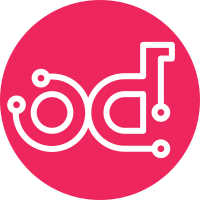
Remove or disable the not used features of commons, like wiki page and qa. Inherited commons profile is dropped due several missing features like inherited library handling and installation task execution order. Change-Id: Iaa2abf00edb3e5d854ffc51ff6aa6c22419145da
113 lines
3.6 KiB
Plaintext
113 lines
3.6 KiB
Plaintext
<?php
|
|
|
|
/**
|
|
* @file
|
|
* Install, update and uninstall functions for the Groups install profile.
|
|
*/
|
|
|
|
/**
|
|
* Implements hook_requirements().
|
|
* Set minimum 'max_execution_time' requirement
|
|
*/
|
|
function groups_requirements($phase) {
|
|
$requirements = array();
|
|
// Ensure translations don't break during installation.
|
|
$t = get_t();
|
|
if($phase == 'install') {
|
|
// Test PHP minimum execution time
|
|
$requirements['php_max_execution_time'] = array(
|
|
'title' => $t('PHP Max Execution Time'),
|
|
'value' => ini_get('max_execution_time'),
|
|
);
|
|
$max_execution_time = (int)ini_get('max_execution_time');
|
|
// Don't set the error when max_execution_time is 0 (Infinite).
|
|
if ($max_execution_time && $max_execution_time < DRUPAL_MINIMUM_MAX_EXECUTION_TIME) {
|
|
$requirements['php_max_execution_time']['description'] = $t('Your PHP execution time is too low, please set it greater than or equal to %time seconds.', array('%time' => DRUPAL_MINIMUM_MAX_EXECUTION_TIME));
|
|
$requirements['php_max_execution_time']['severity'] = REQUIREMENT_ERROR;
|
|
}
|
|
}
|
|
return $requirements;
|
|
}
|
|
|
|
/**
|
|
* Implements hook_install().
|
|
*
|
|
* Perform actions to set up the site for this profile.
|
|
*
|
|
* @see system_install()
|
|
*/
|
|
function groups_install() {
|
|
// Enable the Origins theme and set it as the default.
|
|
theme_enable(array('adaptivetheme', 'commons_origins', 'adaptivetheme_admin'));
|
|
|
|
// The Bartik theme is automatically enabled during installation. Disable it.
|
|
db_update('system')
|
|
->fields(array('status' => 0))
|
|
->condition('type', 'theme')
|
|
->condition('name', 'bartik')
|
|
->execute();
|
|
|
|
// Set the default and administration themes.
|
|
variable_set('theme_default', 'commons_origins');
|
|
// Set a default administrative theme:
|
|
variable_set('admin_theme', 'adaptivetheme_admin');
|
|
variable_set('node_admin_theme', FALSE);
|
|
|
|
// Do not use the administration theme when editing or creating content.
|
|
variable_set('node_admin_theme', '0');
|
|
|
|
// Set a default user avatar.
|
|
/*commons_set_default_avatar();*/
|
|
|
|
// Place site blocks in the menu_bar and header regions.
|
|
$menu_block = array(
|
|
'module' => 'system',
|
|
'delta' => 'main-menu',
|
|
'theme' => 'commons_origins',
|
|
'visibility' => 0,
|
|
'region' => 'menu_bar',
|
|
'status' => 1,
|
|
'pages' => '',
|
|
'title' => '<none>',
|
|
);
|
|
drupal_write_record('block', $menu_block);
|
|
|
|
$search_block = array(
|
|
'module' => 'search',
|
|
'delta' => 'form',
|
|
'theme' => 'commons_origins',
|
|
'visibility' => 0,
|
|
'region' => 'header',
|
|
'status' => 1,
|
|
'pages' => '',
|
|
'weight' => 2,
|
|
'title' => '<none>',
|
|
);
|
|
drupal_write_record('block', $search_block);
|
|
|
|
// Create a default role for site administrators, with all available permissions assigned.
|
|
$admin_role = new stdClass();
|
|
$admin_role->name = 'administrator';
|
|
$admin_role->weight = 2;
|
|
user_role_save($admin_role);
|
|
// Set this as the administrator role.
|
|
variable_set('user_admin_role', $admin_role->rid);
|
|
|
|
// Assign user 1 the "administrator" role.
|
|
db_insert('users_roles')
|
|
->fields(array('uid' => 1, 'rid' => $admin_role->rid))
|
|
->execute();
|
|
|
|
// AdaptiveTheme requires that the system theme settings form
|
|
// be submitted in order for its themes' settings to be properly set
|
|
// and the resulting css files generated.
|
|
// For more background, see http://drupal.org/node/1776730.
|
|
module_load_include('inc', 'system', 'system.admin');
|
|
foreach (array('adaptivetheme', 'commons_origins') as $theme_name) {
|
|
$form_state = form_state_defaults();
|
|
$form_state['build_info']['args'][0] = $theme_name;
|
|
$form_state['values'] = array();
|
|
drupal_form_submit('system_theme_settings', $form_state);
|
|
}
|
|
}
|