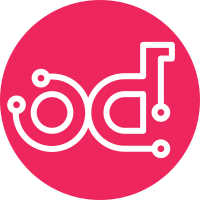
Current the value of TrustId is showed in plaintext in log when murano creates trustes and operates with data. So add 'trustid' in token_sanitizer to hide it like token and pass. Closes-Bug: #1472331 Change-Id: I1e9ea8298a7ffd9aa742cf73fada69db3a734712
65 lines
2.2 KiB
Python
65 lines
2.2 KiB
Python
# Copyright (c) 2013 Mirantis Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
|
|
# implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
import types
|
|
|
|
|
|
class TokenSanitizer(object):
|
|
"""Helper class for cleaning some object from different passwords/tokens.
|
|
Simply searches attribute with `look a like` name as one of
|
|
the token and replace it value with message.
|
|
"""
|
|
def __init__(self, tokens=('token', 'pass', 'trustid'),
|
|
message='*** SANITIZED ***'):
|
|
"""Init method of TokenSanitizer.
|
|
:param tokens: iterable with tokens
|
|
:param message: string by which each token going to be replaced
|
|
"""
|
|
self._tokens = tokens
|
|
self._message = message
|
|
|
|
@property
|
|
def tokens(self):
|
|
"""Iterable with tokens that should be sanitized."""
|
|
return self._tokens
|
|
|
|
@property
|
|
def message(self):
|
|
"""String by which each token going to be replaced."""
|
|
return self._message
|
|
|
|
def _contains_token(self, value):
|
|
for token in self.tokens:
|
|
if token in value.lower():
|
|
return True
|
|
return False
|
|
|
|
def sanitize(self, obj):
|
|
"""Replaces each token found in object by message.
|
|
:param obj: types.DictType, types.ListType, types.Tuple, object
|
|
:return: Sanitized object
|
|
"""
|
|
if isinstance(obj, types.DictType):
|
|
return dict([self.sanitize(item) for item in obj.iteritems()])
|
|
elif isinstance(obj, types.ListType):
|
|
return [self.sanitize(item) for item in obj]
|
|
elif isinstance(obj, types.TupleType):
|
|
k, v = obj
|
|
if self._contains_token(k) and isinstance(v, types.StringTypes):
|
|
return k, self.message
|
|
return k, self.sanitize(v)
|
|
else:
|
|
return obj
|