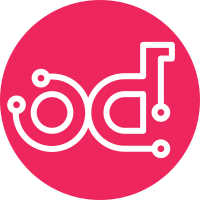
New framework for MuranoPL contracts was written. Now instead of several independent implementations of the same yaql methods (string(), class() etc.) all implementations of the same method are combined into single class so no we have class per contract method. This also simplifies development of new contracts. Each such class can provide methods for data transformation (default contract usqage), validation that is used to decide if the method can be considered an extension method for the value, and json schema generation method that were moved from the schema generator script. Previously for when the class used to override property from the parent class assigned value were stored separately for both of them transformed by each of the contracts. Thus each class saw the value by its contract. In absolute majority of the cases the observed value was the same. However if the contracts were compatible on the provided value (say int() and string() contracts on the value "123") they were different. This is considered to be a bad pattern. Now the value is stored only once per object and transformed by the contract defined in the actual object type. All base contracts are used to validate the transformed object thus the this pattern will not work anymore. The value that is stored in the object's properties is obtained by executing special "finalize" contract implementation which usually returns the input value unmodified. Because validation happens on the transformed value before it gets finalized it is possible for transformation to return a value that will pass the validation though the final value won't. This is used to relax the template() contract limitation that prevented child class to exclude additional properties from the template. string() contracts is no longer converts to string anythin possible but only the scalar values. Previous behavior caused string() property accept lists and convert them to their Python string representation which is clearly not what developers expected. Due to the refactoring contracts work a little bit faster because there is no more need to generate yaql function definition of each contract method on each call. Change-Id: If0e21c2a8c545c4a0419e0ec58e5d129318bb4c2
80 lines
2.9 KiB
Python
80 lines
2.9 KiB
Python
# Copyright (c) 2014 Mirantis, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import sys
|
|
import weakref
|
|
|
|
import six
|
|
|
|
from murano.dsl import dsl_types
|
|
from murano.dsl import exceptions
|
|
from murano.dsl import helpers
|
|
from murano.dsl import meta
|
|
from murano.dsl import typespec
|
|
|
|
|
|
class MuranoProperty(dsl_types.MuranoProperty, typespec.Spec,
|
|
meta.MetaProvider):
|
|
def __init__(self, declaring_type, property_name, declaration):
|
|
super(MuranoProperty, self).__init__(declaration, declaring_type)
|
|
self._property_name = property_name
|
|
self._declaring_type = weakref.ref(declaring_type)
|
|
self._usage = declaration.get('Usage') or dsl_types.PropertyUsages.In
|
|
if self._usage not in dsl_types.PropertyUsages.All:
|
|
raise exceptions.DslSyntaxError(
|
|
'Unknown usage {0}. Must be one of ({1})'.format(
|
|
self._usage, ', '.join(dsl_types.PropertyUsages.All)))
|
|
self._meta = meta.MetaData(
|
|
declaration.get('Meta'),
|
|
dsl_types.MetaTargets.Property, declaring_type)
|
|
self._meta_values = None
|
|
|
|
def transform(self, *args, **kwargs):
|
|
try:
|
|
return super(MuranoProperty, self).transform(*args, **kwargs)
|
|
except exceptions.ContractViolationException as e:
|
|
msg = u'[{0}.{1}{2}] {3}'.format(
|
|
self.declaring_type.name, self.name, e.path, six.text_type(e))
|
|
six.reraise(exceptions.ContractViolationException,
|
|
exceptions.ContractViolationException(msg),
|
|
sys.exc_info()[2])
|
|
|
|
@property
|
|
def name(self):
|
|
return self._property_name
|
|
|
|
@property
|
|
def usage(self):
|
|
return self._usage
|
|
|
|
def get_meta(self, context):
|
|
def meta_producer(cls):
|
|
prop = cls.properties.get(self.name)
|
|
if prop is None:
|
|
return None
|
|
return prop._meta
|
|
|
|
if self._meta_values is None:
|
|
executor = helpers.get_executor()
|
|
context = executor.create_type_context(
|
|
self.declaring_type, caller_context=context)
|
|
|
|
self._meta_values = meta.merge_providers(
|
|
self.declaring_type, meta_producer, context)
|
|
return self._meta_values
|
|
|
|
def __repr__(self):
|
|
return 'MuranoProperty({type}::{name})'.format(
|
|
type=self.declaring_type.name, name=self.name)
|