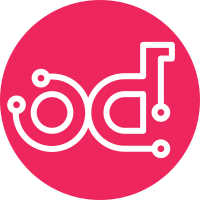
* Introduced class hierarchies Task and Action used by Mistral engine. Note: Action here is a different than executor Action and represents rather actions of different types: regular python action, ad-hoc action and workflow action (since for task action and workflow are polymorphic) * Refactored task_handler.py and action_handler.py with Task and Action hierarchies * Rebuilt a chain call so that the entire action processing would look like a chain of calls Action -> Task -> Workflow where each level knows only about the next level and can influence it (e.g. if adhoc action has failed due to YAQL error in 'output' transformer action itself fails its task) * Refactored policies according to new object model * Fixed some of the tests to match the idea of having two types of exceptions, MistralException and MistralError, where the latter is considered either a harsh environmental problem or a logical issue in the system itself so that it must not be handled anywhere in the code TODO(in subsequent patches): * Refactor WithItemsTask w/o using with_items.py * Remove DB transaction in Scheduler when making a delayed call, helper policy methods like 'continue_workflow' * Refactor policies test so that workflow definitions live right in test methods * Refactor workflow_handler with Workflow abstraction * Get rid of RunExistingTask workflow command, it should be just one command with various properties * Refactor resume and rerun with Task abstraction (same way as other methods, e.g. on_action_complete()) * Add error handling to all required places such as task_handler.continue_task() * More tests for error handling P.S. This patch is very big but it was nearly impossible to split it into multiple smaller patches just because how entangled everything was in Mistral Engine. Partially implements: blueprint mistral-engine-error-handling Implements: blueprint mistral-action-result-processing-pipeline Implements: blueprint mistral-refactor-task-handler Closes-Bug: #1568909 Change-Id: I0668e695c60dde31efc690563fc891387d44d6ba
93 lines
2.6 KiB
Python
93 lines
2.6 KiB
Python
# Copyright 2015 - Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
from oslo_log import log as logging
|
|
import traceback as tb
|
|
|
|
from mistral.db.v2.sqlalchemy import models
|
|
from mistral.engine import actions
|
|
from mistral.engine import task_handler
|
|
from mistral import exceptions as exc
|
|
from mistral.workbook import parser as spec_parser
|
|
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
|
|
def on_action_complete(action_ex, result):
|
|
task_ex = action_ex.task_execution
|
|
|
|
action = _build_action(action_ex)
|
|
|
|
try:
|
|
action.complete(result)
|
|
except exc.MistralException as e:
|
|
msg = ("Failed to complete action [action=%s, task=%s]: %s\n%s" %
|
|
(action_ex.name, task_ex.name, e, tb.format_exc()))
|
|
|
|
LOG.error(msg)
|
|
|
|
action.fail(msg)
|
|
|
|
if task_ex:
|
|
task_handler.fail_task(task_ex, msg)
|
|
|
|
return
|
|
|
|
if task_ex:
|
|
task_handler.on_action_complete(action_ex)
|
|
|
|
|
|
def _build_action(action_ex):
|
|
if isinstance(action_ex, models.WorkflowExecution):
|
|
return actions.WorkflowAction(None, action_ex=action_ex)
|
|
|
|
wf_name = None
|
|
wf_spec_name = None
|
|
|
|
if action_ex.workflow_name:
|
|
wf_name = action_ex.workflow_name
|
|
wf_spec = spec_parser.get_workflow_spec(
|
|
action_ex.task_execution.workflow_execution.spec
|
|
)
|
|
wf_spec_name = wf_spec.get_name()
|
|
|
|
adhoc_action_name = action_ex.runtime_context.get('adhoc_action_name')
|
|
|
|
if adhoc_action_name:
|
|
action_def = actions.resolve_action_definition(
|
|
adhoc_action_name,
|
|
wf_name,
|
|
wf_spec_name
|
|
)
|
|
|
|
return actions.AdHocAction(action_def, action_ex=action_ex)
|
|
|
|
action_def = actions.resolve_action_definition(
|
|
action_ex.name,
|
|
wf_name,
|
|
wf_spec_name
|
|
)
|
|
|
|
return actions.PythonAction(action_def, action_ex=action_ex)
|
|
|
|
|
|
def build_action_by_name(action_name):
|
|
action_def = actions.resolve_action_definition(action_name)
|
|
|
|
action_cls = (actions.PythonAction if not action_def.spec
|
|
else actions.AdHocAction)
|
|
|
|
return action_cls(action_def)
|