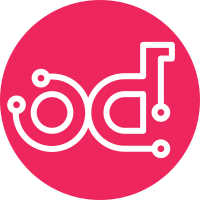
Previously we were checking that myid was written to disk in the expected location. However, it is possible that zk would stop looking in that location for the myid value. To ensure we actually set the value in the running service check the logs for the [myid:4] string. Change-Id: Iee3b126abac13e19dab9ddf4c64ed133d0a98956
83 lines
2.9 KiB
Python
83 lines
2.9 KiB
Python
# Copyright 2020 Red Hat, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import json
|
|
import util
|
|
|
|
|
|
testinfra_hosts = ['zk04.opendev.org']
|
|
|
|
|
|
def test_id_file(host):
|
|
# Test that wacky hostname regex works
|
|
myid = host.file('/var/zookeeper/data/myid')
|
|
assert myid.content == b'4\n'
|
|
|
|
def test_zk_myid_is_set(host):
|
|
# Test that our wacky hostname regex results in the correct
|
|
# myid value in the running service.
|
|
cmd = host.run("docker logs zookeeper-compose_zk_1")
|
|
assert "[myid:4]" in cmd.stdout
|
|
|
|
def test_zk_listening(host):
|
|
zk = host.socket("tcp://0.0.0.0:2181")
|
|
assert zk.is_listening
|
|
|
|
def test_zk_listening_ssl(host):
|
|
zk = host.socket("tcp://0.0.0.0:2281")
|
|
assert zk.is_listening
|
|
|
|
def test_l4_commands(host):
|
|
cmd = host.run("echo srvr | nc localhost 2181")
|
|
assert "Zookeeper version" in cmd.stdout
|
|
assert "not executed because it is not in the whitelist" not in cmd.stdout
|
|
|
|
cmd = host.run("echo stat | nc localhost 2181")
|
|
assert "Zookeeper version" in cmd.stdout
|
|
assert "not executed because it is not in the whitelist" not in cmd.stdout
|
|
|
|
cmd = host.run("echo dump | nc localhost 2181")
|
|
assert "SessionTracker dump" in cmd.stdout
|
|
assert "not executed because it is not in the whitelist" not in cmd.stdout
|
|
|
|
cmd = host.run("echo mntr | nc localhost 2181")
|
|
assert "zk_version" in cmd.stdout
|
|
assert "not executed because it is not in the whitelist" not in cmd.stdout
|
|
|
|
def test_zookeeper_statsd_running(host):
|
|
cmd = host.run("docker inspect zookeeper-compose_zookeeper-statsd_1")
|
|
out = json.loads(cmd.stdout)
|
|
assert out[0]["State"]["Status"] == "running"
|
|
assert out[0]["RestartCount"] == 0
|
|
|
|
def test_zk_2181_accessibility(host):
|
|
# Ask the host to report its own IP addresses. This will use our test
|
|
# local /etc/hosts values and not DNS.
|
|
zk = host.addr("zk04.opendev.org")
|
|
# Verify it is using our local /etc/hosts values
|
|
print(zk.ipv4_addresses)
|
|
print(zk.ipv6_addresses)
|
|
|
|
for addr in zk.ipv4_addresses + zk.ipv6_addresses:
|
|
if addr.startswith("::ffff:"):
|
|
# This is an ipv4 address mapped to ipv6 and is covered by
|
|
# the ipv4_addresses list
|
|
continue
|
|
if addr.startswith("127.") or addr == "::1":
|
|
# We don't want to talk to localhost as we are connecting
|
|
# from our test bridge instance.
|
|
continue
|
|
util.check_unreachable(addr, 2181)
|
|
util.check_unreachable(addr, 2281)
|