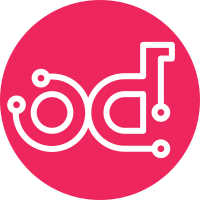
Add bookable room to summit POST /api/v1/summits/{id}/locations/venues/{venue_id}/bookable-rooms payload time_slot_cost: required|numeric currency:required|iso_currency_code(USD,EUR) capacity:required|integer floor_id:sometimes|integer name:sometimes|string|max:255 description:sometimes|string order:sometimes|integer|min:1 update bookable room per summit PUT /api/v1/summits/{id}/locations/venues/{venue_id}/bookable-rooms/{room_id} payload time_slot_cost: required|numeric currency:required|iso_currency_code(USD,EUR) capacity:required|integer floor_id:sometimes|integer name:sometimes|string|max:255 description:sometimes|string order:sometimes|integer|min:1 delete bookable room DELETE /api/v1/summits/{id}/locations/venues/{venue_id}/bookable-rooms/{room_id} add attribute value to bookable room PUT /api/v1/summits/{id}/locations/venues/{venue_id}/bookable-rooms/{room_id}/attributes/{attribute_id} delete attribute value from bookable room DELETE /api/v1/summits/{id}/locations/venues/{venue_id}/bookable-rooms/{room_id}/attributes/{attribute_id} Added missing endpoints to CRUD bookable rooms attributes per summit Get all bookable rooms attribute types GET /api/v1/summits/{id}/bookable-room-attribute-types Get bookable room attribute type by id GET /api/v1/summits/{id}/bookable-room-attribute-types/{type_id} Add bookable room attribute type POST /api/v1/summits/{id}/bookable-room-attribute-types payload type [required|string] Update bookable room attribute type PUT /api/v1/summits/{id}/bookable-room-attribute-types/{type_id} payload type [required|string] delete attribute type by id DELETE /api/v1/summits/{id}/bookable-room-attribute-types/{type_id} get all attribute values by attribute type GET /api/v1/summits/{id}/bookable-room-attribute-types/{type_id}/values add attribute value to attribute type POST /api/v1/summits/{id}/bookable-room-attribute-types/{type_id}/values payload value [required|string] update attribute value PUT /api/v1/summits/{id}/bookable-room-attribute-types/{type_id}/values/{value_id} payload value [required|string] delete attribute value by id DELETE /api/v1/summits/{id}/bookable-room-attribute-types/{type_id}/values/{value_id} refund endpoint (admin only) DELETE /api/v1/summits/{id}/bookable-rooms/{room_id}/reservations/{reservation_id}/refund payload amount [numeric|required] get all reservations by summit (admin) GET /api/v1/summits/{id}/locations/bookable-rooms/all/reservations query params page:integer|min:1 per_page:required_with:page|integer|min:1|max:100 filter: allowed filters summit_id: ['=='] room_name: ['==', '=@'] room_id: ['=='] owner_id: ['=='] status: ['=='] start_datetime: ['>', '<', '<=', '>=', '=='] end_datetime: ['>', '<', '<=', '>=', '=='] order allowed ordering id,start_datetime,end_datetime Change-Id: Ic7f6ed7defaf20b8799a7d94beb0a82103b206f0
117 lines
3.4 KiB
PHP
117 lines
3.4 KiB
PHP
<?php namespace Tests;
|
|
/**
|
|
* Copyright 2019 OpenStack Foundation
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
**/
|
|
|
|
/**
|
|
* Class OAuth2BookableRoomAttributeTypesApiTest
|
|
* @package Tests
|
|
*/
|
|
final class OAuth2BookableRoomAttributeTypesApiTest extends \ProtectedApiTest
|
|
{
|
|
public function testGetBookableAttributeTypesBySummit($summit_id = 27){
|
|
$params = [
|
|
'id' => $summit_id,
|
|
'page' => 1 ,
|
|
'per_page' => 10,
|
|
'expand' => 'values'
|
|
];
|
|
|
|
$headers = array("HTTP_Authorization" => " Bearer " .$this->access_token);
|
|
$response = $this->action(
|
|
"GET",
|
|
"OAuth2SummitBookableRoomsAttributeTypeApiController@getAllBookableRoomAttributeTypes",
|
|
$params,
|
|
[],
|
|
[],
|
|
[],
|
|
$headers
|
|
);
|
|
|
|
$content = $response->getContent();
|
|
$attributes = json_decode($content);
|
|
|
|
$this->assertResponseStatus(200);
|
|
$this->assertTrue(count($attributes->data) > 0);
|
|
}
|
|
|
|
|
|
public function testAddAttributeType($summit_id = 27){
|
|
$params = [
|
|
'id' => $summit_id,
|
|
];
|
|
|
|
$type = str_random(16).'_attribute_type';
|
|
|
|
$data = [
|
|
'type' => $type
|
|
];
|
|
|
|
$headers = [
|
|
"HTTP_Authorization" => " Bearer " . $this->access_token,
|
|
"CONTENT_TYPE" => "application/json"
|
|
];
|
|
|
|
$response = $this->action(
|
|
"POST",
|
|
"OAuth2SummitBookableRoomsAttributeTypeApiController@addBookableRoomAttributeType",
|
|
$params,
|
|
[],
|
|
[],
|
|
[],
|
|
$headers,
|
|
json_encode($data)
|
|
);
|
|
|
|
$content = $response->getContent();
|
|
$this->assertResponseStatus(201);
|
|
$attribute_type = json_decode($content);
|
|
$this->assertTrue(!is_null($attribute_type));
|
|
return $attribute_type;
|
|
}
|
|
|
|
public function testAddAttributeValue($summit_id = 27){
|
|
$type = $this->testAddAttributeType($summit_id);
|
|
$params = [
|
|
'id' => $summit_id,
|
|
'type_id' => $type->id,
|
|
];
|
|
|
|
$val = str_random(16).'_attribute_value';
|
|
|
|
$data = [
|
|
'value' => $val
|
|
];
|
|
|
|
$headers = [
|
|
"HTTP_Authorization" => " Bearer " . $this->access_token,
|
|
"CONTENT_TYPE" => "application/json"
|
|
];
|
|
|
|
$response = $this->action(
|
|
"POST",
|
|
"OAuth2SummitBookableRoomsAttributeTypeApiController@addBookableRoomAttributeValue",
|
|
$params,
|
|
[],
|
|
[],
|
|
[],
|
|
$headers,
|
|
json_encode($data)
|
|
);
|
|
|
|
$content = $response->getContent();
|
|
$this->assertResponseStatus(201);
|
|
$attribute_value = json_decode($content);
|
|
$this->assertTrue(!is_null($attribute_value));
|
|
return $attribute_value;
|
|
}
|
|
} |