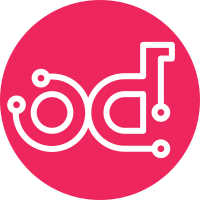
git-review gets its configuration from multiple sources (by priority): * command line options * git-config * options: username, rebase * with local/global/system config * git-review config files * options: scheme, host, port, project, defaultbranch/remote/rebase * locally using .gitreview * globally using ~/.config/gitreview/git-review.conf * system using /etc/git-review/git-review.conf .gitreview file is commonly provided versioned in the git repo, where other git-review config files and git-config is done by developers. It implies for developers multiple places to define local/global/system configuration options but some can only be changed by updating .gitreview (scheme/port...) which is under versioning. This change proposes to use as configuration sources (by priority): * command line options * git-config for developer local/global/system config * options: username, rebase and all .gitreview options * .gitreview local file for repo provided specific config and deprecates git-review global/system files in order to reduce configuration sources and allow to overload .gitreview configuration without updating it. Change-Id: I24aba0fa089de57d51a79049d9c192f76d576f69
90 lines
3.2 KiB
Python
90 lines
3.2 KiB
Python
# -*- coding: utf8 -*-
|
|
|
|
# Copyright (c) 2014 Hewlett-Packard Development Company, L.P.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
|
|
# implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
# Use of io.StringIO in python =< 2.7 requires all strings handled to be
|
|
# unicode. See if StringIO.StringIO is available first
|
|
try:
|
|
import StringIO as io
|
|
except ImportError:
|
|
import io
|
|
import textwrap
|
|
|
|
import mock
|
|
import testtools
|
|
|
|
from git_review import cmd
|
|
|
|
|
|
class ConfigTestCase(testtools.TestCase):
|
|
"""Class testing config behavior."""
|
|
|
|
@mock.patch('git_review.cmd.LOCAL_MODE',
|
|
mock.PropertyMock(return_value=True))
|
|
@mock.patch('git_review.cmd.git_directories', return_value=['', 'fake'])
|
|
@mock.patch('git_review.cmd.run_command_exc')
|
|
def test_git_local_mode(self, run_mock, dir_mock):
|
|
cmd.git_config_get_value('abc', 'def')
|
|
run_mock.assert_called_once_with(
|
|
cmd.GitConfigException,
|
|
'git', 'config', '-f', 'fake/config', '--get', 'abc.def')
|
|
|
|
@mock.patch('git_review.cmd.LOCAL_MODE',
|
|
mock.PropertyMock(return_value=True))
|
|
@mock.patch('os.path.exists', return_value=False)
|
|
def test_gitreview_local_mode(self, exists_mock):
|
|
cmd.Config()
|
|
self.assertFalse(exists_mock.called)
|
|
|
|
|
|
class GitReviewConsole(testtools.TestCase):
|
|
"""Class for testing the console output of git-review."""
|
|
|
|
reviews = [
|
|
{
|
|
'number': '1010101',
|
|
'branch': 'master',
|
|
'subject': 'A simple short subject'
|
|
}, {
|
|
'number': '9877',
|
|
'branch': 'stable/codeword',
|
|
'subject': 'A longer and slightly more wordy subject'
|
|
}, {
|
|
'number': '12345',
|
|
'branch': 'master',
|
|
'subject': 'A ridiculously long subject that can exceed the '
|
|
'normal console width, just need to ensure the '
|
|
'max width is short enough'
|
|
}]
|
|
|
|
@mock.patch('git_review.cmd.query_reviews')
|
|
@mock.patch('git_review.cmd.get_remote_url', mock.MagicMock)
|
|
@mock.patch('git_review.cmd._has_color', False)
|
|
def test_list_reviews_no_blanks(self, mock_query):
|
|
|
|
mock_query.return_value = self.reviews
|
|
with mock.patch('sys.stdout', new_callable=io.StringIO) as output:
|
|
cmd.list_reviews(None)
|
|
console_output = output.getvalue().split('\n')
|
|
|
|
wrapper = textwrap.TextWrapper(replace_whitespace=False,
|
|
drop_whitespace=False)
|
|
for text in console_output:
|
|
for line in wrapper.wrap(text):
|
|
self.assertEqual(line.isspace(), False,
|
|
"Extra blank lines appearing between reviews"
|
|
"in console output")
|