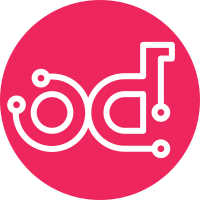
Add a tool to scan a host and generate the sshfp records to go into dns. Hook this into the DNS print out from the node launcher. Change-Id: I686287c3c081debeb6a230e2a3e7b48e5720c65a
49 lines
1.3 KiB
Python
Executable File
49 lines
1.3 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
|
|
import argparse
|
|
import subprocess
|
|
|
|
def generate_sshfp_records(hostname, ip):
|
|
'''Given a hostname and and IP address, scan the IP address (hostname
|
|
not in dns yet) and return a bind string with sshfp records'''
|
|
|
|
s = subprocess.run(['ssh-keyscan', '-D', ip],
|
|
stdout=subprocess.PIPE,
|
|
stderr=subprocess.PIPE).stdout.decode('utf-8')
|
|
|
|
fingerprints = []
|
|
for line in s.split('\n'):
|
|
if not line:
|
|
continue
|
|
_, _, _, algo, key_type, fingerprint = line.split(' ')
|
|
fingerprints.append(
|
|
(algo, key_type, fingerprint))
|
|
|
|
# sort by algo and key_type to keep it consistent
|
|
fingerprints = sorted(fingerprints,
|
|
key=lambda x: (x[0], x[1]))
|
|
|
|
ret = ''
|
|
first = True
|
|
for f in fingerprints:
|
|
ret += '%s%s\t\tIN\tSSHFP\t%s %s %s' % \
|
|
("\n" if not first else '', hostname, f[0], f[1], f[2])
|
|
first = False
|
|
return ret
|
|
|
|
|
|
def sshfp_print_records(hostname, ip):
|
|
print(generate_sshfp_records(hostname, ip))
|
|
|
|
|
|
def main():
|
|
parser = argparse.ArgumentParser()
|
|
parser.add_argument("hostname", help="hostname")
|
|
parser.add_argument("ip", help="address to scan")
|
|
args = parser.parse_args()
|
|
|
|
sshfp_print_records(args.hostname, args.ip)
|
|
|
|
if __name__ == '__main__':
|
|
main()
|