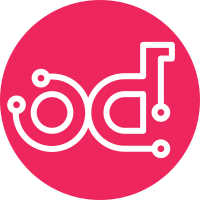
This code is actually dead code we carry over for a long time now, as the pagination support never landed completely. Let's clean that old cruft. Change-Id: Idbb2c93ed00dc2359f555d245c2283844d5a1da4
167 lines
6.4 KiB
Python
167 lines
6.4 KiB
Python
#
|
|
# Copyright 2012 New Dream Network, LLC (DreamHost)
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
"""Base classes for storage engines
|
|
"""
|
|
import ceilometer
|
|
|
|
|
|
class Connection(object):
|
|
"""Base class for alarm storage system connections."""
|
|
|
|
# A dictionary representing the capabilities of this driver.
|
|
CAPABILITIES = {
|
|
'alarms': {'query': {'simple': False,
|
|
'complex': False},
|
|
'history': {'query': {'simple': False,
|
|
'complex': False}}},
|
|
}
|
|
|
|
STORAGE_CAPABILITIES = {
|
|
'storage': {'production_ready': False},
|
|
}
|
|
|
|
def __init__(self, url):
|
|
pass
|
|
|
|
@staticmethod
|
|
def upgrade():
|
|
"""Migrate the database to `version` or the most recent version."""
|
|
|
|
@staticmethod
|
|
def get_alarms(name=None, user=None, state=None, meter=None,
|
|
project=None, enabled=None, alarm_id=None,
|
|
alarm_type=None, severity=None):
|
|
"""Yields a lists of alarms that match filters.
|
|
|
|
:param name: Optional name for alarm.
|
|
:param user: Optional ID for user that owns the resource.
|
|
:param state: Optional string for alarm state.
|
|
:param meter: Optional string for alarms associated with meter.
|
|
:param project: Optional ID for project that owns the resource.
|
|
:param enabled: Optional boolean to list disable alarm.
|
|
:param alarm_id: Optional alarm_id to return one alarm.
|
|
:param alarm_type: Optional alarm type.
|
|
:parmr severity: Optional alarm severity
|
|
"""
|
|
raise ceilometer.NotImplementedError('Alarms not implemented')
|
|
|
|
@staticmethod
|
|
def create_alarm(alarm):
|
|
"""Create an alarm. Returns the alarm as created.
|
|
|
|
:param alarm: The alarm to create.
|
|
"""
|
|
raise ceilometer.NotImplementedError('Alarms not implemented')
|
|
|
|
@staticmethod
|
|
def update_alarm(alarm):
|
|
"""Update alarm."""
|
|
raise ceilometer.NotImplementedError('Alarms not implemented')
|
|
|
|
@staticmethod
|
|
def delete_alarm(alarm_id):
|
|
"""Delete an alarm."""
|
|
raise ceilometer.NotImplementedError('Alarms not implemented')
|
|
|
|
@staticmethod
|
|
def get_alarm_changes(alarm_id, on_behalf_of,
|
|
user=None, project=None, alarm_type=None,
|
|
severity=None, start_timestamp=None,
|
|
start_timestamp_op=None, end_timestamp=None,
|
|
end_timestamp_op=None):
|
|
"""Yields list of AlarmChanges describing alarm history
|
|
|
|
Changes are always sorted in reverse order of occurrence, given
|
|
the importance of currency.
|
|
|
|
Segregation for non-administrative users is done on the basis
|
|
of the on_behalf_of parameter. This allows such users to have
|
|
visibility on both the changes initiated by themselves directly
|
|
(generally creation, rule changes, or deletion) and also on those
|
|
changes initiated on their behalf by the alarming service (state
|
|
transitions after alarm thresholds are crossed).
|
|
|
|
:param alarm_id: ID of alarm to return changes for
|
|
:param on_behalf_of: ID of tenant to scope changes query (None for
|
|
administrative user, indicating all projects)
|
|
:param user: Optional ID of user to return changes for
|
|
:param project: Optional ID of project to return changes for
|
|
:param alarm_type: Optional change type
|
|
:param severity: Optional change severity
|
|
:param start_timestamp: Optional modified timestamp start range
|
|
:param start_timestamp_op: Optional timestamp start range operation
|
|
:param end_timestamp: Optional modified timestamp end range
|
|
:param end_timestamp_op: Optional timestamp end range operation
|
|
"""
|
|
raise ceilometer.NotImplementedError('Alarm history not implemented')
|
|
|
|
@staticmethod
|
|
def record_alarm_change(alarm_change):
|
|
"""Record alarm change event."""
|
|
raise ceilometer.NotImplementedError('Alarm history not implemented')
|
|
|
|
@staticmethod
|
|
def clear():
|
|
"""Clear database."""
|
|
|
|
@staticmethod
|
|
def query_alarms(filter_expr=None, orderby=None, limit=None):
|
|
"""Return an iterable of model.Alarm objects.
|
|
|
|
:param filter_expr: Filter expression for query.
|
|
:param orderby: List of field name and direction pairs for order by.
|
|
:param limit: Maximum number of results to return.
|
|
"""
|
|
|
|
raise ceilometer.NotImplementedError('Complex query for alarms '
|
|
'is not implemented.')
|
|
|
|
@staticmethod
|
|
def query_alarm_history(filter_expr=None, orderby=None, limit=None):
|
|
"""Return an iterable of model.AlarmChange objects.
|
|
|
|
:param filter_expr: Filter expression for query.
|
|
:param orderby: List of field name and direction pairs for order by.
|
|
:param limit: Maximum number of results to return.
|
|
"""
|
|
|
|
raise ceilometer.NotImplementedError('Complex query for alarms '
|
|
'history is not implemented.')
|
|
|
|
@classmethod
|
|
def get_capabilities(cls):
|
|
"""Return an dictionary with the capabilities of each driver."""
|
|
return cls.CAPABILITIES
|
|
|
|
@classmethod
|
|
def get_storage_capabilities(cls):
|
|
"""Return a dictionary representing the performance capabilities.
|
|
|
|
This is needed to evaluate the performance of each driver.
|
|
"""
|
|
return cls.STORAGE_CAPABILITIES
|
|
|
|
@staticmethod
|
|
def clear_expired_alarm_history_data(alarm_history_ttl):
|
|
"""Clear expired alarm history data from the backend storage system.
|
|
|
|
Clearing occurs according to the time-to-live.
|
|
|
|
:param alarm_history_ttl: Number of seconds to keep alarm history
|
|
records for.
|
|
"""
|
|
raise ceilometer.NotImplementedError('Clearing alarm history '
|
|
'not implemented')
|