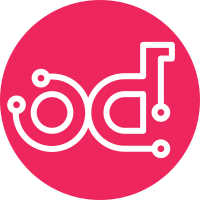
By default our mariadb database for gitea nodes limits itself to a maximum of 100 connections. We've seen errors like this: ...eb/routing/logger.go:102:func1() [I] router: completed POST /openstack/requirements/git-upload-pack for 127.0.0.1:50562, 500 Internal Server Error in 2.6ms @ context/user.go:17(web.gitHTTPRouters.UserAssignmentWeb) ...ules/context/repo.go:467:RepoAssignment() [E] GetUserByName: Error 1040: Too many connections And after reading gitea's source code this appears to be related to user lookups to determine if the user making a request against a repo owns the repo. To do this gitea does a db request to lookup the user from the request and when this hits the connection limit it bubbles up the mysql error 1040: Too may connections error. This problem seems infrequent so we double the limit to 200 which is both much larger but still a reasonable number. We also modify the test that checks for gitea server errors without an http 500 return code to avoid it matching this change improperly. This was happening because the commit message ends up in the rendered pages for system-config in the test gitea. Change-Id: If8c72ab277e88ae09a44a64a1571f94e43df23f8
151 lines
5.9 KiB
Python
151 lines
5.9 KiB
Python
# Copyright 2018 Red Hat, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from util import take_screenshots
|
|
|
|
testinfra_hosts = ['gitea99.opendev.org']
|
|
|
|
|
|
def test_gitea_listening(host):
|
|
gitea_https = host.socket("tcp://0.0.0.0:3000")
|
|
assert gitea_https.is_listening
|
|
gitea_http = host.socket("tcp://0.0.0.0:3080")
|
|
assert gitea_http.is_listening
|
|
gitea_ssh = host.socket("tcp://0.0.0.0:222")
|
|
assert gitea_ssh.is_listening
|
|
gitea_proxy = host.socket("tcp://0.0.0.0:3081")
|
|
assert gitea_proxy.is_listening
|
|
|
|
def test_ulimit(host):
|
|
cmd = host.run("docker exec gitea-docker_gitea-web_1 prlimit")
|
|
expected = ("STACK max stack size "
|
|
"16777216 9223372036854775807 bytes")
|
|
assert expected in cmd.stdout.split('\n')
|
|
|
|
def test_robots(host):
|
|
cmd = host.run('curl --insecure '
|
|
'--resolve gitea99.opendev.org:3081:127.0.0.1 '
|
|
'https://gitea99.opendev.org:3081/robots.txt')
|
|
assert 'Disallow: /' in cmd.stdout
|
|
assert 'This was kindly seeded with a mix of' in cmd.stdout
|
|
|
|
def test_matrix_server(host):
|
|
cmd = host.run('curl --insecure -v '
|
|
'--resolve gitea99.opendev.org:3081:127.0.0.1 '
|
|
'https://gitea99.opendev.org:3081/.well-known/matrix/server')
|
|
assert '"m.server": "opendev.ems.host:443"' in cmd.stdout
|
|
assert 'Access-Control-Allow-Origin' not in cmd.stderr
|
|
|
|
def test_matrix_client(host):
|
|
cmd = host.run('curl --insecure -v '
|
|
'--resolve gitea99.opendev.org:3081:127.0.0.1 '
|
|
'https://gitea99.opendev.org:3081/.well-known/matrix/client')
|
|
assert '"base_url": "https://opendev.ems.host"' in cmd.stdout
|
|
assert 'Access-Control-Allow-Origin' in cmd.stderr
|
|
|
|
def test_proxy(host):
|
|
cmd = host.run('curl --insecure '
|
|
'--resolve gitea99.opendev.org:3081:127.0.0.1 '
|
|
'https://gitea99.opendev.org:3081/')
|
|
assert 'Git with a cup of tea' in cmd.stdout
|
|
|
|
def test_proxy_ua_blacklist(host):
|
|
cmd = host.run('curl --insecure -A '
|
|
'" Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 5.1; TencentTraveler 4.0)" '
|
|
'--resolve gitea99.opendev.org:3081:127.0.0.1 '
|
|
'https://gitea99.opendev.org:3081/')
|
|
assert '403 Forbidden' in cmd.stdout
|
|
|
|
def test_ondisk_logs(host):
|
|
mariadb_log = host.file('/var/log/containers/docker-mariadb.log')
|
|
assert mariadb_log.exists
|
|
|
|
gitea_log = host.file('/var/log/containers/docker-gitea.log')
|
|
assert gitea_log.exists
|
|
|
|
gitea_ssh_log = host.file('/var/log/containers/docker-gitea-ssh.log')
|
|
assert gitea_ssh_log.exists
|
|
assert gitea_ssh_log.contains("Server listening on :: port 222.")
|
|
|
|
def test_project_clone(host):
|
|
# Note this tests the result of a project rename in gitea as well.
|
|
cmd = host.run(
|
|
'GIT_SSL_NO_VERIFY=1 '
|
|
'git clone https://localhost:3081/opendev/disk-image-builder '
|
|
'/tmp/disk-image-builder')
|
|
assert "Cloning into '/tmp/disk-image-builder'..." in cmd.stderr
|
|
assert cmd.succeeded
|
|
# Check that our default of master is still honored.
|
|
# Gitea defaults to main as of 1.17.0.
|
|
cmd = host.run(
|
|
'git -C /tmp/disk-image-builder '
|
|
'symbolic-ref refs/remotes/origin/HEAD')
|
|
assert "refs/remotes/origin/master" in cmd.stdout
|
|
assert "refs/remotes/origin/main" not in cmd.stdout
|
|
assert cmd.succeeded
|
|
|
|
def test_partial_project_clone(host):
|
|
cmd = host.run(
|
|
'GIT_SSL_NO_VERIFY=1 '
|
|
'git clone --filter=blob:none '
|
|
'https://localhost:3081/opendev/system-config '
|
|
'/tmp/test-system-config-clone')
|
|
assert "Cloning into '/tmp/test-system-config-clone'..." in cmd.stderr
|
|
assert cmd.succeeded
|
|
# Check that our default of master is still honored.
|
|
# Gitea defaults to main as of 1.17.0.
|
|
cmd = host.run(
|
|
'git -C /tmp/test-system-config-clone '
|
|
'symbolic-ref refs/remotes/origin/HEAD')
|
|
assert "refs/remotes/origin/master" in cmd.stdout
|
|
assert "refs/remotes/origin/main" not in cmd.stdout
|
|
assert cmd.succeeded
|
|
|
|
def test_no_500_template_content(host):
|
|
# We discovered that gitea template rendering errors produce 500 errors
|
|
# in the rendered template but not in our http response codes. Check a
|
|
# number of pages for 500 errors in the html response.
|
|
paths_to_check = [
|
|
'/',
|
|
'/opendev/system-config',
|
|
'/opendev/system-config/src/branch/master/playbooks/roles/gitea/tasks/main.yaml',
|
|
'/explore/repos',
|
|
'/explore/users',
|
|
'/explore/organizations',
|
|
'/explore/code?q=gitea&t=',
|
|
]
|
|
for path in paths_to_check:
|
|
cmd = host.run('curl --insecure '
|
|
'--resolve gitea99.opendev.org:3081:127.0.0.1 '
|
|
'https://gitea99.opendev.org:3081' + path)
|
|
assert 'status-page-500' not in cmd.stdout
|
|
assert '<title>Internal Server Error' not in cmd.stdout
|
|
|
|
def test_gitea_screenshots(host):
|
|
|
|
shots = (
|
|
('https://localhost:3081', None, 'gitea-main.png'),
|
|
('https://localhost:3081/opendev/system-config', None,
|
|
'gitea-project-system-config.png'),
|
|
('https://localhost:3081/opendev/disk-image-builder', None,
|
|
'gitea-project-dib.png'),
|
|
('https://localhost:3081/opendev/', None,
|
|
'gitea-org-opendev.png'),
|
|
('https://localhost:3081/explore/organizations', None,
|
|
'gitea-org-explore.png'),
|
|
)
|
|
|
|
take_screenshots(host, shots)
|
|
|