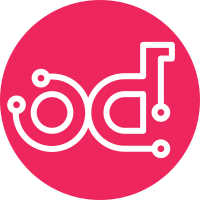
The __future__ module [1] was used in this context to ensure compatibility between python 2 and python 3. We previously dropped the support of python 2.7 [2] and now we only support python 3 so we don't need to continue to use this module and the imports listed below. Imports commonly used and their related PEPs: - `division` is related to PEP 238 [3] - `print_function` is related to PEP 3105 [4] - `unicode_literals` is related to PEP 3112 [5] - `with_statement` is related to PEP 343 [6] - `absolute_import` is related to PEP 328 [7] [1] https://docs.python.org/3/library/__future__.html [2] https://governance.openstack.org/tc/goals/selected/ussuri/drop-py27.html [3] https://www.python.org/dev/peps/pep-0238 [4] https://www.python.org/dev/peps/pep-3105 [5] https://www.python.org/dev/peps/pep-3112 [6] https://www.python.org/dev/peps/pep-0343 [7] https://www.python.org/dev/peps/pep-0328 Change-Id: Ib1ed5b598a52375e29e247db9ab4786df5b6d142
80 lines
2.3 KiB
Python
80 lines
2.3 KiB
Python
# -*- coding: utf-8 -*-
|
|
|
|
import sys
|
|
from setuptools import setup, find_packages
|
|
from setuptools.command.test import test as TestCommand
|
|
|
|
version = "0.0.1.dev1"
|
|
install_require = [
|
|
]
|
|
|
|
tests_require = [
|
|
'tox >= 2.3.1',
|
|
]
|
|
|
|
class Tox(TestCommand):
|
|
user_options = [('tox-args=', 'a', "Arguments to pass to tox")]
|
|
def initialize_options(self):
|
|
TestCommand.initialize_options(self)
|
|
self.tox_args = None
|
|
def finalize_options(self):
|
|
TestCommand.finalize_options(self)
|
|
self.test_args = []
|
|
self.test_suite = True
|
|
def run_tests(self):
|
|
#import here, cause outside the eggs aren't loaded
|
|
import tox
|
|
import shlex
|
|
args = self.tox_args
|
|
# remove the 'test' arg from argv as tox passes it to ostestr which
|
|
# breaks it.
|
|
sys.argv.pop()
|
|
if args:
|
|
args = shlex.split(self.tox_args)
|
|
errno = tox.cmdline(args=args)
|
|
sys.exit(errno)
|
|
|
|
|
|
if sys.argv[-1] == 'publish':
|
|
os.system("python setup.py sdist upload")
|
|
os.system("python setup.py bdist_wheel upload")
|
|
sys.exit()
|
|
|
|
|
|
if sys.argv[-1] == 'tag':
|
|
os.system("git tag -a %s -m 'version %s'" % (version, version))
|
|
os.system("git push --tags")
|
|
sys.exit()
|
|
|
|
|
|
setup(
|
|
name='charms.openstack',
|
|
version=version,
|
|
description='Provide base module for layer-openstack.',
|
|
classifiers=[
|
|
"Development Status :: 2 - Pre-Alpha",
|
|
"Intended Audience :: Developers",
|
|
"Topic :: System",
|
|
"Topic :: System :: Installation/Setup",
|
|
"Topic :: System :: Software Distribution",
|
|
"Programming Language :: Python :: 2",
|
|
"Programming Language :: Python :: 2.7",
|
|
"Programming Language :: Python :: 3",
|
|
"Programming Language :: Python :: 3.5",
|
|
"License :: OSI Approved :: Apache Software License",
|
|
],
|
|
url='https://github.com/openstack-charmers/charms.openstack',
|
|
author='Alex Kavanagh',
|
|
author_email='alex.kavanagh@canonical.com',
|
|
license='Apache-2.0: http://www.apache.org/licenses/LICENSE-2.0',
|
|
packages=find_packages(exclude=["unit_tests"]),
|
|
exclude_package_data={'': ['.gitignore', '.git']},
|
|
zip_safe=False,
|
|
cmdclass={'test': Tox},
|
|
install_requires=install_require,
|
|
extras_require={
|
|
'testing': tests_require,
|
|
},
|
|
tests_require=tests_require,
|
|
)
|