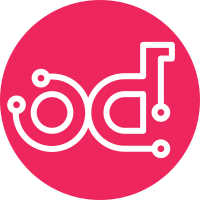
* In order to avoid duplicates of same 'join' tasks we now use named locks to exclusively create a task execution for 'join' tasks in DB. Transaction that does that is always separate from task completion logic and is very short. This is needed to eliminate DB contention on same records of task execution table. This is also a reason to use a separate mechanism such as named locks, and additionally this reduces a number possible scenarios for getting into deadlocks because for task executions we have too many different access patterns that can lead to them in case of doing locking on right on their table records. So this approach guarantees that there's only one transaction creates a new task execution object for 'join' task and schedules 'refresh_task_state' job that check 'join' completion. * Dropped scheduler 'unique_key' column with unique constraint because in practice it causes DB deadlocks (at least on MySQL) while simultaneously inserting and updating the table * Instead of 'unique_key' column we added non-unique 'key' column that can potentially be used for squashing delayed calls by scheduler itself (not implemented yet) * Adjusted Scheduler implementation and tests accordingly * Fixed task() YAQL function to work without precisely resolve task execution object in case it's called for the current task. Previously it was dependent on the luck and we were lucky enough that tests were passing. * Increased length of 'unique_key' column for task executions to 250 which is close to a limit for string fields participating in unique constraints. Change-Id: Ib7aaa20c2c8834ab0f2d9c90457677c9edb62805
76 lines
2.5 KiB
Python
76 lines
2.5 KiB
Python
# Copyright 2013 - Mirantis, Inc.
|
|
# Copyright 2015 - StackStorm, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
from mistral.engine import dispatcher
|
|
from mistral.tests.unit import base
|
|
from mistral.workflow import commands
|
|
|
|
|
|
def _print_commands(cmds):
|
|
print("commands:")
|
|
|
|
for cmd in cmds:
|
|
if isinstance(cmd, commands.RunTask):
|
|
print("%s, %s, %s" % (type(cmd), cmd.is_waiting(), cmd.unique_key))
|
|
else:
|
|
print("%s" % type(cmd))
|
|
|
|
|
|
class CommandDispatcherTest(base.BaseTest):
|
|
def setUp(self):
|
|
super(CommandDispatcherTest, self).setUp()
|
|
|
|
def test_rearrange_commands(self):
|
|
no_wait = commands.RunTask(None, None, None, None)
|
|
fail = commands.FailWorkflow(None, None, None, None)
|
|
succeed = commands.SucceedWorkflow(None, None, None, None)
|
|
|
|
wait1 = commands.RunTask(None, None, None, None)
|
|
wait1.wait = True
|
|
wait1.unique_key = 'wait1'
|
|
|
|
wait2 = commands.RunTask(None, None, None, None)
|
|
wait2.wait = True
|
|
wait2.unique_key = 'wait2'
|
|
|
|
wait3 = commands.RunTask(None, None, None, None)
|
|
wait3.wait = True
|
|
wait3.unique_key = 'wait3'
|
|
|
|
# 'set state' command is the first, others must be ignored.
|
|
initial = [fail, no_wait, wait1, wait3, wait2]
|
|
expected = [fail]
|
|
|
|
cmds = dispatcher._rearrange_commands(initial)
|
|
|
|
self.assertEqual(expected, cmds)
|
|
|
|
# 'set state' command is the last, tasks before it must be sorted.
|
|
initial = [no_wait, wait2, wait1, wait3, succeed]
|
|
expected = [no_wait, wait1, wait2, wait3, succeed]
|
|
|
|
cmds = dispatcher._rearrange_commands(initial)
|
|
|
|
self.assertEqual(expected, cmds)
|
|
|
|
# 'set state' command is in the middle, tasks before it must be sorted
|
|
# and the task after it must be ignored.
|
|
initial = [wait3, wait2, no_wait, succeed, wait1]
|
|
expected = [no_wait, wait2, wait3, succeed]
|
|
|
|
cmds = dispatcher._rearrange_commands(initial)
|
|
|
|
self.assertEqual(expected, cmds)
|