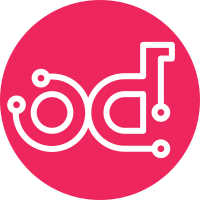
Add new method for calling openstack clis Add new method for getting uuid via cli These methods are based upon the identity register provider ones Add tests Related change in network: I89dd47c802a544bdd998059b36884cb10f628223 Change-Id: I2b2f1d94dbd67550ad3a352c2205a532173f6065 Implements: blueprint neutron-icehouse-default-changes
105 lines
3.3 KiB
Ruby
Executable File
105 lines
3.3 KiB
Ruby
Executable File
# encoding: UTF-8
|
|
require_relative 'spec_helper'
|
|
require ::File.join ::File.dirname(__FILE__), '..', 'libraries', 'cli'
|
|
|
|
describe 'openstack-common::default' do
|
|
describe 'Openstack CLI' do
|
|
let(:runner) { ChefSpec::Runner.new(CHEFSPEC_OPTS) }
|
|
let(:node) { runner.node }
|
|
let(:chef_run) do
|
|
runner.converge(described_recipe)
|
|
end
|
|
let(:subject) { Object.new.extend(Openstack) }
|
|
|
|
include_context 'library-stubs'
|
|
|
|
describe 'openstack_command_env' do
|
|
it 'returns cli enviroment' do
|
|
subject.stub(:get_password)
|
|
.with('user', 'name')
|
|
.and_return('pass')
|
|
|
|
expect(
|
|
subject.openstack_command_env('name', 'tenant')
|
|
).to eq(
|
|
'OS_USERNAME' => 'name',
|
|
'OS_PASSWORD' => 'pass',
|
|
'OS_TENANT_NAME' => 'tenant',
|
|
'OS_AUTH_URL' => 'http://127.0.0.1:35357/v2.0'
|
|
)
|
|
end
|
|
end
|
|
|
|
describe 'openstack_command' do
|
|
it 'runs openstack command' do
|
|
env =
|
|
{
|
|
'OS_USERNAME' => 'name',
|
|
'OS_PASSWORD' => 'pass',
|
|
'OS_TENANT_NAME' => 'tenant',
|
|
'OS_AUTH_URL' => 'http://127.0.0.1:35357/v2.0'
|
|
}
|
|
subject.stub(:shell_out).with(
|
|
['keystone', 'user-list'],
|
|
env: env
|
|
).and_return double('shell_out', exitstatus: 0, stdout: 'good', stderr: '')
|
|
|
|
result = subject.openstack_command('keystone', 'user-list', env)
|
|
expect(result).to eq('good')
|
|
end
|
|
|
|
it 'runs openstack command with args' do
|
|
env =
|
|
{
|
|
'OS_USERNAME' => 'name',
|
|
'OS_PASSWORD' => 'pass',
|
|
'OS_TENANT_NAME' => 'tenant',
|
|
'OS_AUTH_URL' => 'http://127.0.0.1:35357/v2.0'
|
|
}
|
|
subject.stub(:shell_out).with(
|
|
%w(keystone user-list --key1 value1 --key2 value2),
|
|
env: env
|
|
).and_return double('shell_out', exitstatus: 0, stdout: 'good', stderr: '')
|
|
|
|
result = subject.openstack_command('keystone', 'user-list', env, 'key1' => 'value1', 'key2' => 'value2')
|
|
expect(result).to eq('good')
|
|
end
|
|
|
|
it 'runs openstack command with failure' do
|
|
env =
|
|
{
|
|
'OS_USERNAME' => 'name',
|
|
'OS_PASSWORD' => 'pass',
|
|
'OS_TENANT_NAME' => 'tenant',
|
|
'OS_AUTH_URL' => 'http://127.0.0.1:35357/v2.0'
|
|
}
|
|
subject.stub(:shell_out).with(
|
|
['keystone', 'user-list'],
|
|
env: env
|
|
).and_return double('shell_out', exitstatus: 123, stdout: 'fail', stderr: '')
|
|
|
|
# TODO: need to figure out why this won't work.
|
|
# expect(subject.openstack_command('keystone', 'user-list', env)).to fail
|
|
end
|
|
end
|
|
|
|
describe 'identity_uuid' do
|
|
it 'runs identity command to query uuid' do
|
|
env =
|
|
{
|
|
'OS_USERNAME' => 'name',
|
|
'OS_PASSWORD' => 'pass',
|
|
'OS_TENANT_NAME' => 'tenant',
|
|
'OS_AUTH_URL' => 'http://127.0.0.1:35357/v2.0'
|
|
}
|
|
subject.stub(:openstack_command).with('keystone', 'user-list', env, {})
|
|
subject.stub(:prettytable_to_array)
|
|
.and_return([{ 'name' => 'user1', 'id' => '1234567890ABCDEFGH' }])
|
|
|
|
result = subject.identity_uuid('user', 'name', 'user1', env)
|
|
expect(result).to eq('1234567890ABCDEFGH')
|
|
end
|
|
end
|
|
end
|
|
end
|