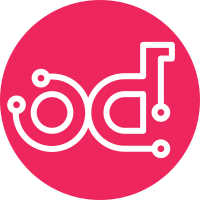
Previously the test was checking that stderr reported "Cloning into $PATH" which also happens in failure cases. We add an explicit check for a successful command return code to ensure that we aren't failing with that output. Change-Id: Iec51217f2cc97e6a56ff9d8b7a260650010f229f
79 lines
2.9 KiB
Python
79 lines
2.9 KiB
Python
# Copyright 2018 Red Hat, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from selenium import webdriver
|
|
from selenium.webdriver.support.ui import WebDriverWait
|
|
from selenium.common.exceptions import TimeoutException
|
|
import time
|
|
|
|
testinfra_hosts = [
|
|
'review01.openstack.org',
|
|
]
|
|
|
|
|
|
def test_gerrit_listening(host):
|
|
gerrit_web = host.socket("tcp://:::8081")
|
|
assert gerrit_web.is_listening
|
|
|
|
def test_gerrit_x_project_clone(host):
|
|
cmd = host.run(
|
|
'git clone http://localhost:8081/x/test-project /tmp/test-project')
|
|
assert "Cloning into '/tmp/test-project'..." in cmd.stderr
|
|
assert cmd.succeeded
|
|
|
|
def test_gerrit_screenshot(host):
|
|
driver = webdriver.Remote(
|
|
command_executor='http://%s:4444/wd/hub' % (host.backend.get_hostname()),
|
|
desired_capabilities=webdriver.DesiredCapabilities.FIREFOX)
|
|
|
|
try:
|
|
driver.get("http://localhost:8081")
|
|
WebDriverWait(driver, 30).until(lambda driver: driver.execute_script(
|
|
'return document.readyState') == 'complete')
|
|
# NOTE(ianw): The page doesn't really seem to be complete at
|
|
# this point, not sure what to listen for...
|
|
time.sleep(5)
|
|
driver.save_screenshot("/var/log/screenshots/gerrit-main-page.png")
|
|
|
|
for change in (1, 2):
|
|
driver.get("http://localhost:8081/c/x/test-project/+/%s" % change)
|
|
time.sleep(5)
|
|
driver.execute_script(
|
|
"document.querySelector('gr-app').shadowRoot"
|
|
".querySelector('gr-app-element').shadowRoot"
|
|
".querySelector('main')"
|
|
".querySelector('gr-change-view').shadowRoot"
|
|
".querySelector('paper-tab[data-name=\"change-view-tab-header-zuul-results-summary\"]')"
|
|
".click()"
|
|
)
|
|
time.sleep(5)
|
|
|
|
original_size = driver.get_window_size()
|
|
required_width = driver.execute_script(
|
|
'return document.body.parentNode.scrollWidth')
|
|
required_height = driver.execute_script(
|
|
'return document.body.parentNode.scrollHeight') + 100
|
|
driver.set_window_size(required_width, required_height)
|
|
|
|
driver.find_element_by_tag_name('body'). \
|
|
screenshot("/var/log/screenshots/gerrit-change-page-%s.png" % change)
|
|
|
|
driver.set_window_size(
|
|
original_size['width'], original_size['height'])
|
|
|
|
except TimeoutException as e:
|
|
raise e
|
|
finally:
|
|
driver.quit()
|