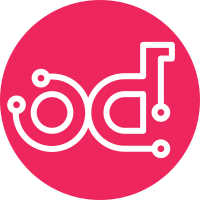
* Refactored tests hierarcy (moved get_resource to the base class) * Fixed logic in abstract engine (transaction scoope was wrong) * Added stub methods for context manipulations (not everywhere yet) * Refactored actions * Created ECHO action to be able to emulate required output * Added simple data flow test (two dependent tasks) TODO: * More tests * Refactor actions according to the latest discussions Change-Id: I5ba5e330110889014eeca53501ddae54dc9a1236
63 lines
1.8 KiB
Python
63 lines
1.8 KiB
Python
# -*- coding: utf-8 -*-
|
|
#
|
|
# Copyright 2013 - Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
import abc
|
|
import yaql
|
|
|
|
from mistral.openstack.common import log as logging
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
|
|
class Evaluator(object):
|
|
"""Expression evaluator interface.
|
|
|
|
Having this interface gives the flexibility to change the actual expression
|
|
language used in Mistral DSL for conditions, output calculation etc.
|
|
"""
|
|
|
|
@classmethod
|
|
@abc.abstractmethod
|
|
def evaluate(cls, expression, context):
|
|
"""Evaluates the expression against the given data context.
|
|
|
|
:param expression: Expression string
|
|
:param context: Data context
|
|
:return: Expression result
|
|
"""
|
|
pass
|
|
|
|
|
|
class YAQLEvaluator(Evaluator):
|
|
@classmethod
|
|
def evaluate(cls, expression, context):
|
|
LOG.debug("Evaluating YAQL expression [expression='%s', context=%s]")
|
|
|
|
return yaql.parse(expression).evaluate(context)
|
|
|
|
|
|
# TODO(rakhmerov): Make it configurable.
|
|
_EVALUATOR = YAQLEvaluator()
|
|
|
|
|
|
def is_expression(s):
|
|
# TODO(rakhmerov): It should be generalized since it may not be YAQL.
|
|
return s and s.startswith('$.')
|
|
|
|
|
|
def evaluate(expression, context):
|
|
return _EVALUATOR.evaluate(expression, context)
|