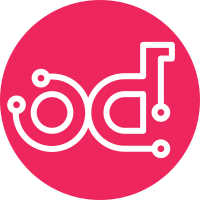
When accessing nested facts, such as in ansible_local sections, guard statements in the when clause can get quite long, due to having to repeate the 'in/not in' logic for every key at every level. The get_nested filter attempts to instead to move the guard into a single line, making the conditions easier to write and maintain. As example, ('openstack_ansible' not in ansible_local or 'swift' not in ansible_local['openstack_ansible'] or 'venv_tag' not in ansible_local['openstack_ansible']['swift'] or ansible_local['openstack_ansible']['swift']['venv_tag'] == swift_venv_tag) could be rewritten as get_nested(ansible_local, 'openstack_ansible.swift.venv_tag') == swift_venv_tag Change-Id: I3b43c25c8783c43cf5285f2b3e7267b2c5712ea0
138 lines
4.6 KiB
YAML
138 lines
4.6 KiB
YAML
---
|
|
# Copyright 2016, @WalmartLabs
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
|
|
|
|
- name: Test filters
|
|
hosts: localhost
|
|
connection: local
|
|
gather_facts: no
|
|
tasks:
|
|
- name: Validate bit_length_power_of_2 filter
|
|
assert:
|
|
that:
|
|
- "{{ 1024 | bit_length_power_of_2 }} == 1024"
|
|
- "{{ 9600 | bit_length_power_of_2 }} == 16384"
|
|
|
|
- name: Set net filter facts
|
|
set_fact:
|
|
url_netloc: "{{ 'http://review.openstack.org:29418/something' | netloc }}"
|
|
url_netloc_no_port: "{{ 'http://review.openstack.org:29418/something' | netloc_no_port }}"
|
|
url_netorigin: "{{ 'http://review.openstack.org:29418/something' | netorigin }}"
|
|
- name: Validate net filters
|
|
assert:
|
|
that:
|
|
- "url_netloc == 'review.openstack.org:29418'"
|
|
- "url_netloc_no_port == 'review.openstack.org'"
|
|
- "url_netorigin == 'http://review.openstack.org:29418'"
|
|
|
|
- name: Validate string_2_int filter
|
|
assert:
|
|
that:
|
|
- "{{ 'test' | string_2_int }} == 3752"
|
|
|
|
- name: Set pip package list facts
|
|
set_fact:
|
|
pip_package_list_1:
|
|
- pip==8.1.2
|
|
- setuptools==25.1.0
|
|
- wheel==0.29.0
|
|
pip_package_list_1_names:
|
|
- pip
|
|
- setuptools
|
|
- wheel
|
|
pip_package_list_2:
|
|
- babel==2.3.4
|
|
- pip==8.1.0
|
|
pip_package_list_merged:
|
|
- babel==2.3.4
|
|
- pip==8.1.0
|
|
- setuptools==25.1.0
|
|
- wheel==0.29.0
|
|
- name: Set pip package filter facts
|
|
set_fact:
|
|
pip_package_list_1_names_filtered: "{{ pip_package_list_1 | pip_requirement_names }}"
|
|
pip_package_list_constraint_filtered: "{{ pip_package_list_1 | pip_constraint_update(pip_package_list_2) }}"
|
|
- name: Validate pip requirement filters
|
|
assert:
|
|
that:
|
|
- "pip_package_list_1_names_filtered == pip_package_list_1_names"
|
|
- "pip_package_list_constraint_filtered == pip_package_list_merged"
|
|
|
|
- name: Set splitlines string facts
|
|
set_fact:
|
|
string_with_lines: |
|
|
this
|
|
is
|
|
a
|
|
test
|
|
string_split_lines:
|
|
- this
|
|
- is
|
|
- a
|
|
- test
|
|
- name: Set splitlines filter fact
|
|
set_fact:
|
|
string_split_lines_filtered: "{{ string_with_lines | splitlines }}"
|
|
- name: Validate splitlines filter
|
|
assert:
|
|
that: "string_split_lines_filtered == string_split_lines"
|
|
|
|
- name: Set git repo facts
|
|
set_fact:
|
|
git_repo: "git+https://git.openstack.org/openstack/nova@2bc8128d7793cc72ca2e146de3a092e1fef5033b#egg=nova&gitname=nova"
|
|
git_repo_name: nova
|
|
git_repo_link_parts:
|
|
name: nova
|
|
version: 2bc8128d7793cc72ca2e146de3a092e1fef5033b
|
|
plugin_path: null
|
|
url: "https://git.openstack.org/openstack/nova"
|
|
original: "git+https://git.openstack.org/openstack/nova@2bc8128d7793cc72ca2e146de3a092e1fef5033b#egg=nova&gitname=nova"
|
|
- name: Set git link parse filter facts
|
|
set_fact:
|
|
git_repo_link_parse_filtered: "{{ git_repo | git_link_parse }}"
|
|
git_repo_link_parse_name_filtered: "{{ git_repo | git_link_parse_name }}"
|
|
- name: Validate git link parse filters
|
|
assert:
|
|
that:
|
|
- "git_repo_link_parse_filtered == git_repo_link_parts"
|
|
- "git_repo_link_parse_name_filtered == git_repo_name"
|
|
|
|
- name: Set deprecation variable facts
|
|
set_fact:
|
|
new_var: new
|
|
old_var: old
|
|
- name: Set deprecated filter fact
|
|
set_fact:
|
|
deprecated_value: "{{ new_var | deprecated(old_var, 'old_var', 'new_var', 'Next Release', false) }}"
|
|
- name: Validate deprecated filter
|
|
assert:
|
|
that: "deprecated_value == old_var"
|
|
|
|
- name: Set test_dict fact
|
|
set_fact:
|
|
test_dict:
|
|
a:
|
|
b:
|
|
c: d
|
|
|
|
- name: Validate get_nested returns value
|
|
assert:
|
|
that: "{{ test_dict|get_nested('a.b.c') == 'd' }}"
|
|
|
|
- name: Validate get_nested returns None on missing key
|
|
assert:
|
|
that: "{{ test_dict|get_nested('a.c') == None }}"
|