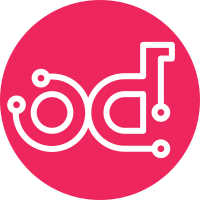
RPC casts never return anything, only RPC calls do, so remove the misleading return in the worker client. Change-Id: I82e2ead0b350be42f808f6cb6b9b57b786aeadcf
50 lines
1.4 KiB
Python
50 lines
1.4 KiB
Python
# Copyright (c) 2014 Hewlett-Packard Development Company, L.P.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
|
|
# implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
"""
|
|
Client side of the heat worker RPC API.
|
|
"""
|
|
|
|
from heat.common import messaging
|
|
from heat.rpc import worker_api
|
|
|
|
|
|
class WorkerClient(object):
|
|
'''Client side of the heat worker RPC API.
|
|
|
|
API version history::
|
|
|
|
1.0 - Initial version.
|
|
'''
|
|
|
|
BASE_RPC_API_VERSION = '1.0'
|
|
|
|
def __init__(self):
|
|
self._client = messaging.get_rpc_client(
|
|
topic=worker_api.TOPIC,
|
|
version=self.BASE_RPC_API_VERSION)
|
|
|
|
@staticmethod
|
|
def make_msg(method, **kwargs):
|
|
return method, kwargs
|
|
|
|
def cast(self, ctxt, msg, version=None):
|
|
method, kwargs = msg
|
|
if version is not None:
|
|
client = self._client.prepare(version=version)
|
|
else:
|
|
client = self._client
|
|
client.cast(ctxt, method, **kwargs)
|