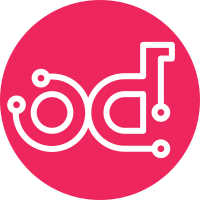
Implements: blueprint add-export-import-volumes Volume manage/unmanage support. This change adds two new API extensions: volume_unmanage.py: Adds an "os-unmanage" action on an existing volume, which causes a delete operation to flow through the stack, with a flag that indicates that a different method ("unmanage") should be called on the driver instead of delete_volume. A default, empty, implementation of unmanage is provided. volume_manage.py Adds a new "os-manage-volume" API. A POST to this URI is very similar to volume creation, except that the images, snapshots and existing volumes cannot be specified. Instead the following must be specified: host: Cinder host on which the existing storage resides ref: Driver-specific reference to the existing storage object name, description, volume_type, metadata and availability_zone are supported as per a normal volume creation. In order to support some re-use between volume_manage and the regular volume creation, add_visible_admin_metadata has been factored out into the cinder utils.py module. The rest of the changes are just the implications of the host/ref specification moving through the api, rpcapi, manager and flow (API and Manager) layers. Management of an existing volume causes the manage_existing_get_size() and manage_existing() methods to be called on the driver, and a reference LVM implementation is provided. brick/local_dev/lvm.py now includes a method to rename an LV. Change-Id: Ifc5255b2fd277c0f60d25fc82a777e405b861320
79 lines
2.8 KiB
Python
Executable File
79 lines
2.8 KiB
Python
Executable File
# Copyright 2014 IBM Corp.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import webob
|
|
from webob import exc
|
|
|
|
from cinder.api import extensions
|
|
from cinder.api.openstack import wsgi
|
|
from cinder import exception
|
|
from cinder.openstack.common import log as logging
|
|
from cinder import volume
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
authorize = extensions.extension_authorizer('volume', 'volume_unmanage')
|
|
|
|
|
|
class VolumeUnmanageController(wsgi.Controller):
|
|
def __init__(self, *args, **kwargs):
|
|
super(VolumeUnmanageController, self).__init__(*args, **kwargs)
|
|
self.volume_api = volume.API()
|
|
|
|
@wsgi.response(202)
|
|
@wsgi.action('os-unmanage')
|
|
def unmanage(self, req, id, body):
|
|
"""Stop managing a volume.
|
|
|
|
This action is very much like a delete, except that a different
|
|
method (unmanage) is called on the Cinder driver. This has the effect
|
|
of removing the volume from Cinder management without actually
|
|
removing the backend storage object associated with it.
|
|
|
|
There are no required parameters.
|
|
|
|
A Not Found error is returned if the specified volume does not exist.
|
|
|
|
A Bad Request error is returned if the specified volume is still
|
|
attached to an instance.
|
|
"""
|
|
context = req.environ['cinder.context']
|
|
authorize(context)
|
|
|
|
LOG.audit(_("Unmanage volume with id: %s"), id, context=context)
|
|
|
|
try:
|
|
vol = self.volume_api.get(context, id)
|
|
self.volume_api.delete(context, vol, unmanage_only=True)
|
|
except exception.NotFound:
|
|
msg = _("Volume could not be found")
|
|
raise exc.HTTPNotFound(explanation=msg)
|
|
except exception.VolumeAttached:
|
|
msg = _("Volume cannot be deleted while in attached state")
|
|
raise exc.HTTPBadRequest(explanation=msg)
|
|
return webob.Response(status_int=202)
|
|
|
|
|
|
class Volume_unmanage(extensions.ExtensionDescriptor):
|
|
"""Enable volume unmanage operation."""
|
|
|
|
name = "VolumeUnmanage"
|
|
alias = "os-volume-unmanage"
|
|
namespace = "http://docs.openstack.org/volume/ext/volume-unmanage/api/v1.1"
|
|
updated = "2012-05-31T00:00:00+00:00"
|
|
|
|
def get_controller_extensions(self):
|
|
controller = VolumeUnmanageController()
|
|
extension = extensions.ControllerExtension(self, 'volumes', controller)
|
|
return [extension]
|