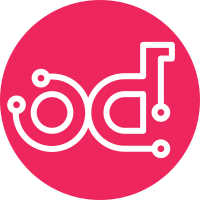
I noticed on our hosts some logrotate files named '*.1234.conf' -- these are coming from callers of logrotate role specifying '/var/log/program/*.log', where the '*' is turning into a literal filename. I didn't really consider this case. Having a file-name starting with '*' may technically be fine, but is a bad idea for everyone's sanity and it's potential to foot-gun some sort of operation that suddenly wipes out a lot more than you wanted to. Let's just use the hash of the name to be unambiguous and still idempotent. Make it more git-ish by using the same 7 digits as a default short-hash. Change-Id: I13d376f85a25a7b8c3a0bc0dcbabd916e8a9774a
147 lines
5.0 KiB
Python
147 lines
5.0 KiB
Python
# Copyright 2018 Red Hat, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import util
|
|
|
|
testinfra_hosts = ['all']
|
|
|
|
|
|
def test_exim_is_installed(host):
|
|
if host.system_info.distribution in ['ubuntu', 'debian']:
|
|
exim = host.package("exim4-base")
|
|
else:
|
|
exim = host.package("exim")
|
|
assert exim.is_installed
|
|
|
|
cmd = host.run("exim -bt root")
|
|
assert cmd.rc == 0
|
|
|
|
|
|
def test_iptables(host):
|
|
rules = util.verify_iptables(host)
|
|
|
|
# Make sure that the zuul console stream rule is still present
|
|
zuul = ('-A openstack-INPUT -p tcp -m state --state NEW'
|
|
' -m tcp --dport 19885 -j ACCEPT')
|
|
assert zuul in rules
|
|
|
|
|
|
def test_ntp(host):
|
|
package = host.package("ntp")
|
|
if host.system_info.codename in ('xenial'):
|
|
assert package.is_installed
|
|
|
|
if host.system_info.distribution in ['ubuntu', 'debian']:
|
|
service = host.service("ntp")
|
|
else:
|
|
service = host.service("ntpd")
|
|
assert service.is_running
|
|
assert service.is_enabled
|
|
|
|
else:
|
|
assert not package.is_installed
|
|
|
|
service = host.service('systemd-timesyncd')
|
|
assert service.is_running
|
|
|
|
# Focal updates the status string to just say NTP
|
|
if host.system_info.codename == 'bionic':
|
|
stdout_string = 'systemd-timesyncd.service active'
|
|
else:
|
|
stdout_string = 'NTP service: active'
|
|
cmd = host.run("timedatectl status")
|
|
assert stdout_string in cmd.stdout
|
|
|
|
|
|
def test_snmp(host):
|
|
service = host.service("snmpd")
|
|
assert service.is_running
|
|
assert service.is_enabled
|
|
|
|
|
|
def test_timezone(host):
|
|
tz = host.check_output('date +%Z')
|
|
assert tz == "UTC"
|
|
|
|
|
|
def test_unbound(host):
|
|
output = host.check_output('host opendev.org')
|
|
assert 'has address' in output
|
|
|
|
|
|
def test_unattended_upgrades(host):
|
|
if host.system_info.distribution in ['ubuntu', 'debian']:
|
|
package = host.package("unattended-upgrades")
|
|
assert package.is_installed
|
|
|
|
package = host.package("mailutils")
|
|
assert package.is_installed
|
|
|
|
cfg_file = host.file("/etc/apt/apt.conf.d/10periodic")
|
|
assert cfg_file.exists
|
|
assert cfg_file.contains('^APT::Periodic::Enable "1"')
|
|
assert cfg_file.contains('^APT::Periodic::Update-Package-Lists "1"')
|
|
assert cfg_file.contains('^APT::Periodic::Download-Upgradeable-Packages "1"')
|
|
assert cfg_file.contains('^APT::Periodic::AutocleanInterval "5"')
|
|
assert cfg_file.contains('^APT::Periodic::Unattended-Upgrade "1"')
|
|
assert cfg_file.contains('^APT::Periodic::RandomSleep "1800"')
|
|
|
|
cfg_file = host.file("/etc/apt/apt.conf.d/20auto-upgrades")
|
|
assert cfg_file.exists
|
|
assert cfg_file.contains('^APT::Periodic::Enable "1"')
|
|
assert cfg_file.contains('^APT::Periodic::Update-Package-Lists "1"')
|
|
assert cfg_file.contains('^APT::Periodic::Download-Upgradeable-Packages "1"')
|
|
assert cfg_file.contains('^APT::Periodic::AutocleanInterval "5"')
|
|
assert cfg_file.contains('^APT::Periodic::Unattended-Upgrade "1"')
|
|
assert cfg_file.contains('^APT::Periodic::RandomSleep "1800"')
|
|
|
|
cfg_file = host.file("/etc/apt/apt.conf.d/50unattended-upgrades")
|
|
assert cfg_file.contains('^Unattended-Upgrade::Mail "root"')
|
|
|
|
else:
|
|
package = host.package("yum-cron")
|
|
assert package.is_installed
|
|
|
|
service = host.service("crond")
|
|
assert service.is_enabled
|
|
assert service.is_running
|
|
|
|
cfg_file = host.file("/etc/yum/yum-cron.conf")
|
|
assert cfg_file.exists
|
|
assert cfg_file.contains('apply_updates = yes')
|
|
|
|
|
|
def test_logrotate(host):
|
|
'''Check for log rotation configuration files
|
|
|
|
The magic number here is [0:5] of the sha1 hash of the full
|
|
path to the rotated logfile; the role adds this for uniqueness.
|
|
'''
|
|
ansible_vars = host.ansible.get_variables()
|
|
if ansible_vars['inventory_hostname'].startswith('bridge'):
|
|
# Generated for idempotence by logrotate role; hash of
|
|
# "/var/log/ansible/ansible.log"
|
|
cfg_file = host.file("/etc/logrotate.d/372374.conf")
|
|
assert cfg_file.exists
|
|
assert cfg_file.contains('/var/log/ansible/ansible.log')
|
|
|
|
|
|
def test_no_recommends(host):
|
|
if host.system_info.distribution in ['ubuntu', 'debian']:
|
|
cfg_file = host.file("/etc/apt/apt.conf.d/95disable-recommends")
|
|
assert cfg_file.exists
|
|
|
|
assert cfg_file.contains('^APT::Install-Recommends "0"')
|
|
assert cfg_file.contains('^APT::Install-Suggests "0"')
|