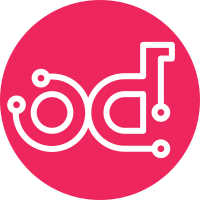
Get all track questions GET /api/v1/track-question-templates params 'page' => 'integer|min:1' 'per_page' => 'required_with:page|integer|min:5|max:100' filter 'name' => ['=@', '=='] 'label' => ['=@', '=='] 'class_name' => ['=='] order * id * name * label expand * tracks scopes %s/summits/read/all Add track question POST /api/v1/track-question-templates payload 'name' => 'sometimes|alpha_dash|max:255' 'label' => 'sometimes|string' 'is_mandatory' => 'sometimes|boolean' 'is_read_only' => 'sometimes|boolean' 'tracks' => 'sometimes|int_array' for TrackCheckBoxQuestionTemplate and TrackTextBoxQuestionTemplate 'initial_value' => 'string|sometimes' for TrackDropDownQuestionTemplate 'is_multiselect' => 'sometimes|boolean' 'is_country_selector' => 'sometimes|boolean' scopes %s/summits/write %s/track-question-templates/write PUT /api/v1/track-question-templates/{track_question_template_id} payload same as POST scopes %s/summits/write %s/track-question-templates/write delete track question DELETE /api/v1/track-question-templates/{track_question_template_id} scopes %s/summits/write %s/track-question-templates/write get track question metadata GET /api/v1/track-question-templates/metadata scopes %s/summits/read/all add track question value POST /api/v1/track-question-templates/{track_question_template_id}/values payload 'value' => 'required|string|max:255' 'label' => 'required|string' scopes %s/summits/write %s/track-question-templates/write update track question value /api/v1/track-question-templates/{track_question_template_id}/values/{track_question_template_value_id} payload 'value' => 'sometimes|string|max:255' 'label' => 'sometimes|string' 'order' => 'sometimes|integer|min:1' delete track question value DELETE /api/v1/track-question-templates/{track_question_template_id}/values/{track_question_template_value_id} scopes %s/summits/write %s/track-question-templates/write get track question template value GET /api/v1/track-question-templates/{track_question_template_id}/values/{track_question_template_value_id} scopes '%s/summits/read/all' Change-Id: I663bccf3987cb0b7e337e0fe5b92f3723fac5cd6
38 lines
1.2 KiB
PHP
38 lines
1.2 KiB
PHP
<?php namespace App\Models\Foundation\Summit\Repositories;
|
|
/**
|
|
* Copyright 2018 OpenStack Foundation
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
**/
|
|
use App\Models\Foundation\Summit\Events\Presentations\TrackQuestions\TrackQuestionTemplate;
|
|
use models\utils\IBaseRepository;
|
|
/**
|
|
* Interface ITrackQuestionTemplateRepository
|
|
* @package App\Models\Foundation\Summit\Repositories
|
|
*/
|
|
interface ITrackQuestionTemplateRepository extends IBaseRepository
|
|
{
|
|
/**
|
|
* @param string $name
|
|
* @return TrackQuestionTemplate
|
|
*/
|
|
public function getByName($name);
|
|
|
|
/**
|
|
* @param string $label
|
|
* @return TrackQuestionTemplate
|
|
*/
|
|
public function getByLabel($label);
|
|
|
|
/**
|
|
* @return array
|
|
*/
|
|
public function getQuestionsMetadata();
|
|
} |