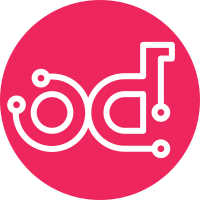
The v2.0 API is stable, so we need to communicate this fact to the consumers of Glance. This updates the status of the v2.0 version entity in responses from / and those that result in a 300 Multiple Choices status code from 'EXPERIMENTAL' to 'CURRENT.' Change-Id: I9e4514025904d7be2e947c6d14ccb90b6b066f8c
108 lines
3.9 KiB
Python
108 lines
3.9 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
|
|
# Copyright 2012 OpenStack LLC.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import json
|
|
|
|
import webob
|
|
|
|
from glance.api.middleware import version_negotiation
|
|
from glance.api import versions
|
|
from glance.tests.unit import base
|
|
|
|
|
|
class VersionsTest(base.IsolatedUnitTest):
|
|
|
|
"""Test the version information returned from the API service."""
|
|
|
|
def test_get_version_list(self):
|
|
req = webob.Request.blank('/', base_url='http://127.0.0.1:9292/')
|
|
req.accept = 'application/json'
|
|
self.config(bind_host='127.0.0.1', bind_port=9292)
|
|
res = versions.Controller().index(req)
|
|
self.assertEqual(res.status_int, 300)
|
|
self.assertEqual(res.content_type, 'application/json')
|
|
results = json.loads(res.body)['versions']
|
|
expected = [
|
|
{
|
|
'id': 'v2.0',
|
|
'status': 'CURRENT',
|
|
'links': [{'rel': 'self',
|
|
'href': 'http://127.0.0.1:9292/v2/'}],
|
|
},
|
|
{
|
|
'id': 'v1.1',
|
|
'status': 'CURRENT',
|
|
'links': [{'rel': 'self',
|
|
'href': 'http://127.0.0.1:9292/v1/'}],
|
|
},
|
|
{
|
|
'id': 'v1.0',
|
|
'status': 'SUPPORTED',
|
|
'links': [{'rel': 'self',
|
|
'href': 'http://127.0.0.1:9292/v1/'}],
|
|
},
|
|
]
|
|
self.assertEqual(results, expected)
|
|
|
|
|
|
class VersionNegotiationTest(base.IsolatedUnitTest):
|
|
|
|
def setUp(self):
|
|
super(VersionNegotiationTest, self).setUp()
|
|
self.middleware = version_negotiation.VersionNegotiationFilter(None)
|
|
|
|
def test_request_url_v1(self):
|
|
request = webob.Request.blank('/v1/images')
|
|
self.middleware.process_request(request)
|
|
self.assertEqual('/v1/images', request.path_info)
|
|
|
|
def test_request_url_v1_0(self):
|
|
request = webob.Request.blank('/v1.0/images')
|
|
self.middleware.process_request(request)
|
|
self.assertEqual('/v1/images', request.path_info)
|
|
|
|
def test_request_url_v1_1(self):
|
|
request = webob.Request.blank('/v1.1/images')
|
|
self.middleware.process_request(request)
|
|
self.assertEqual('/v1/images', request.path_info)
|
|
|
|
def test_request_accept_v1(self):
|
|
request = webob.Request.blank('/images')
|
|
request.headers = {'accept': 'application/vnd.openstack.images-v1'}
|
|
self.middleware.process_request(request)
|
|
self.assertEqual('/v1/images', request.path_info)
|
|
|
|
def test_request_url_v2(self):
|
|
request = webob.Request.blank('/v2/images')
|
|
self.middleware.process_request(request)
|
|
self.assertEqual('/v2/images', request.path_info)
|
|
|
|
def test_request_url_v2_0(self):
|
|
request = webob.Request.blank('/v2.0/images')
|
|
self.middleware.process_request(request)
|
|
self.assertEqual('/v2/images', request.path_info)
|
|
|
|
def test_request_url_v2_1_unsupported(self):
|
|
request = webob.Request.blank('/v2.1/images')
|
|
resp = self.middleware.process_request(request)
|
|
self.assertTrue(isinstance(resp, versions.Controller))
|
|
|
|
def test_request_url_v3_unsupported(self):
|
|
request = webob.Request.blank('/v3/images')
|
|
resp = self.middleware.process_request(request)
|
|
self.assertTrue(isinstance(resp, versions.Controller))
|