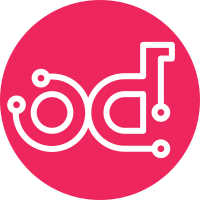
Now that almost all of the rubocop blueprints have been completed, make one final pass through all of the cookbooks ensuring they're all in sync with each other. - Upgrade rubocop to 0.18.1 - Fix violations caused by 0.18.1 upgrade - Move Excludes for non-existent folders to Includes so they automatically cover future additions Change-Id: Id66903d1ca0f5fc0fa3323cca2ec6880730db3fe Implements: blueprint lint-and-unit-testing-for-havana
59 lines
2.0 KiB
Ruby
59 lines
2.0 KiB
Ruby
# encoding: UTF-8
|
|
|
|
#
|
|
# Cookbook Name:: openstack-common
|
|
# library:: parse
|
|
#
|
|
# Copyright 2013, Craig Tracey <craigtracey@gmail.com>
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
module ::Openstack # rubocop:disable Documentation
|
|
# The current state of (at least some) OpenStack CLI tools do not provide a
|
|
# mechanism for outputting data in formats other than PrettyTable output.
|
|
# Therefore this function is intended to parse PrettyTable output into a
|
|
# usable array of hashes. Similarly, it will flatten Property/Value tables
|
|
# into a single element array.
|
|
# table - the raw PrettyTable output of the CLI command
|
|
# output - array of hashes representing the data.
|
|
def prettytable_to_array(table) # rubocop:disable MethodLength
|
|
ret = []
|
|
return ret if table.nil?
|
|
indicies = []
|
|
(table.split(/$/).map { |x| x.strip }).each do |line|
|
|
unless line.start_with?('+--') || line.empty?
|
|
cols = line.split('|').map { |x| x.strip }
|
|
cols.shift
|
|
if indicies == []
|
|
indicies = cols
|
|
next
|
|
end
|
|
newobj = {}
|
|
cols.each { |val| newobj[indicies[newobj.length]] = val }
|
|
ret.push(newobj)
|
|
end
|
|
end
|
|
|
|
# this kinda sucks, but some prettytable data comes
|
|
# as Property Value pairs. If this is the case, then
|
|
# flatten it as expected.
|
|
newobj = {}
|
|
if indicies == ['Property', 'Value']
|
|
ret.each { |x| newobj[x['Property']] = x['Value'] }
|
|
[newobj]
|
|
else
|
|
ret
|
|
end
|
|
end
|
|
end
|