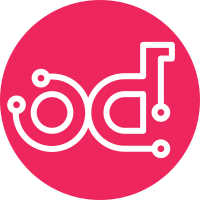
This will help us catch any regressions in our workaround for handling x/ routes in Gerrit. We update the test project in Gerrit to be x/test-project then add a testinfra test to clone it. If we can clone x/test-project then our workaround continues to function. Change-Id: I50e4cb1a5d3c9f7c4405500f09bf6c3be3e7df9c
218 lines
7.4 KiB
YAML
218 lines
7.4 KiB
YAML
- hosts: "review"
|
|
tasks:
|
|
|
|
- name: Wait for Gerrit to be up
|
|
uri:
|
|
url: http://localhost:8081/a/accounts/admin/sshkeys
|
|
method: GET
|
|
user: admin
|
|
password: secret
|
|
register: result
|
|
until: result.status == 200 and not result.redirected
|
|
delay: 1
|
|
retries: 120
|
|
|
|
- name: Create CI group
|
|
uri:
|
|
url: 'http://localhost:8081/a/groups/CI-tools'
|
|
method: PUT
|
|
user: admin
|
|
password: secret
|
|
body_format: json
|
|
body:
|
|
description: 'Continuous Integration Tools'
|
|
status_code: 201
|
|
|
|
- name: Create Zuul user
|
|
uri:
|
|
url: http://localhost:8081/a/accounts/zuul
|
|
method: PUT
|
|
user: admin
|
|
password: secret
|
|
body_format: json
|
|
body:
|
|
name: 'Zuul'
|
|
email: 'zuul@example.com'
|
|
http_password: 'secret'
|
|
status_code: 201
|
|
|
|
- name: Add Zuul to group
|
|
uri:
|
|
url: 'http://localhost:8081/a/groups/CI-tools/members/zuul'
|
|
method: PUT
|
|
user: admin
|
|
password: secret
|
|
status_code: 201
|
|
|
|
- name: Get group UUID
|
|
uri:
|
|
url: 'http://localhost:8081/a/groups/CI-tools'
|
|
method: GET
|
|
user: admin
|
|
password: secret
|
|
status_code: 200
|
|
return_content: yes
|
|
register: group_raw
|
|
|
|
- name: Debug serialized json
|
|
debug:
|
|
var: group_raw
|
|
|
|
- name: Deserialize returned data to internal variable.
|
|
set_fact:
|
|
# The first 4 bytes of the returned data are )]}' which is
|
|
# invalid json.
|
|
group_json: '{{ group_raw.content | regex_replace("^....", "") | from_json }}'
|
|
|
|
- name: Debug deserialized json
|
|
debug:
|
|
var: group_json
|
|
|
|
- name: Setup key and initial gerrit config
|
|
shell:
|
|
executable: /bin/bash
|
|
cmd: |
|
|
set -xe
|
|
|
|
ssh-keygen -t ed25519 -f /root/.ssh/id_25519 -P ""
|
|
curl -X POST --user "admin:secret" -H "Content-Type: text/plain" -d@/root/.ssh/id_25519.pub http://localhost:8081/a/accounts/admin/sshkeys
|
|
ssh-keyscan -p 29418 localhost >> /root/.ssh/known_hosts
|
|
|
|
git config --global user.name "Admin"
|
|
git config --global user.email "admin@example.com"
|
|
|
|
git clone http://admin:secret@localhost:8081/a/All-Projects
|
|
|
|
pushd All-Projects
|
|
|
|
echo "{{ group_json.id }} CI-tools" >> groups # noqa 203
|
|
git commit -a -m "Add CI-tools group"
|
|
git push origin HEAD:refs/meta/config
|
|
|
|
cat >> project.config << EOF
|
|
[label "Verified"]
|
|
function = MaxWithBlock
|
|
value = -2 Fails
|
|
value = -1 Doesn't seem to work
|
|
value = 0 No score
|
|
value = +1 Works for me
|
|
value = +2 Verified
|
|
[access "refs/heads/*"]
|
|
label-Verified = -2..+2 group CI-tools
|
|
EOF
|
|
git commit -a -m 'Update verify tags'
|
|
git push origin HEAD:refs/meta/config
|
|
|
|
popd
|
|
|
|
- name: Create temp dir for project creation
|
|
shell: mktemp -d
|
|
register: project_tmp
|
|
|
|
- name: Create project project in Gerrit
|
|
uri:
|
|
url: http://localhost:8081/a/projects/x%2Ftest-project
|
|
method: PUT
|
|
user: admin
|
|
password: secret
|
|
status_code: 201
|
|
|
|
- name: Create initial commit and change in test-project
|
|
shell:
|
|
executable: /bin/sh
|
|
chdir: "{{ project_tmp.stdout }}"
|
|
cmd: |
|
|
set -x
|
|
|
|
git init .
|
|
cat >.gitreview <<EOF
|
|
[gerrit]
|
|
host=localhost
|
|
port=29418
|
|
project=x/test-project
|
|
EOF
|
|
git add .gitreview
|
|
git commit -m "Initial commit"
|
|
git remote add gerrit http://admin:secret@localhost:8081/x/test-project
|
|
git push -f --set-upstream gerrit +HEAD:master
|
|
|
|
curl -Lo .git/hooks/commit-msg http://localhost:8081/tools/hooks/commit-msg
|
|
chmod u+x .git/hooks/commit-msg
|
|
|
|
cat >file-1.txt <<EOF
|
|
Hello, this is a file
|
|
EOF
|
|
git add file-1.txt
|
|
git commit -m "Sample review 1"
|
|
git push gerrit HEAD:refs/for/master
|
|
|
|
cat >file-2.txt <<EOF
|
|
Hello, this is a file
|
|
EOF
|
|
git add file-2.txt
|
|
git commit -m "Sample review 2"
|
|
git push gerrit HEAD:refs/for/master
|
|
|
|
cat >file-3.txt <<EOF
|
|
Hello, this is a file
|
|
EOF
|
|
git add file-3.txt
|
|
git commit -m "Sample review 3"
|
|
git push gerrit HEAD:refs/for/master
|
|
|
|
- name: Post a sample failure review
|
|
uri:
|
|
url: 'http://localhost:8081/a/changes/{{ item }}/revisions/1/review'
|
|
method: POST
|
|
user: zuul
|
|
password: secret
|
|
body_format: json
|
|
body:
|
|
tag: 'autogenerated:zuul:check'
|
|
labels:
|
|
'Verified': -2
|
|
message: |
|
|
Build failed (check pipeline). For information on how to proceed, see
|
|
https://docs.opendev.org/opendev/infra-manual/latest/developers.html#automated-testing
|
|
|
|
|
|
- test-success https://zuul.opendev.org/t/openstack/build/00b1ce7d5d994339a54dd8142a6813a6 : SUCCESS in {{ range(1, 59) | random }}m 22s
|
|
- test-failure https://zuul.opendev.org/t/openstack/build/00b1ce7d5d994339a54dd8142a6813a6 : FAILURE in {{ range(1, 59) | random }}m 22s
|
|
- test-retry-limit https://zuul.opendev.org/t/openstack/build/20efbb1b6983453884103e9628a70942 : RETRY_LIMIT in {{ range(1, 59) | random }}m 24s
|
|
- test-skipped https://zuul.opendev.org/t/openstack/build/43a00a7e190b4ce2b3e20a86166c011a : SKIPPED in {{ range(1, 59) | random }}m 40s
|
|
- test-aborted https://zuul.opendev.org/t/openstack/build/bc4a4559645b4088a9f8586c043c4764 : ABORTED in {{ range(1, 59) | random }}m 19s
|
|
- test-merger-failure https://zuul.opendev.org/t/openstack/build/cb469750b250430b95a02697931d6030 : MERGER_FAILURE in {{ range(1, 59) | random }}m 14s
|
|
- test-node-failure https://zuul.opendev.org/t/openstack/build/0897fafc9652480794652fc49e3c9baf : NODE_FAILURE in {{ range(1, 59) | random }}m 48s
|
|
- test-timed-out https://zuul.opendev.org/t/openstack/build/57c4eb5174554cbfbb06fd9118eb1ae4 : TIMED_OUT in {{ range(1, 59) | random }}m 37s (non-voting)
|
|
- test-post-failure https://zuul.opendev.org/t/openstack/build/30afb96669fe4f19b6215e79615ecd90 : POST_FAILURE in {{ range(1, 59) | random }}m 58s
|
|
- test-config-error https://zuul.opendev.org/t/openstack/build/76c69b8062284d959a85b55bc460f822 : CONFIG_ERROR in {{ range(1, 59) | random }}m 27s
|
|
- test-disk-full https://zuul.opendev.org/t/openstack/build/0897fafc9743580794652fc49e3c9baf : DISK_FULL in {{ range(1, 59) | random }}m 48s
|
|
loop:
|
|
- 1
|
|
- 2
|
|
- 3
|
|
|
|
- name: Post a sample good review in another pipeline
|
|
uri:
|
|
url: 'http://localhost:8081/a/changes/{{ item }}/revisions/1/review'
|
|
method: POST
|
|
user: zuul
|
|
password: secret
|
|
body_format: json
|
|
body:
|
|
tag: 'autogenerated:zuul:check-secondary'
|
|
message: |
|
|
Build succeeded (Secondary pipeline).
|
|
|
|
|
|
- test-second-ci https://zuul.opendev.org/t/openstack/build/f9c14856bf4e485089ca8fc8f00f324b : SUCCESS in {{ range(1, 59) | random}}m 11s
|
|
loop:
|
|
- 1
|
|
- 2
|
|
- 3
|
|
|
|
- name: Run selenium container
|
|
include_role:
|
|
name: run-selenium
|
|
|