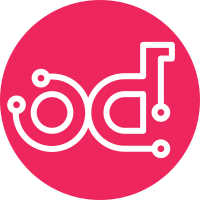
* version bump to 13.0.0 for mitaka release * removed suse support * removed general endpoint method, since we should be able to always specify which endpoint we need * removed fallbacks in specific_endpoint method, since this behaviour is not a very obvious one to the user and it should rather return an error than an unexpected result * dry public, internal and admin endpoint methods * removed obsolete private methods * adapted method calls for admin_endpoint in libraries/cli.rb * refactored set_endpoints_by_interface recipe to directly call address_for instead of address, since the recipe already checks for an existing attribute ..['bind_interface'] and therefore address would redirect to address_for anyways * moved the nested hash order for the public, internal and admin attributes to to be more clear and to break all existing calls to fix them during the refactoring process of all cookbooks e.g: node['openstack']['endpoints']['internal']['identity'] is now node['openstack']['endpoints']['identity']['internal'] and can be moved into the identity cookbook. This also streamlines these endpoint attributes with the bind_interface and host attributes * removed dependency on openstack-identity cookbooks by moving openrc recipe to opentack-identity (same for corrensponding specs and template) * removed address method and use the address (or hostname) defined in the endpoints hash directly (logic to set this attribute should rather be done in a wrapper (with a fitting method) instead of a static and predefined one) * removed set_endpoints_by_interface recipe since logic for defining the endpoints will be moved to wrapper cookbooks * added helper method merge_config_options for generation of config hashes used in service config templates * added template for openstack-service.conf.erb which can be used by all service cookbooks * deleted all endpoints attibutes, since these are moved to the service cookbooks for easier dependency handling Implements: blueprint cookbook-refactoring Change-Id: I0547182085eed91d05384fdd7734408a839a9a2c
126 lines
3.3 KiB
Ruby
126 lines
3.3 KiB
Ruby
# encoding: UTF-8
|
|
require_relative 'spec_helper'
|
|
require ::File.join ::File.dirname(__FILE__), '..', 'libraries', 'uri'
|
|
require 'uri'
|
|
|
|
describe 'Openstack uri' do
|
|
let(:subject) { Object.new.extend(Openstack) }
|
|
|
|
describe '#uri_from_hash' do
|
|
it 'returns uri when uri key found, ignoring other parts' do
|
|
uri = 'http://localhost/'
|
|
hash = {
|
|
'port' => 8888,
|
|
'path' => '/path',
|
|
'uri' => uri
|
|
}
|
|
result = subject.uri_from_hash(hash)
|
|
expect(result).to be_a URI
|
|
expect(result.to_s).to eq(uri)
|
|
end
|
|
|
|
it 'constructs from host' do
|
|
uri = 'https://localhost:8888/path'
|
|
hash = {
|
|
'scheme' => 'https',
|
|
'port' => 8888,
|
|
'path' => '/path',
|
|
'host' => 'localhost'
|
|
}
|
|
expect(
|
|
subject.uri_from_hash(hash).to_s
|
|
).to eq(uri)
|
|
end
|
|
|
|
it 'constructs with defaults' do
|
|
uri = 'https://localhost'
|
|
hash = {
|
|
'scheme' => 'https',
|
|
'host' => 'localhost'
|
|
}
|
|
expect(
|
|
subject.uri_from_hash(hash).to_s
|
|
).to eq(uri)
|
|
end
|
|
|
|
it 'constructs with extraneous keys' do
|
|
uri = 'http://localhost'
|
|
hash = {
|
|
'host' => 'localhost',
|
|
'network' => 'public' # To emulate the osops-utils::ip_location way...
|
|
}
|
|
expect(
|
|
subject.uri_from_hash(hash).to_s
|
|
).to eq(uri)
|
|
end
|
|
end
|
|
|
|
describe '#uri_join_paths' do
|
|
it 'returns nil when no paths are passed in' do
|
|
expect(subject.uri_join_paths).to be_nil
|
|
end
|
|
|
|
it 'preserves absolute path when only absolute path passed in' do
|
|
path = '/abspath'
|
|
expect(
|
|
subject.uri_join_paths(path)
|
|
).to eq(path)
|
|
end
|
|
|
|
it 'preserves relative path when only relative path passed in' do
|
|
path = 'abspath/'
|
|
expect(
|
|
subject.uri_join_paths(path)
|
|
).to eq(path)
|
|
end
|
|
|
|
it 'preserves leadng and trailing slashes' do
|
|
expected = '/path/to/resource/'
|
|
expect(
|
|
subject.uri_join_paths('/path', 'to', 'resource/')
|
|
).to eq(expected)
|
|
end
|
|
|
|
it 'removes extraneous intermediate slashes' do
|
|
expected = '/path/to/resource'
|
|
expect(
|
|
subject.uri_join_paths('/path', '//to/', '/resource')
|
|
).to eq(expected)
|
|
end
|
|
end
|
|
|
|
describe '#auth_uri_transform' do
|
|
it 'preserves the original auth uri when the auth version passed is v2.0' do
|
|
auth_version = 'v2.0'
|
|
auth_uri = 'http://localhost:5000/v2.0'
|
|
expect(
|
|
subject.auth_uri_transform(auth_uri, auth_version)
|
|
).to eq(auth_uri)
|
|
end
|
|
|
|
it 'substitute /v2.0 with /v3 in the passed auth uri when auth version passed is v3.0' do
|
|
auth_version = 'v3.0'
|
|
auth_uri = 'http://localhost:5000/v2.0'
|
|
expected_auth_uri = 'http://localhost:5000/v3'
|
|
expect(
|
|
subject.auth_uri_transform(auth_uri, auth_version)
|
|
).to eq(expected_auth_uri)
|
|
end
|
|
end
|
|
|
|
describe '#identity_uri_transform' do
|
|
it 'removes the path segment from identity admin endpoint' do
|
|
expect(
|
|
subject.identity_uri_transform('http://localhost:35357/v2.0')
|
|
).to eq('http://localhost:35357/')
|
|
end
|
|
|
|
it 'does not effect a valid identity admin endpoint' do
|
|
identity_uri = 'http://localhost:35357/'
|
|
expect(
|
|
subject.identity_uri_transform(identity_uri)
|
|
).to eq(identity_uri)
|
|
end
|
|
end
|
|
end
|