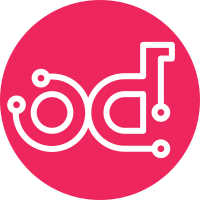
This change establishes Python 3 compatibility for the major part of
Gerrit build tool chain. Only two scripts remain non Python 3
compatible:
* Documentation/replace_macros.py
* tools/bzl/license-map.py
Those scripts explicitly invoked with python2 version.
Test Plan:
a. Python 2.7
* Switch to system where /usr/bin/python points to Python 2.7
* bazel build release
* bazel test //...
* tools/eclipse/project.py
b. Python 3.6
* Switch to system where /usr/bin/python points to Python 3.6
* bazel build release
* bazel test //...
* tools/eclipse/project.py
Pre-requisites for the test plan:
In case bazel action and repository caching is activated on the SUT, the
caches would need to be wiped out, to make sure that the complete build
tool chain was tested. On my system I had to run these commands:
* bazel clean --expunge_async
* rm -rf ~/.gerritcodereview/buck-cache/downloaded-artifacts/*
* rm -rf ~/.gerritcodereview/bazel-cache/cas/*
* rm -rf ~/.gerritcodereview/bazel-cache/repository/*
Bug: Issue 8151
Change-Id: Iece59d0c5149b77a02754b3fed4ce84d5d8085ee
(cherry picked from commit 0c9e13c11a
)
75 lines
2.1 KiB
Python
Executable File
75 lines
2.1 KiB
Python
Executable File
#!/usr/bin/env python
|
|
# Copyright (C) 2015 The Android Open Source Project
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
from __future__ import print_function
|
|
|
|
import atexit
|
|
import json
|
|
import os
|
|
import shutil
|
|
import subprocess
|
|
import sys
|
|
import tarfile
|
|
import tempfile
|
|
|
|
|
|
def is_bundled(tar):
|
|
# No entries for directories, so scan for a matching prefix.
|
|
for entry in tar.getmembers():
|
|
if entry.name.startswith('package/node_modules/'):
|
|
return True
|
|
return False
|
|
|
|
|
|
def bundle_dependencies():
|
|
with open('package.json') as f:
|
|
package = json.load(f)
|
|
package['bundledDependencies'] = list(package['dependencies'].keys())
|
|
with open('package.json', 'w') as f:
|
|
json.dump(package, f)
|
|
|
|
|
|
def main(args):
|
|
if len(args) != 2:
|
|
print('Usage: %s <package> <version>' % sys.argv[0], file=sys.stderr)
|
|
return 1
|
|
|
|
name, version = args
|
|
filename = '%s-%s.tgz' % (name, version)
|
|
url = 'http://registry.npmjs.org/%s/-/%s' % (name, filename)
|
|
|
|
tmpdir = tempfile.mkdtemp();
|
|
tgz = os.path.join(tmpdir, filename)
|
|
atexit.register(lambda: shutil.rmtree(tmpdir))
|
|
|
|
subprocess.check_call(['curl', '--proxy-anyauth', '-ksfo', tgz, url])
|
|
with tarfile.open(tgz, 'r:gz') as tar:
|
|
if is_bundled(tar):
|
|
print('%s already has bundled node_modules' % filename)
|
|
return 1
|
|
tar.extractall(path=tmpdir)
|
|
|
|
oldpwd = os.getcwd()
|
|
os.chdir(os.path.join(tmpdir, 'package'))
|
|
bundle_dependencies()
|
|
subprocess.check_call(['npm', 'install'])
|
|
subprocess.check_call(['npm', 'pack'])
|
|
shutil.copy(filename, os.path.join(oldpwd, filename))
|
|
return 0
|
|
|
|
|
|
if __name__ == '__main__':
|
|
sys.exit(main(sys.argv[1:]))
|