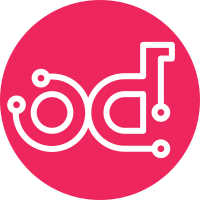
This uses edit_resource to add a notification in the identity apache configuration when it gets updated. This is a workaround due to the fact we are using a version of the apache2 cookbook that is still using definitions and cannot add notifications with definitions. This is intended to ensure we only restart apache when the configuration is updated. Otherwise, the old behaviour was to restart apache on every run which is problematic in production environments. I have been using this in our production wrapper cookbook for the past year or so without any issue. This will be removed in the Stein release when we migrate to the newer apache2 cookbook which uses proper resources. Change-Id: I13de063d1e7ffd356d754eb0f2d8286a3c694836 Signed-off-by: Lance Albertson <lance@osuosl.org>
89 lines
2.9 KiB
Ruby
89 lines
2.9 KiB
Ruby
# Encoding: UTF-8
|
|
require 'chefspec'
|
|
require 'chefspec/berkshelf'
|
|
|
|
ChefSpec::Coverage.start! { add_filter 'openstack-identity' }
|
|
|
|
RSpec.configure do |config|
|
|
config.color = true
|
|
config.formatter = :documentation
|
|
config.log_level = :fatal
|
|
config.file_cache_path = '/var/chef/cache'
|
|
end
|
|
|
|
REDHAT_OPTS = {
|
|
platform: 'redhat',
|
|
version: '7.4',
|
|
}.freeze
|
|
UBUNTU_OPTS = {
|
|
platform: 'ubuntu',
|
|
version: '16.04',
|
|
}.freeze
|
|
|
|
# Helper methods
|
|
module Helpers
|
|
# Create an anchored regex to exactly match the entire line
|
|
# (name borrowed from grep --line-regexp)
|
|
#
|
|
# @param [String] str The whole line to match
|
|
# @return [Regexp] The anchored/escaped regular expression
|
|
def line_regexp(str)
|
|
/^#{Regexp.quote(str)}$/
|
|
end
|
|
end
|
|
|
|
shared_context 'identity_stubs' do
|
|
before do
|
|
allow_any_instance_of(Chef::Recipe).to receive(:rabbit_servers)
|
|
.and_return('rabbit_servers_value')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:memcached_servers)
|
|
.and_return([])
|
|
allow_any_instance_of(Chef::Recipe).to receive(:get_password)
|
|
.with('db', anything)
|
|
.and_return('')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:get_password)
|
|
.with('user', anything)
|
|
.and_return('')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:get_password)
|
|
.with('user', 'guest')
|
|
.and_return('guest')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:get_password)
|
|
.with('user', 'user1')
|
|
.and_return('secret1')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:get_password)
|
|
.with('user', 'identity_admin')
|
|
.and_return('identity_admin_pass')
|
|
stub_command('/usr/sbin/apache2 -t')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:search_for)
|
|
.with('os-identity').and_return(
|
|
[{
|
|
'openstack' => {
|
|
'identity' => {
|
|
'admin_tenant_name' => 'admin',
|
|
'admin_user' => 'admin',
|
|
},
|
|
},
|
|
}]
|
|
)
|
|
allow_any_instance_of(Chef::Recipe).to receive(:get_password)
|
|
.with('user', 'admin')
|
|
.and_return('admin')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:secret)
|
|
.with('secrets', 'credential_key0')
|
|
.and_return('thisiscredentialkey0')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:secret)
|
|
.with('secrets', 'credential_key1')
|
|
.and_return('thisiscredentialkey1')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:secret)
|
|
.with('secrets', 'fernet_key0')
|
|
.and_return('thisisfernetkey0')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:secret)
|
|
.with('secrets', 'fernet_key1')
|
|
.and_return('thisisfernetkey1')
|
|
allow_any_instance_of(Chef::Recipe).to receive(:rabbit_transport_url)
|
|
.with('identity')
|
|
.and_return('rabbit://openstack:mypass@127.0.0.1:5672')
|
|
stub_command("[ ! -e /etc/httpd/conf/httpd.conf ] && [ -e /etc/redhat-release ] && [ $(/sbin/sestatus | grep -c '^Current mode:.*enforcing') -eq 1 ]").and_return(true)
|
|
end
|
|
end
|