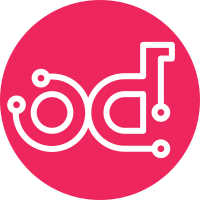
This change make the ceilometer-api getting rid the pipeline. samples will be push to notification bus when creating via /v2/meters API. To avoid the ceilometer-api's dependency of notification-agent, especially in some testing scenarios, this change also provide a optional parameter that allow samples posted to storage directly. NOTE: This change reverting the reverted patch[1] and merging it with patch[2], the original patch[1] has caused the related bug so has been reverted by patch[3], the patch[2] tried to fix the related bug before the patch[1] been reverted. [1] https://review.openstack.org/#/c/193472/ [2] https://review.openstack.org/#/c/195866/ [3] https://review.openstack.org/#/c/195570/ Related-bug: #1468697 Partially implements: blueprint api-no-pipeline Change-Id: I88cbd83910dcf79bed8a0f9616f98e4346362ab3
61 lines
2.4 KiB
Python
61 lines
2.4 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright 2014 IBM Corp.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import mock
|
|
from oslo_config import cfg
|
|
from oslo_config import fixture as fixture_config
|
|
from oslo_log import log
|
|
|
|
from ceilometer.api import app
|
|
from ceilometer.tests import base
|
|
|
|
|
|
class TestApp(base.BaseTestCase):
|
|
|
|
def setUp(self):
|
|
super(TestApp, self).setUp()
|
|
self.CONF = self.useFixture(fixture_config.Config()).conf
|
|
log.register_options(cfg.CONF)
|
|
|
|
def test_api_paste_file_not_exist(self):
|
|
self.CONF.set_override('api_paste_config', 'non-existent-file')
|
|
with mock.patch.object(self.CONF, 'find_file') as ff:
|
|
ff.return_value = None
|
|
self.assertRaises(cfg.ConfigFilesNotFoundError, app.load_app)
|
|
|
|
@mock.patch('ceilometer.storage.get_connection_from_config',
|
|
mock.MagicMock())
|
|
@mock.patch('pecan.make_app')
|
|
def test_pecan_debug(self, mocked):
|
|
def _check_pecan_debug(g_debug, p_debug, expected, workers=1):
|
|
self.CONF.set_override('debug', g_debug)
|
|
if p_debug is not None:
|
|
self.CONF.set_override('pecan_debug', p_debug, group='api')
|
|
self.CONF.set_override('api_workers', workers)
|
|
app.setup_app()
|
|
args, kwargs = mocked.call_args
|
|
self.assertEqual(expected, kwargs.get('debug'))
|
|
|
|
_check_pecan_debug(g_debug=False, p_debug=None, expected=False)
|
|
_check_pecan_debug(g_debug=True, p_debug=None, expected=False)
|
|
_check_pecan_debug(g_debug=True, p_debug=False, expected=False)
|
|
_check_pecan_debug(g_debug=False, p_debug=True, expected=True)
|
|
_check_pecan_debug(g_debug=True, p_debug=None, expected=False,
|
|
workers=5)
|
|
_check_pecan_debug(g_debug=False, p_debug=True, expected=False,
|
|
workers=5)
|