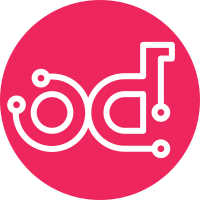
With "extends_documentation_fragment: ['openstack.cloud.openstack']" it is not necessary to list required Python libraries in section 'requirements' of DOCUMENTATION docstring in modules. Ansible will merge requirements from doc fragments and DOCUMENTATION docstring which previously resulted in duplicates such as in server module [0]: * openstacksdk * openstacksdk >= 0.36, < 0.99.0 * python >= 3.6 When removing the 'requirements' section from server module, then Ansible will list openstacksdk once only: * openstacksdk >= 0.36, < 0.99.0 * python >= 3.6 To see what documentation Ansible will produce for server module run: ansible-doc --type module openstack.cloud.server [0] https://docs.ansible.com/ansible/latest/collections/openstack/\ cloud/server_module.html Change-Id: I727ed95ee480bb644b5a533f6a9526973677064c
109 lines
2.7 KiB
Python
109 lines
2.7 KiB
Python
#!/usr/bin/python
|
|
# -*- coding: utf-8 -*-
|
|
|
|
# Copyright (c) 2016 Hewlett-Packard Enterprise Corporation
|
|
# GNU General Public License v3.0+ (see COPYING or https://www.gnu.org/licenses/gpl-3.0.txt)
|
|
|
|
DOCUMENTATION = r'''
|
|
---
|
|
module: compute_service_info
|
|
short_description: Fetch OpenStack Compute (Nova) services
|
|
author: OpenStack Ansible SIG
|
|
description:
|
|
- Fetch OpenStack Compute (Nova) services.
|
|
options:
|
|
binary:
|
|
description:
|
|
- Filter the service list result by binary name of the service.
|
|
type: str
|
|
host:
|
|
description:
|
|
- Filter the service list result by the host name.
|
|
type: str
|
|
extends_documentation_fragment:
|
|
- openstack.cloud.openstack
|
|
'''
|
|
|
|
EXAMPLES = r'''
|
|
- name: Fetch all OpenStack Compute (Nova) services
|
|
openstack.cloud.compute_service_info:
|
|
cloud: awesomecloud
|
|
|
|
- name: Fetch a subset of OpenStack Compute (Nova) services
|
|
openstack.cloud.compute_service_info:
|
|
cloud: awesomecloud
|
|
binary: "nova-compute"
|
|
host: "localhost"
|
|
'''
|
|
|
|
RETURN = r'''
|
|
compute_services:
|
|
description: List of dictionaries describing Compute (Nova) services.
|
|
returned: always
|
|
type: list
|
|
elements: dict
|
|
contains:
|
|
availability_zone:
|
|
description: The availability zone name.
|
|
type: str
|
|
binary:
|
|
description: The binary name of the service.
|
|
type: str
|
|
disabled_reason:
|
|
description: The reason why the service is disabled
|
|
type: str
|
|
id:
|
|
description: Unique UUID.
|
|
type: str
|
|
is_forced_down:
|
|
description: If the service has been forced down or nova-compute
|
|
type: bool
|
|
host:
|
|
description: The name of the host.
|
|
type: str
|
|
name:
|
|
description: Service name
|
|
type: str
|
|
state:
|
|
description: The state of the service. One of up or down.
|
|
type: str
|
|
status:
|
|
description: The status of the service. One of enabled or disabled.
|
|
type: str
|
|
update_at:
|
|
description: The date and time when the resource was updated
|
|
type: str
|
|
'''
|
|
|
|
from ansible_collections.openstack.cloud.plugins.module_utils.openstack import OpenStackModule
|
|
|
|
|
|
class ComputeServiceInfoModule(OpenStackModule):
|
|
argument_spec = dict(
|
|
binary=dict(),
|
|
host=dict(),
|
|
)
|
|
|
|
module_kwargs = dict(
|
|
supports_check_mode=True
|
|
)
|
|
|
|
def run(self):
|
|
kwargs = {k: self.params[k]
|
|
for k in ['binary', 'host']
|
|
if self.params[k] is not None}
|
|
compute_services = self.conn.compute.services(**kwargs)
|
|
|
|
self.exit_json(changed=False,
|
|
compute_services=[s.to_dict(computed=False)
|
|
for s in compute_services])
|
|
|
|
|
|
def main():
|
|
module = ComputeServiceInfoModule()
|
|
module()
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main()
|