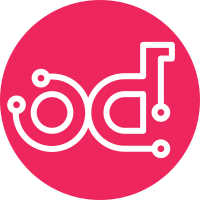
Most of assignment expressions involving indexation operator except for the most trivial one fail. For example the following failed: $myDict[$keyName]: value $myDict['A']['B']: value (even if $myDict['A'] is a dictionary) $myList[2 + 2]: value $myList[$index]: value This commit adds support for sub-expressions within LHS-expressions Change-Id: I4b72e85b5f9bda04c6156d5c8b07eb8f5358643d Closes-Bug: #1358545
44 lines
1.5 KiB
Python
44 lines
1.5 KiB
Python
# Copyright (c) 2014 Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from murano.tests.unit.dsl.foundation import object_model as om
|
|
from murano.tests.unit.dsl.foundation import test_case
|
|
|
|
|
|
class TestAssignments(test_case.DslTestCase):
|
|
def setUp(self):
|
|
super(TestAssignments, self).setUp()
|
|
self._runner = self.new_runner(
|
|
om.Object(
|
|
'SampleClass1',
|
|
stringProperty='string',
|
|
classProperty=om.Object(
|
|
'SampleClass2',
|
|
class2Property='another string')))
|
|
|
|
def test_assignment(self):
|
|
self.assertEqual(
|
|
{
|
|
'Arr': [5, 2, [10, 123]],
|
|
'Dict': {
|
|
'Key1': 'V1',
|
|
'Key2': {'KEY2': 'V3', 'a_b': 'V2'}
|
|
}
|
|
}, self._runner.testAssignment())
|
|
|
|
def test_assign_by_copy(self):
|
|
self.assertEqual(
|
|
[1, 2, 3],
|
|
self._runner.testAssignByCopy([1, 2, 3]))
|