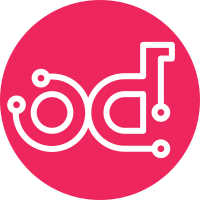
Misc updates as a result of testing with latest OpenStack Mitaka RDO packages from IBM: - neutron.conf: disable neutron_rootwrap_daemon - non-functional. - nova.conf: set lock_path to /var/lib/nova/tmp inline with rpm packaging. - run proxy install process during config-changed, ensuring that any new compute hosts get installed and configured. - enable and start services on install, as rpm packages install disabled. - refactor use of proxy in hooks module to ensure its not created on module load, but as an when required. - change behaviour of remote-key to write key to secured local file, avoiding the need to run this charm from a local copy with the key embedded. README updated for any behavioural changes in configuration. Change-Id: I53d7331a2ddcf73bc41bc8d73be5bf165bf55a92
118 lines
2.9 KiB
Python
Executable File
118 lines
2.9 KiB
Python
Executable File
#!/usr/bin/python
|
|
# Copyright 2016 Canonical Ltd
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
import sys
|
|
|
|
from charmhelpers.core.hookenv import (
|
|
Hooks,
|
|
config,
|
|
log,
|
|
relation_ids,
|
|
relation_set,
|
|
UnregisteredHookError,
|
|
)
|
|
|
|
from charmhelpers.fetch import (
|
|
apt_install,
|
|
)
|
|
|
|
from nova_compute_utils import (
|
|
restart_map,
|
|
register_configs,
|
|
assess_status,
|
|
)
|
|
from nova_compute_proxy import (
|
|
REMOTEProxy,
|
|
restart_on_change,
|
|
)
|
|
|
|
hooks = Hooks()
|
|
CONFIGS = register_configs()
|
|
|
|
|
|
def get_proxy():
|
|
return REMOTEProxy(user=config('remote-user'),
|
|
ssh_key=config('remote-key'),
|
|
hosts=config('remote-hosts'),
|
|
repository=config('remote-repos'),
|
|
password=config('remote-password'))
|
|
|
|
|
|
@hooks.hook('install.real')
|
|
def install():
|
|
apt_install(['fabric'], fatal=True)
|
|
|
|
|
|
@hooks.hook('config-changed')
|
|
def config_changed():
|
|
proxy = get_proxy()
|
|
proxy.install()
|
|
proxy.configure()
|
|
if config('instances-path') is not None:
|
|
proxy.fix_path_ownership(config('instances-path'), user='nova')
|
|
|
|
@restart_on_change(restart_map(), proxy.restart_service)
|
|
def write_config():
|
|
CONFIGS.write_all()
|
|
write_config()
|
|
|
|
proxy.commit()
|
|
|
|
|
|
@hooks.hook('amqp-relation-joined')
|
|
def amqp_joined(relation_id=None):
|
|
relation_set(relation_id=relation_id,
|
|
username=config('rabbit-user'),
|
|
vhost=config('rabbit-vhost'))
|
|
|
|
|
|
@hooks.hook('amqp-relation-broken',
|
|
'image-service-relation-broken',
|
|
'neutron-plugin-api-relation-broken',
|
|
'nova-ceilometer-relation-changed',
|
|
'cloud-compute-relation-changed',
|
|
'neutron-plugin-api-relation-changed',
|
|
'image-service-relation-changed',
|
|
'amqp-relation-changed',
|
|
'amqp-relation-departed')
|
|
def relation_broken():
|
|
proxy = get_proxy()
|
|
|
|
@restart_on_change(restart_map(), proxy.restart_service)
|
|
def write_config():
|
|
CONFIGS.write_all()
|
|
write_config()
|
|
proxy.commit()
|
|
|
|
|
|
@hooks.hook('upgrade-charm')
|
|
def upgrade_charm():
|
|
for r_id in relation_ids('amqp'):
|
|
amqp_joined(relation_id=r_id)
|
|
|
|
|
|
@hooks.hook('update-status')
|
|
def update_status():
|
|
log('Updating status.')
|
|
assess_status(CONFIGS)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
try:
|
|
hooks.execute(sys.argv)
|
|
except UnregisteredHookError as e:
|
|
log('Unknown hook {} - skipping.'.format(e))
|
|
assess_status(CONFIGS)
|