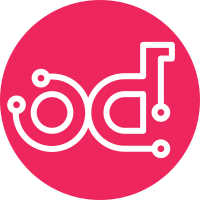
This changeset reimplements the API using Pecan and WSME instead of Flask. Pecan uses "object dispatch" instead of declared routes. The controller classes are chained together to implement the API. Most of what we have are simple REST lookups, but a few cases required custom methods. WSME is used to define types of inputs and outputs for each controller method. The WSME layer handles serizlization and deserialization in several formats. In our case, only JSON and XML are configured. There are a few small changes to the return types in the API, as well as to error handling. Now all errors are returned as JSON messages made up of a mapping containing the key 'error_message' and the text of the error. This will later be enhanced to include XML support for XML requests. This change also moves the script for starting the V1 API to a new name and replaces it with a script that starts the V2 API. There is an open bug/blueprint to fix that so both versions of the API are loaded. blueprint api-server-pecan-wsme Signed-off-by: Doug Hellmann <doug.hellmann@dreamhost.com> Change-Id: I1b99a16de68f902370a8999eca073c56f9f14865
56 lines
1.7 KiB
Python
56 lines
1.7 KiB
Python
# -*- encoding: utf-8 -*-
|
|
#
|
|
# Copyright © 2012 New Dream Network, LLC (DreamHost)
|
|
#
|
|
# Author: Doug Hellmann <doug.hellmann@dreamhost.com>
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
"""Set up the ACL to acces the API server."""
|
|
|
|
from ceilometer import policy
|
|
|
|
from pecan import hooks
|
|
|
|
from webob import exc
|
|
|
|
import keystoneclient.middleware.auth_token as auth_token
|
|
|
|
|
|
def register_opts(conf):
|
|
"""Register keystoneclient middleware options
|
|
"""
|
|
conf.register_opts(auth_token.opts,
|
|
group='keystone_authtoken',
|
|
)
|
|
auth_token.CONF = conf
|
|
|
|
|
|
def install(app, conf):
|
|
"""Install ACL check on application."""
|
|
new_app = auth_token.AuthProtocol(app,
|
|
conf=conf,
|
|
)
|
|
return new_app
|
|
|
|
|
|
class AdminAuthHook(hooks.PecanHook):
|
|
"""Verify that the user has admin rights
|
|
"""
|
|
|
|
def before(self, state):
|
|
headers = state.request.headers
|
|
if not policy.check_is_admin(headers.get('X-Roles', "").split(","),
|
|
headers.get('X-Tenant-Id'),
|
|
headers.get('X-Tenant-Name')):
|
|
raise exc.HTTPUnauthorized()
|