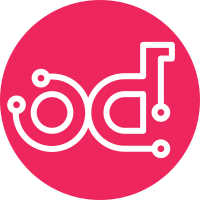
PUT /api/v1/summits/{id}/locations/venues/{venue_id}/floors/{floor_id} Payload * name (sometimes|string|max:50) * number (sometimes|integer) * description (sometimes|string) Change-Id: I575680d5f32814393eeac655d55227dd1cae349a
75 lines
1.6 KiB
PHP
75 lines
1.6 KiB
PHP
<?php namespace App\Events;
|
|
/**
|
|
* Copyright 2018 OpenStack Foundation
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
**/
|
|
use Illuminate\Queue\SerializesModels;
|
|
/**
|
|
* Class SummitEventTypeAction
|
|
* @package App\Events
|
|
*/
|
|
class SummitEventTypeAction
|
|
{
|
|
use SerializesModels;
|
|
|
|
/**
|
|
* @var int
|
|
*/
|
|
private $event_type_id;
|
|
|
|
/**
|
|
* @var string
|
|
*/
|
|
private $class_name;
|
|
|
|
/**
|
|
* @var int
|
|
*/
|
|
private $summit_id;
|
|
|
|
/**
|
|
* SummitEventTypeAction constructor.
|
|
* @param int $event_type_id
|
|
* @param string $class_name
|
|
* @param int $summit_id
|
|
*/
|
|
public function __construct($event_type_id, $class_name, $summit_id)
|
|
{
|
|
$this->event_type_id = $event_type_id;
|
|
$this->class_name = $class_name;
|
|
$this->summit_id = $summit_id;
|
|
}
|
|
|
|
/**
|
|
* @return int
|
|
*/
|
|
public function getEventTypeId()
|
|
{
|
|
return $this->event_type_id;
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function getClassName()
|
|
{
|
|
return $this->class_name;
|
|
}
|
|
|
|
/**
|
|
* @return int
|
|
*/
|
|
public function getSummitId()
|
|
{
|
|
return $this->summit_id;
|
|
}
|
|
|
|
} |