tools]
|
|
.. Git
|
|
... git connection details
|
|
... Commands, scenarios
|
|
.... link:user-upload.html[Uploading Changes]
|
|
.... link:error-messages.html[Error Messages]
|
|
... Changes
|
|
.... link:user-changeid.html[Change-Id Lines]
|
|
.... link:user-signedoffby.html[Signed-off-by Lines]
|
|
... Patch sets
|
|
. Project management
|
|
.. link:project-setup.html[Project Setup]
|
|
.. link:access-control.html[Access Controls]
|
|
... link:config-labels.html[Review Labels]
|
|
.. Multi-project management
|
|
... Submodules
|
|
... Repo
|
|
.. Prolog rules
|
|
... link:prolog-cookbook.html[Prolog Cookbook]
|
|
... link:prolog-change-facts.html[Prolog Facts for Gerrit Changes]
|
|
.. link:user-submodules.html[Subscribing to Git Submodules]
|
|
.. Project sunset
|
|
. Customization and integration
|
|
.. link:user-dashboards.html[Dashboards]
|
|
.. link:rest-api.html[REST API]
|
|
.. link:config-gitweb.html[Gitweb Integration]
|
|
.. link:config-themes.html[Themes]
|
|
.. link:config-sso.html[Single Sign-On Systems]
|
|
.. link:config-hooks.html[Hooks]
|
|
.. link:config-mail.html[Mail Templates]
|
|
.. link:config-cla.html[Contributor Agreements]
|
|
. Server administration
|
|
.. link:config-gerrit.html[System Settings]
|
|
.. Backup
|
|
.. Performance tuning
|
|
... link:cmd-index.html[Command Line Tools]
|
|
... Reading show-caches efficiently
|
|
... How to read stats from the JVM
|
|
.. High availability
|
|
.. Replication
|
|
.. Plugins
|
|
.. link:dev-design.html[System Design]
|
|
.. link:config-contact.html[User Contact Information]
|
|
.. link:config-reverseproxy.html[Reverse Proxy]
|
|
.. link:pgm-index.html[Server Side Administrative Tools]
|
|
. Developer
|
|
.. link:dev-readme.html[Developer Setup]
|
|
.. link:dev-eclipse.html[Eclipse Setup]
|
|
.. link:dev-contributing.html[Contributing to Gerrit]
|
|
.. Documentation formatting guide for contributions
|
|
.. link:dev-design.html[System Design]
|
|
.. link:i18n-readme.html[i18n Support]
|
|
.. Plugin development
|
|
... link:dev-plugins.html[Developing Plugins]
|
|
... link:js-api.html[JavaScript Plugin API]
|
|
... link:config-validation.html[Commit Validation]
|
|
. Maintainer
|
|
.. link:dev-release.html[Developer Release]
|
|
.. link:dev-release-subproject.html[Developer Subproject Release]
|
|
|
|
|
|
Resources
|
|
---------
|
|
|
|
* link:http://code.google.com/p/gerrit/[Homepage]
|
|
* link:http://code.google.com/p/gerrit/downloads/list[Downloads]
|
|
* link:http://code.google.com/p/gerrit/issues/list[Issue Tracking]
|
|
* link:http://code.google.com/p/gerrit/source/checkout[Source Code]
|
|
* link:http://code.google.com/p/gerrit/wiki/Background[A History of Gerrit Code Review]
|