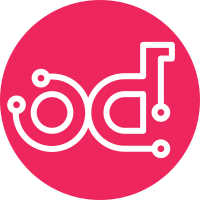
The example in the docs doesn't quite work as the file stored in the "content" variable is already closed, and ends up spitting out encoded data to the destination files. It was properly being sent to S3, but not getting saved locally. This saves the file locally first, then using local storage, opens the file once again for the S3BotoStorage save call.
90 lines
3.6 KiB
Plaintext
90 lines
3.6 KiB
Plaintext
.. _remote_storages:
|
|
|
|
Remote storages
|
|
---------------
|
|
|
|
In some cases it's useful to use a CDN_ for serving static files such as
|
|
those generated by Django Compressor. Due to the way Django Compressor
|
|
processes files, it requires the files to be processed (in the
|
|
``{% compress %}`` block) to be available in a local file system cache.
|
|
|
|
Django Compressor provides hooks to automatically have compressed files
|
|
pushed to a remote storage backend. Simply set the storage backend
|
|
that saves the result to a remote service (see
|
|
:attr:`~django.conf.settings.COMPRESS_STORAGE`).
|
|
|
|
django-storages
|
|
^^^^^^^^^^^^^^^
|
|
|
|
So assuming your CDN is `Amazon S3`_, you can use the boto_ storage backend
|
|
from the 3rd party app `django-storages-redux`_. Some required settings are::
|
|
|
|
AWS_ACCESS_KEY_ID = 'XXXXXXXXXXXXXXXXXXXXX'
|
|
AWS_SECRET_ACCESS_KEY = 'XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
|
|
AWS_STORAGE_BUCKET_NAME = 'compressor-test'
|
|
|
|
Next, you need to specify the new CDN base URL and update the URLs to the
|
|
files in your templates which you want to compress::
|
|
|
|
COMPRESS_URL = "http://compressor-test.s3.amazonaws.com/"
|
|
|
|
.. note::
|
|
|
|
For staticfiles just set ``STATIC_URL = COMPRESS_URL``
|
|
|
|
The storage backend to save the compressed files needs to be changed, too::
|
|
|
|
COMPRESS_STORAGE = 'storages.backends.s3boto.S3BotoStorage'
|
|
|
|
Using staticfiles
|
|
^^^^^^^^^^^^^^^^^
|
|
|
|
If you are using Django's staticfiles_ contrib app, you'll need to use a
|
|
temporary filesystem cache for Django Compressor to know which files to
|
|
compress. Since staticfiles provides a management command to collect static
|
|
files from various locations which uses a storage backend, this is where both
|
|
apps can be integrated.
|
|
|
|
#. Make sure the :attr:`~django.conf.settings.COMPRESS_ROOT` and STATIC_ROOT_
|
|
settings are equal since both apps need to look at the same directories
|
|
when to do their job.
|
|
|
|
#. You need to create a subclass of the remote storage backend you want
|
|
to use; below is an example of the boto S3 storage backend from
|
|
django-storages-redux_::
|
|
|
|
from django.core.files.storage import get_storage_class
|
|
from storages.backends.s3boto import S3BotoStorage
|
|
|
|
class CachedS3BotoStorage(S3BotoStorage):
|
|
"""
|
|
S3 storage backend that saves the files locally, too.
|
|
"""
|
|
def __init__(self, *args, **kwargs):
|
|
super(CachedS3BotoStorage, self).__init__(*args, **kwargs)
|
|
self.local_storage = get_storage_class(
|
|
"compressor.storage.CompressorFileStorage")()
|
|
|
|
def save(self, name, content):
|
|
self.local_storage._save(name, content)
|
|
super(CachedS3BotoStorage, self).save(name, self.local_storage._open(name))
|
|
return name
|
|
|
|
#. Set your :attr:`~django.conf.settings.COMPRESS_STORAGE` and STATICFILES_STORAGE_
|
|
settings to the dotted path of your custom cached storage backend, e.g.
|
|
``'mysite.storage.CachedS3BotoStorage'``.
|
|
|
|
#. To have Django correctly render the URLs to your static files, set the
|
|
STATIC_URL_ setting to the same value as
|
|
:attr:`~django.conf.settings.COMPRESS_URL` (e.g.
|
|
``"http://compressor-test.s3.amazonaws.com/"``).
|
|
|
|
.. _CDN: http://en.wikipedia.org/wiki/Content_delivery_network
|
|
.. _Amazon S3: https://s3.amazonaws.com/
|
|
.. _boto: http://boto.cloudhackers.com/
|
|
.. _django-storages-redux: http://github.com/jschneier/django-storages
|
|
.. _staticfiles: http://docs.djangoproject.com/en/dev/howto/static-files/
|
|
.. _STATIC_ROOT: http://docs.djangoproject.com/en/dev/ref/settings/#static-root
|
|
.. _STATIC_URL: http://docs.djangoproject.com/en/dev/ref/settings/#static-url
|
|
.. _STATICFILES_STORAGE: http://docs.djangoproject.com/en/dev/ref/contrib/staticfiles/#staticfiles-storage
|