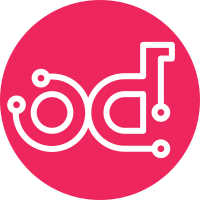
Currently, the api endpoint function for listing packages returns all packages by default. This commit adds a new 'include_disabled' parameter which, when specified by an admin as 'True', results in the packages search results including disabled packages from all users in addition to all enabled packages. When the include_disabled parameter is specified 'True' by non-admins, the search will include both all enabled packages (public and owned), plus only the disabled packages owned by the searcher. If a non-admin specifies 'True' for the include_disabled paramater AND also specifies the 'owned' parameter, then the non-admin gets all of their own packages, public and private, both enabled and disabled. This commit effectively disables the 'owned' parameter for admin users. It is the only way to make the package search API function usable for both generating views of packages for deployment as well as views of packages for editing packages. Change-Id: I28dfc49e1559dd9f0a87b89936df3e4b5c938264 Partial-Bug: #1307963
78 lines
2.6 KiB
Python
78 lines
2.6 KiB
Python
# Copyright (c) 2013 Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from muranoapi.db import models
|
|
from muranoapi.db import session as db_session
|
|
|
|
stats = None
|
|
|
|
SUPPORTED_PARAMS = ('order_by', 'category', 'marker', 'tag', 'class_name',
|
|
'limit', 'type', 'fqn', 'category', 'owned', 'search',
|
|
'include_disabled')
|
|
LIST_PARAMS = ('category', 'tag', 'class', 'order_by')
|
|
ORDER_VALUES = ('fqn', 'name', 'created')
|
|
PKG_PARAMS_MAP = {'display_name': 'name',
|
|
'full_name': 'fully_qualified_name',
|
|
'raw_ui': 'ui_definition',
|
|
'logo': 'logo',
|
|
'package_type': 'type',
|
|
'description': 'description',
|
|
'author': 'author',
|
|
'classes': 'class_definitions',
|
|
'tags': 'tags'}
|
|
|
|
|
|
def get_draft(environment_id=None, session_id=None):
|
|
unit = db_session.get_session()
|
|
#TODO: When session is deployed should be returned env.description
|
|
if session_id:
|
|
session = unit.query(models.Session).get(session_id)
|
|
return session.description
|
|
else:
|
|
environment = unit.query(models.Environment).get(environment_id)
|
|
return environment.description
|
|
|
|
|
|
def save_draft(session_id, draft):
|
|
unit = db_session.get_session()
|
|
session = unit.query(models.Session).get(session_id)
|
|
|
|
session.description = draft
|
|
session.save(unit)
|
|
|
|
|
|
def get_service_status(environment_id, session_id, service):
|
|
status = 'draft'
|
|
|
|
unit = db_session.get_session()
|
|
session_state = unit.query(models.Session).get(session_id).state
|
|
|
|
entities = [u['id'] for u in service['units']]
|
|
reports_count = unit.query(models.Status).filter(
|
|
models.Status.environment_id == environment_id
|
|
and models.Status.session_id == session_id
|
|
and models.Status.entity_id.in_(entities)
|
|
).count()
|
|
|
|
if session_state == 'deployed':
|
|
status = 'finished'
|
|
|
|
if session_state == 'deploying' and reports_count == 0:
|
|
status = 'pending'
|
|
|
|
if session_state == 'deploying' and reports_count > 0:
|
|
status = 'inprogress'
|
|
|
|
return status
|