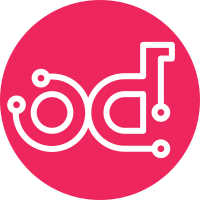
ORIGINALLY: Iafd4b80af53624027c347f0f443b4cdfde292e29 This change makes the change view and the diff view change edit aware. Mutation operations on edit itself, like editing files in editor, are beyond the scope of this change. Change edits must be fetched with a separate change edit endpoint and merged into the change.revisions object. The _number of an editInfo is 'edit'. It has a special property called 'basePatchNum' that marks which patch set the edit is based on. In patch set selectors, the edit is sorted right after its basePatchNum. Alternative implementation considerations: It could be easier to handle edits on the Polygerrit UI, if GET /changes/<id>/detail endpoint would optionally include the change edit in the resulting change.revsions map. TODOs: * Overwrite change.current_revision with change edit commit in some use cases * Mark the change edit as Change Edit in the header of the change view * Allow for modification of the edit in the change/diff view * Disable commenting on files in edit patchsets * Modify file list rows to have appropriate actions when edit is selected (e.g. allow revert, disable marking as reviewed) * Identify and whitelist valid change/revision actions for an edit Bug: Issue 4437 Change-Id: Ia4690d20954de730cd625ac76920e849beb12f7c
95 lines
3.1 KiB
JavaScript
95 lines
3.1 KiB
JavaScript
// Copyright (C) 2016 The Android Open Source Project
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
(function() {
|
|
'use strict';
|
|
|
|
// Maximum length for patch set descriptions.
|
|
const PATCH_DESC_MAX_LENGTH = 500;
|
|
|
|
Polymer({
|
|
is: 'gr-patch-range-select',
|
|
|
|
properties: {
|
|
availablePatches: Array,
|
|
changeNum: String,
|
|
filesWeblinks: Object,
|
|
path: String,
|
|
patchRange: {
|
|
type: Object,
|
|
observer: '_updateSelected',
|
|
},
|
|
revisions: Object,
|
|
_sortedRevisions: Array,
|
|
_rightSelected: String,
|
|
_leftSelected: String,
|
|
},
|
|
|
|
observers: ['_updateSortedRevisions(revisions.*)'],
|
|
|
|
behaviors: [Gerrit.PatchSetBehavior],
|
|
|
|
_updateSelected() {
|
|
this._rightSelected = this.patchRange.patchNum;
|
|
this._leftSelected = this.patchRange.basePatchNum;
|
|
},
|
|
|
|
_updateSortedRevisions(revisionsRecord) {
|
|
const revisions = revisionsRecord.base;
|
|
this._sortedRevisions = this.sortRevisions(Object.values(revisions));
|
|
},
|
|
|
|
_handlePatchChange(e) {
|
|
const leftPatch = this._leftSelected;
|
|
const rightPatch = this._rightSelected;
|
|
let rangeStr = rightPatch;
|
|
if (leftPatch != 'PARENT') {
|
|
rangeStr = leftPatch + '..' + rangeStr;
|
|
}
|
|
page.show('/c/' + this.changeNum + '/' + rangeStr + '/' + this.path);
|
|
e.target.blur();
|
|
},
|
|
|
|
_computeLeftDisabled(patchNum, patchRange) {
|
|
return this.findSortedIndex(patchNum, this._sortedRevisions) >=
|
|
this.findSortedIndex(patchRange.patchNum, this._sortedRevisions);
|
|
},
|
|
|
|
_computeRightDisabled(patchNum, patchRange) {
|
|
if (patchRange.basePatchNum == 'PARENT') { return false; }
|
|
|
|
return this.findSortedIndex(patchNum, this._sortedRevisions) <=
|
|
this.findSortedIndex(patchRange.basePatchNum, this._sortedRevisions);
|
|
},
|
|
|
|
// On page load, the dom-if for options getting added occurs after
|
|
// the value was set in the select. This ensures that after they
|
|
// are loaded, the correct value will get selected. I attempted to
|
|
// debounce these, but because they are detecting two different
|
|
// events, sometimes the timing was off and one ended up missing.
|
|
_synchronizeSelectionRight() {
|
|
this.$.rightPatchSelect.value = this._rightSelected;
|
|
},
|
|
|
|
_synchronizeSelectionLeft() {
|
|
this.$.leftPatchSelect.value = this._leftSelected;
|
|
},
|
|
|
|
_computePatchSetDescription(revisions, patchNum) {
|
|
const rev = this.getRevisionByPatchNum(revisions, patchNum);
|
|
return (rev && rev.description) ?
|
|
rev.description.substring(0, PATCH_DESC_MAX_LENGTH) : '';
|
|
},
|
|
});
|
|
})();
|